github.com/aergoio/aergo@v1.3.1/cmd/brick/README.md (about) 1 2 # Brick - Toy for Contract Developers 3 4 This is an interactive shell program to communicate with an aergo vm for testing. 5 This also provides a batch function to test and help to develop smart contrat. 6 7 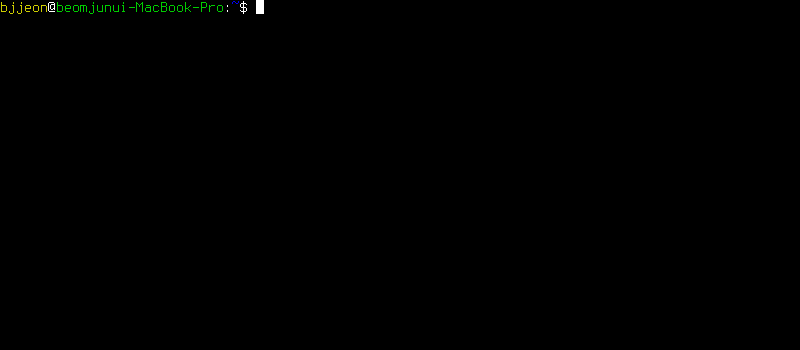 8 9 ## Features 10 11 ### Aergo VM for Testing 12 13 * provides an way to run smart contracts in same environment with aergo 14 * supports state db, sql 15 * alias for account and contract address 16 * able to debug smart contracts 17 18 ### Interactive Shell 19 20 powered by [go-prompt](https://github.com/c-bata/go-prompt) 21 22 * auto complete 23 * keywords suggestion 24 * history 25 26 ### Batch for Integration Tests 27 28 * load and execute bulk of commands from file 29 * contract integration test 30 * support incremental test 31 32 ## Install 33 34 1. git clone and build aergo in debug mode (release is ok, but you cannot debug contracts), unix & mac: `make debug`, windows + mingw64: `mingw32-make debug` 35 2. create a directory, where you want 36 3. copy binary `brick` to the directory, or add aergo's `bin` folder to system PATH 37 4. move to the directory 38 5. run `brick` 39 6. (optional) copy log config file `cmd/brick/arglog.toml` to the directory, and adjust levels to get for more detailed info 40 41 ## Usage 42 43 ### inject 44 45 creates an account and deposite aergo. `inject inject <account_name> <amount>` 46 47 ``` lua 48 0> inject tester 100 49 INF inject an account successfully cmd=inject module=brick 50 ``` 51 52 ### getstate 53 54 get current balance of an account. `getstate getstate <account_name> [expected_balance]` This will returns in a form of `<internal_address>=<remaining_balance>` 55 56 ``` lua 57 1> getstate tester 58 INF Amh8FdZavYGu9ABhZQF6Y7LnSMi2a714bGvpm6mQuMnRpmoYn11C=100 cmd=getstate module=brick 59 ``` 60 61 ### send 62 63 transfer aergo between two addresses. `send <sender_name> <receiver_name> <amount>` 64 65 ``` lua 66 1> send tester receiver 10 67 INF send aergo successfully cmd=send module=brick 68 ``` 69 70 ### deploy 71 72 deploy a smart contract. `deploy <sender_name> <fee_amount> <contract_name> <definition_file_path> [contructor_json_arg]` 73 74 ``` lua 75 2> deploy tester 0 helloContract ./example/hello.lua 76 INF deploy a smart contract successfully cmd=deploy module=brick 77 ``` 78 79 deploy a raw text file on http also available. 80 81 ``` lua 82 2> deploy tester 0 helloContract https://raw.githubusercontent.com/aergoio/aergo-contract-ex/master/contracts/helloworld/test/test-helloworld.brick 83 INF deploy a smart contract successfully cmd=deploy module=brick 84 ``` 85 86 ### call 87 88 call to execute a smart contract. `call <sender_name> <amount> <contract_name> <func_name> <call_json_str> [expected_error]` 89 90 ``` lua 91 3> call tester 0 helloContract set_name `["aergo"]` 92 INF call a smart contract successfully cmd=call module=brick 93 ``` 94 95 ### query 96 97 query to a smart contract. `query <contract_name> <func_name> <query_json_str> [expected_query_result] [expected_error]` 98 99 ``` lua 100 4> query helloContract hello `[]` 101 INF "hello aergo" cmd=query module=brick 102 ``` 103 104 `expected_query_result`, surrounded by [], is optional. But you can test whether the results are correct using this. 105 106 ``` lua 107 4> query helloContract hello `[]` `"hello aergo"` 108 INF query compare successfully cmd=query module=brick 109 4> query helloContract hello `[]` `"hello incorrect example"` 110 ERR execution fail error="expected: \"hello incorrect example\", but got: \"hello aergo\"" cmd=query module=brick 111 ``` 112 113 ### batch 114 115 keeps commands in a text file (local or http) and use at later. `batch <batch_file_path>` 116 117 ``` lua 118 4> batch ./example/hello.brick 119 Batch is successfully finished 120 8> 121 ``` 122 123 ### undo 124 125 cancels the last tx (inject, send, deploy, call). `undo` 126 127 ``` lua 128 8> undo 129 INF Undo, Succesfully cmd=undo module=brick 130 ``` 131 132 ### forward 133 134 skip blocks. `forward [height_to_skip]` 135 136 ``` lua 137 7> forward 100 138 INF fast forward blocks successfully cmd=forward module=brick 139 ``` 140 141 ### reset 142 143 clear all txs and reset the chain. `reset` 144 145 ``` lua 146 107> reset 147 INF reset a dummy chain successfully cmd=reset module=brick 148 0> 149 ``` 150 151 Number before cursor is a block height. Each block contains one tx. So after reset, number becames 0 152 153 ### batch in command line 154 155 In command line, users can run a brick batch file. A running result contains line numbers and original texts for debugging purpose. 