github.com/42z-io/confik@v0.0.2-0.20231103050132-21d8f377356c/README.md (about) 1 # confik 2 [](https://github.com/42z-io/confik/actions/workflows/build_test.yml) [](https://coveralls.io/github/42z-io/confik?branch=main) [](https://github.com/42z-io/confik/releases/) 3 [](https://github.com/42z-io/confik/blob/main/LICENSE) [](https://pkg.go.dev/github.com/42z-io/confik) 4 5 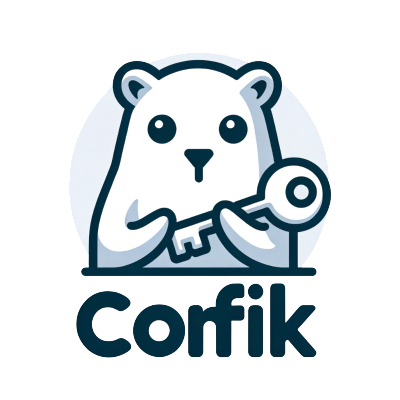 6 7 8 `Confik` parses environment files and variables and loads them into a struct. 9 10 ## Usage 11 12 ``` 13 go get github.com/42z-io/confik 14 ``` 15 16 ```go 17 import ( 18 "os" 19 "fmt" 20 "github.com/42z-io/confik" 21 ) 22 23 type ExampleConfig struct { 24 Name string 25 Age uint8 `env:"AGE,optional"` 26 Height float32 27 } 28 29 func init() { 30 os.Setenv("NAME", "Bob") 31 os.Setenv("AGE", "20") 32 os.Setenv("HEIGHT", "5.3") 33 34 cfg, _ := confik.LoadFromEnv(Config[ExampleConfig]{ 35 UseEnvFile: false, 36 }) 37 38 fmt.Println(cfg.Name) 39 fmt.Println(cfg.Age) 40 fmt.Println(cfg.Height) 41 // Output: Bob 42 // 20 43 // 5.3 44 } 45 ```