github.com/99designs/gqlgen@v0.17.45/README.md (about) 1 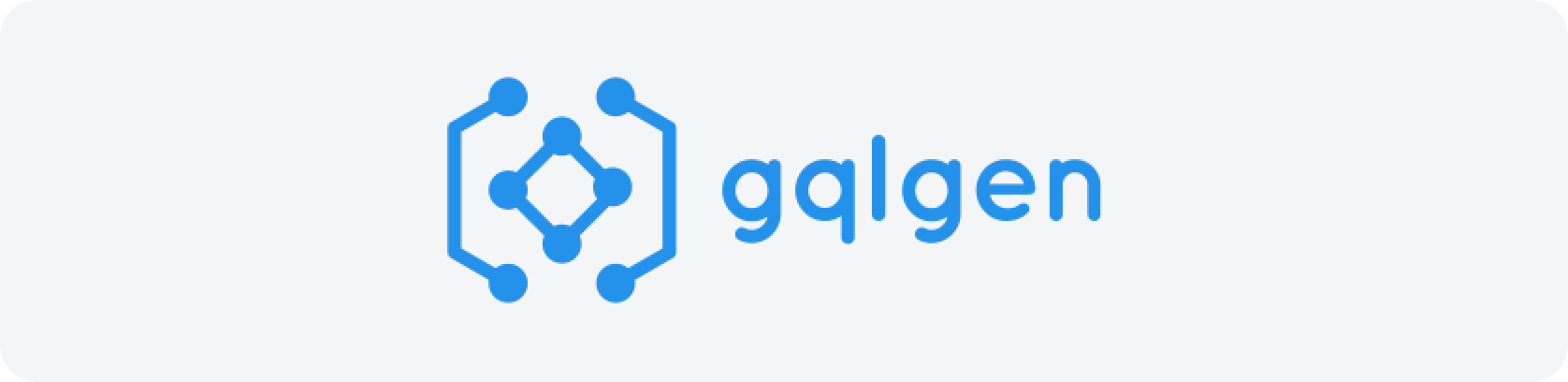 2 3 4 # gqlgen [](https://github.com/99designs/gqlgen/actions) [](https://coveralls.io/github/99designs/gqlgen?branch=master) [](https://goreportcard.com/report/github.com/99designs/gqlgen) [](https://pkg.go.dev/github.com/99designs/gqlgen) [](http://gqlgen.com/) 5 6 ## What is gqlgen? 7 8 [gqlgen](https://github.com/99designs/gqlgen) is a Go library for building GraphQL servers without any fuss.<br/> 9 10 - **gqlgen is based on a Schema first approach** — You get to Define your API using the GraphQL [Schema Definition Language](http://graphql.org/learn/schema/). 11 - **gqlgen prioritizes Type safety** — You should never see `map[string]interface{}` here. 12 - **gqlgen enables Codegen** — We generate the boring bits, so you can focus on building your app quickly. 13 14 Still not convinced enough to use **gqlgen**? Compare **gqlgen** with other Go graphql [implementations](https://gqlgen.com/feature-comparison/) 15 16 ## Quick start 17 1. [Initialise a new go module](https://golang.org/doc/tutorial/create-module) 18 19 mkdir example 20 cd example 21 go mod init example 22 23 2. Add `github.com/99designs/gqlgen` to your [project's tools.go](https://github.com/golang/go/wiki/Modules#how-can-i-track-tool-dependencies-for-a-module) 24 25 printf '// +build tools\npackage tools\nimport (_ "github.com/99designs/gqlgen"\n _ "github.com/99designs/gqlgen/graphql/introspection")' | gofmt > tools.go 26 27 go mod tidy 28 29 3. Initialise gqlgen config and generate models 30 31 go run github.com/99designs/gqlgen init 32 33 go mod tidy 34 35 4. Start the graphql server 36 37 go run server.go 38 39 More help to get started: 40 - [Getting started tutorial](https://gqlgen.com/getting-started/) - a comprehensive guide to help you get started 41 - [Real-world examples](https://github.com/99designs/gqlgen/tree/master/_examples) show how to create GraphQL applications 42 - [Reference docs](https://pkg.go.dev/github.com/99designs/gqlgen) for the APIs 43 44 ## Reporting Issues 45 46 If you think you've found a bug, or something isn't behaving the way you think it should, please raise an [issue](https://github.com/99designs/gqlgen/issues) on GitHub. 47 48 ## Contributing 49 50 We welcome contributions, Read our [Contribution Guidelines](https://github.com/99designs/gqlgen/blob/master/CONTRIBUTING.md) to learn more about contributing to **gqlgen** 51 ## Frequently asked questions 52 53 ### How do I prevent fetching child objects that might not be used? 54 55 When you have nested or recursive schema like this: 56 57 ```graphql 58 type User { 59 id: ID! 60 name: String! 61 friends: [User!]! 