github.com/GetStream/moq@v0.0.0-20181113105103-b721cd3f6524/README.md (about) 1 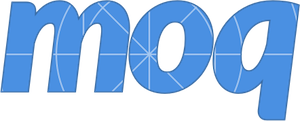 [](https://travis-ci.org/matryer/moq) [](https://goreportcard.com/report/github.com/matryer/moq) 2 3 Interface mocking tool for go generate. 4 5 By [Mat Ryer](https://twitter.com/matryer) and [David Hernandez](https://github.com/dahernan), with ideas lovingly stolen from [Ernesto Jimenez](https://github.com/ernesto-jimenez). 6 7 ### What is Moq? 8 9 Moq is a tool that generates a struct from any interface. The struct can be used in test code as a mock of the interface. 10 11 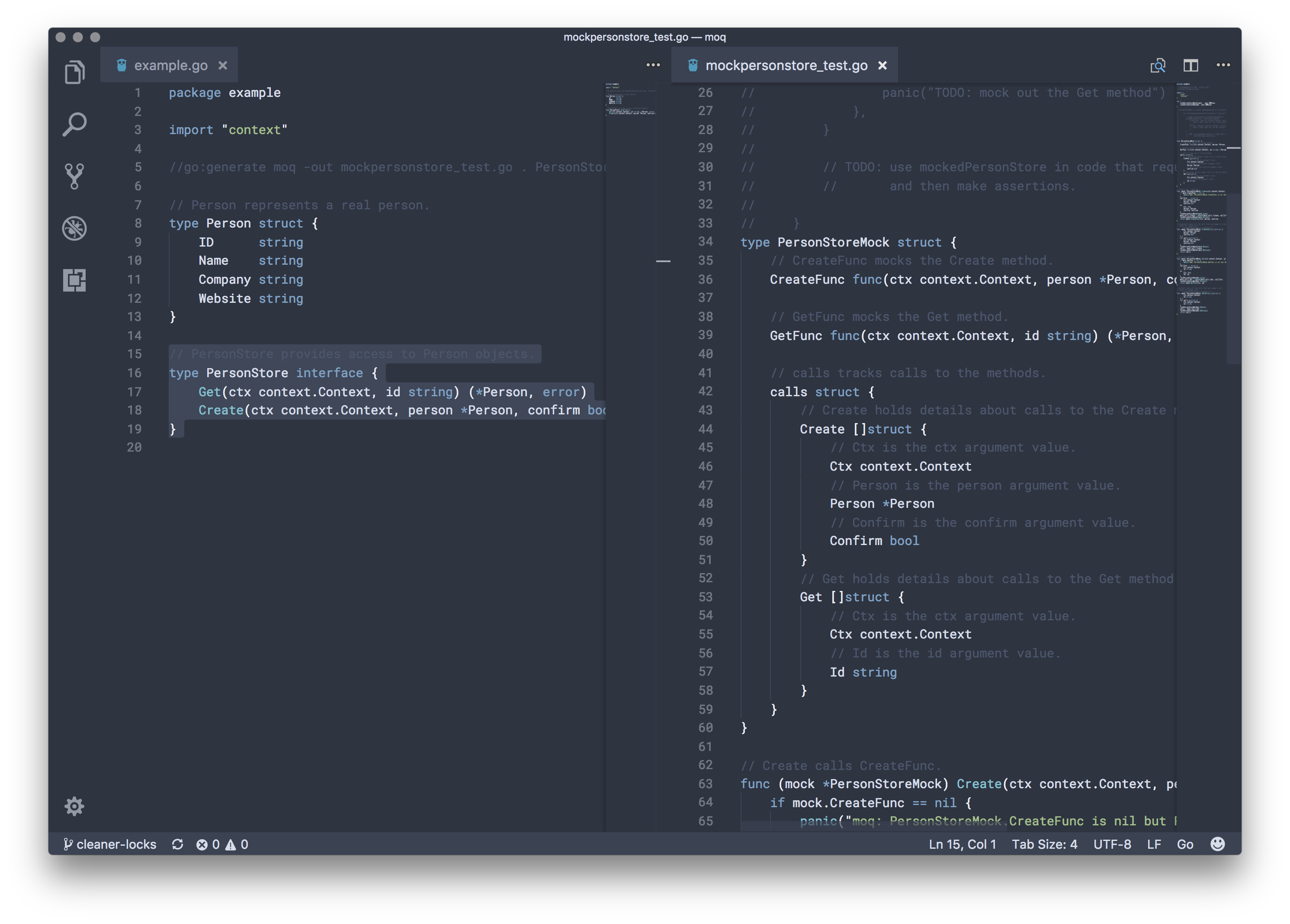 12 13 above: Moq generates the code on the right. 14 15 You can read more in the [Meet Moq blog post](http://bit.ly/meetmoq). 16 17 ### Installing 18 19 To start using Moq, just run go get: 20 ``` 21 $ go get github.com/matryer/moq 22 ``` 23 24 ### Usage 25 26 ``` 27 moq [flags] destination interface [interface2 [interface3 [...]]] 28 -out string 29 output file (default stdout) 30 -pkg string 31 package name (default will infer) 32 ``` 33 34 In a command line: 35 36 ``` 37 $ moq -out mocks_test.go . MyInterface 38 ``` 39 40 In code (for go generate): 41 42 ```go 43 package my 44 45 //go:generate moq -out myinterface_moq_test.go . MyInterface 46 47 type MyInterface interface { 48 Method1() error 49 Method2(i int) 50 } 51 ``` 52 53 Then run `go generate` for your package. 54 55 ### How to use it 56 57 Mocking interfaces is a nice way to write unit tests where you can easily control the behaviour of the mocked object. 58 59 Moq creates a struct that has a function field for each method, which you can declare in your test code. 60 61 This this example, Moq generated the `EmailSenderMock` type: 62 63 ```go 64 func TestCompleteSignup(t *testing.T) { 65 66 var sentTo string 67 68 mockedEmailSender = &EmailSenderMock{ 69 SendFunc: func(to, subject, body string) error { 70 sentTo = to 71 return nil 72 }, 73 } 74 75 CompleteSignUp("me@email.com", mockedEmailSender) 76 77 callsToSend := len(mockedEmailSender.SendCalls()) 78 if callsToSend != 1 { 79 t.Errorf("Send was called %d times", callsToSend) 80 } 81 if sentTo != "me@email.com" { 82 t.Errorf("unexpected recipient: %s", sentTo) 83 } 84 85 } 86 87 func CompleteSignUp(to string, sender EmailSender) { 88 // TODO: this 89 } 90 ``` 91 92 The mocked structure implements the interface, where each method calls the associated function field. 93 94 ## Tips 95 96 * Keep mocked logic inside the test that is using it 97 * Only mock the fields you need 98 * It will panic if a nil function gets called 99 * Name arguments in the interface for a better experience 100 * Use closured variables inside your test function to capture details about the calls to the methods 101 * Use `.MethodCalls()` to track the calls 102 * Use `go:generate` to invoke the `moq` command 103 104 ## License 105 106 The Moq project (and all code) is licensed under the [MIT License](LICENSE). 107 108 The Moq logo was created by [Chris Ryer](http://chrisryer.co.uk) and is licensed under the [Creative Commons Attribution 3.0 License](https://creativecommons.org/licenses/by/3.0/).