github.com/amimof/huego@v1.2.1/README.md (about) 1 [](https://github.com/amimof/huego/actions/workflows/go.yaml) [](https://goreportcard.com/report/github.com/amimof/huego) [](https://codecov.io/gh/amimof/huego) [](https://github.com/avelino/awesome-go) 2 3 # Huego 4 5 An extensive Philips Hue client library for [`Go`](https://golang.org/) with an emphasis on simplicity. It is designed to be clean, unbloated and extensible. With `Huego` you can interact with any Philips Hue bridge and its resources including `Lights`, `Groups`, `Scenes`, `Sensors`, `Rules`, `Schedules`, `Resourcelinks`, `Capabilities` and `Configuration` . 6 7 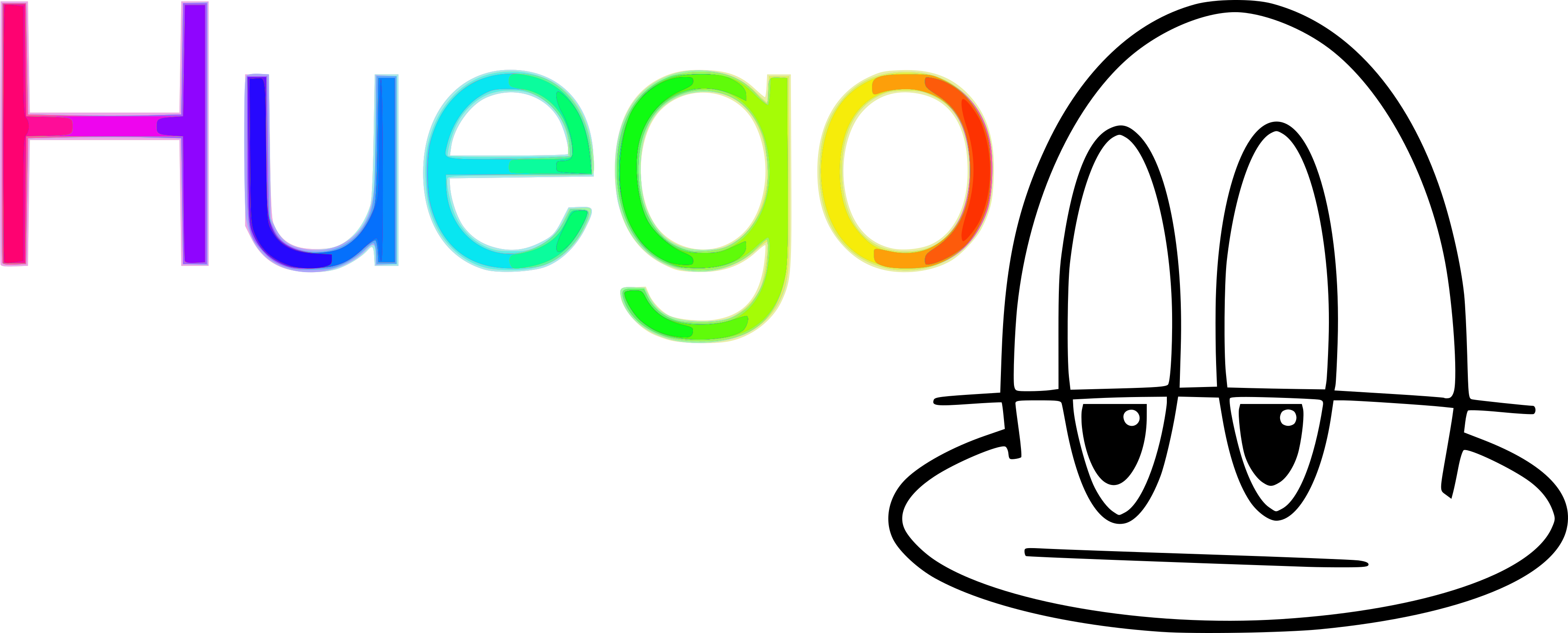 8 9 10 ## Installation 11 Get the package and import it in your code. 12 ``` 13 go get github.com/amimof/huego 14 ``` 15 You may use [`New()`](https://godoc.org/github.com/amimof/huego#New) if you have already created an user and know the IP address to your bridge. 16 ```Go 17 package main 18 19 import ( 20 "github.com/amimof/huego" 21 "fmt" 22 ) 23 24 func main() { 25 bridge := huego.New("192.168.1.59", "username") 26 l, err := bridge.GetLights() 27 if err != nil { 28 panic(err) 29 } 30 fmt.Printf("Found %d lights", len(l)) 31 } 32 ``` 33 Or discover a bridge on your network with [`Discover()`](https://godoc.org/github.com/amimof/huego#Discover) and create a new user with [`CreateUser()`](https://godoc.org/github.com/amimof/huego#Bridge.CreateUser). To successfully create a user, the link button on your bridge must have been pressed before calling `CreateUser()` in order to authorise the request. 34 ```Go 35 func main() { 36 bridge, _ := huego.Discover() 37 user, _ := bridge.CreateUser("my awesome hue app") // Link button needs to be pressed 38 bridge = bridge.Login(user) 39 light, _ := bridge.GetLight(3) 40 light.Off() 41 } 42 ``` 43 44 ## Documentation 45 46 See [godoc.org/github.com/amimof/huego](https://godoc.org/github.com/amimof/huego) for the full package documentation. 47 48 ## Contributing 49 50 All help in any form is highly appreciated and your are welcome participate in developing `Huego` together. To contribute submit a `Pull Request`. If you want to provide feedback, open up a Github `Issue` or contact me personally.