156 157 ``` bash 158 $ ./brick ./example/hello.brick 159 Batch is successfully finished 160 INF batch exec is finished cmd=batch module=brick 161 ``` 162 User can check the detail results by setting `-v` options. 163 164 ``` bash 165 $ ./brick ./example/hello.brick -v 166 1 # create an account and deposit coin 167 2 inject bj 100 168 INF inject an account successfully cmd=inject module=brick 169 3 170 4 # check balance 171 5 getstate bj 172 INF AmgiDbXB3x5Zv5cBBcmyzSJiqMbLXG1yZN2xE4gUuXMBaXPfGoun = 100 cmd=getstate module=brick 173 6 174 7 # delpoy helloworld smart contract 175 8 deploy bj 0 helloctr `./example/hello.lua` 176 INF deploy a smart contract successfully cmd=deploy module=brick 177 9 178 10 # query to contract, this will print "hello world" 179 11 query helloctr hello `[]` `"hello world"` 180 INF query compare successfully cmd=query module=brick 181 12 182 13 # call and execute contract 183 14 call bj 0 helloctr set_name `["aergo"]` 184 INF call a smart contract successfully cmd=call module=brick 185 15 186 16 # query again, this now will print "hello aergo" 187 17 query helloctr hello `[]` `"hello aergo"` 188 INF query compare successfully cmd=query module=brick 189 Batch is successfully finished 190 INF batch exec is finished cmd=batch module=brick 191 ``` 192 Or user can set the option `-w` to display the batch execution results continuously according to the file changes. This is an useful feature for the development phase. 193 194 ## Debugging 195 196 If you build in debug mode (`make debug`), you can use `os, io, debug` modules which is not allowed in release mode. There is no limit to which debugger to use, but brick provides built-in debugger using customized [clidebugger](https://github.com/ToddWegner/clidebugger). For debugging purpose, brick has extended commands. 197 198 ### setb (brick / debugmode) 199 200 Set a breakpoint to the contract. When vm reach the line of breakpoint during a call or query of a contract, it enters debugmode only. contract_name is optional in debugmode. `setb <line> [contract_name]` 201 202 ### delb (brick / debugmode) 203 204 Delete an existing breakpoint. contract_name is optional in debugmode. `delb <line> [contract_name]` 205 206 ### listb (brick / debugmode) 207 208 Prints all breakpoints. `listb` 209 210 ### resetb (brick / debugmode) 211 212 Clear all breakpoints. `resetb` 213 214 ### setw (brick / debugmode) 215 216 Set an watchpoint expression. If one of watchpoint expressions is satisfied without regard to which contract is being executed, debug mode is activated. `setw <watch_expression>` 217 218 ### delw (brick / debugmode) 219 220 Delete an existing watchpoint. `delw <watch_index>` 221 222 ### listw (brick / debugmode) 223 224 Prints all watchpoints. `listw` 225 226 ### resetw (brick / debugmode) 227 228 Clear all watchpoints. `resetw` 229 230 ### in debugmode 231 232 When vm enters debugmode, prompt changes to `[DEBUG]>`. In debugmode, command set is changed for debugging purpose, like `run`, `exit`, `show`, `vars`. For more detail, type `help`. 233 234 ## Debug using Zerobrane Studio 235 236 Here we describe GUI based debugging using the zerobrane studio. 237 238 1. download [zerobrane studio](https://studio.zerobrane.com/support) (lua ide) 239 2. set envoronment var `LUA_PATH, LUACPATH` following an [instruction](https://studio.zerobrane.com/doc-remote-debugging) 240 3. Paste `require('mobdebug').start()` at the beginning of a smart contract, you want to investigate 241 4. Open the contract file you want to investigate in the editor 242 5. In the editor, run debug server `project -> start debugger server` 243 6. Using brick, deploy and run the contract 244 7. When you get to that line, it will automatically be switched to the editor 245 246 (CAUTION!) After testing, When distributing to an actual blockchain, you must remove the code used for debugging. It will cause error.