62 } 63 ``` 64 65 You need to tell gqlgen that it should only fetch friends if the user requested it. There are two ways to do this; 66 67 - #### Using Custom Models 68 69 Write a custom model that omits the friends field: 70 71 ```go 72 type User struct { 73 ID int 74 Name string 75 } 76 ``` 77 78 And reference the model in `gqlgen.yml`: 79 80 ```yaml 81 # gqlgen.yml 82 models: 83 User: 84 model: github.com/you/pkg/model.User # go import path to the User struct above 85 ``` 86 87 - #### Using Explicit Resolvers 88 89 If you want to keep using the generated model, mark the field as requiring a resolver explicitly in `gqlgen.yml` like this: 90 91 ```yaml 92 # gqlgen.yml 93 models: 94 User: 95 fields: 96 friends: 97 resolver: true # force a resolver to be generated 98 ``` 99 100 After doing either of the above and running generate we will need to provide a resolver for friends: 101 102 ```go 103 func (r *userResolver) Friends(ctx context.Context, obj *User) ([]*User, error) { 104 // select * from user where friendid = obj.ID 105 return friends, nil 106 } 107 ``` 108 109 You can also use inline config with directives to achieve the same result 110 111 ```graphql 112 directive @goModel(model: String, models: [String!]) on OBJECT 113 | INPUT_OBJECT 114 | SCALAR 115 | ENUM 116 | INTERFACE 117 | UNION 118 119 directive @goField(forceResolver: Boolean, name: String, omittable: Boolean) on INPUT_FIELD_DEFINITION 120 | FIELD_DEFINITION 121 122 type User @goModel(model: "github.com/you/pkg/model.User") { 123 id: ID! @goField(name: "todoId") 124 friends: [User!]! @goField(forceResolver: true) 125 } 126 ``` 127 128 ### Can I change the type of the ID from type String to Type Int? 129 130 Yes! You can by remapping it in config as seen below: 131 132 ```yaml 133 models: 134 ID: # The GraphQL type ID is backed by 135 model: 136 - github.com/99designs/gqlgen/graphql.IntID # a go integer 137 - github.com/99designs/gqlgen/graphql.ID # or a go string 138 ``` 139 140 This means gqlgen will be able to automatically bind to strings or ints for models you have written yourself, but the 141 first model in this list is used as the default type and it will always be used when: 142 143 - Generating models based on schema 144 - As arguments in resolvers 145 146 There isn't any way around this, gqlgen has no way to know what you want in a given context. 147 148 ### Why do my interfaces have getters? Can I disable these? 149 These were added in v0.17.14 to allow accessing common interface fields without casting to a concrete type. 150 However, certain fields, like Relay-style Connections, cannot be implemented with simple getters. 151 152 If you'd prefer to not have getters generated in your interfaces, you can add the following in your `gqlgen.yml`: 153 ```yaml 154 # gqlgen.yml 155 omit_getters: true 156 ``` 157 158 ## Other Resources 159 160 - [Christopher Biscardi @ Gophercon UK 2018](https://youtu.be/FdURVezcdcw) 161 - [Introducing gqlgen: a GraphQL Server Generator for Go](https://99designs.com.au/blog/engineering/gqlgen-a-graphql-server-generator-for-go/) 162 - [Dive into GraphQL by Iván Corrales Solera](https://medium.com/@ivan.corrales.solera/dive-into-graphql-9bfedf22e1a) 163 - [Sample Project built on gqlgen with Postgres by Oleg Shalygin](https://github.com/oshalygin/gqlgen-pg-todo-example) 164 - [Hackernews GraphQL Server with gqlgen by Shayegan Hooshyari](https://www.howtographql.com/graphql-go/0-introduction/)