github.com/darmach/terratest@v0.34.8-0.20210517103231-80931f95e3ff/examples/terraform-asg-scp-example/README.md (about) 1 # Terraform ASG SCP Example 2 3 This folder contains a simple Terraform module that deploys resources in [AWS](https://aws.amazon.com/) to demonstrate 4 how you can use Terratest to write automated tests for your AWS Terraform code. This module deploys an ASG with one instance. 5 The EC2 Instance allows SSH requests on the port specified by the `ssh_port` variable. 6 7 Check out [test/terraform_scp_example_test.go](/test/terraform_scp_example_test.go) to see how you can write 8 automated tests for this module. 9 10 Note that the example in this module is still fairly simplified, as the EC2 Instance doesn't do a whole lot! For a more 11 complicated, real-world, end-to-end example of a Terraform module and web server, see 12 [terraform-packer-example](/examples/terraform-packer-example). 13 14 **WARNING**: This module and the automated tests for it deploy real resources into your AWS account which can cost you 15 money. The resources are all part of the [AWS Free Tier](https://aws.amazon.com/free/), so if you haven't used that up, 16 it should be free, but you are completely responsible for all AWS charges. 17 18 ## Overview 19 20 When a test fails, it is often important to be able to quickly get to logs and config files from your deployed apps and services. Currently, getting at this information is a bit of a pain. Often times, it would be necessary to "catch it in the act". Usually this would require running tests and then "pausing"/not tearing down the infrastructure, ssh-ing to individual instances and then viewing the logs/config files that way. This in not very convenient and gets even more tricky when trying to get the same results for tests being executed by your CI server. 21 22 You can use terratest to help with this task by specifying `RemoteFileSpecification` structs that describe which files you want to copy from your instances: 23 24 ```go 25 logstashSpec := aws.RemoteFileSpecification{ 26 SshUser:sshUserName, 27 UseSudo:true, 28 KeyPair:keyPair, 29 LocalDestinationDir:filepath.Join("/tmp", "logs", t.Name(), "logstash"), 30 AsgNames: strings.Split(strings.Replace(terraform.OutputRequired(t, terraformOptions, "logstash_server_asg_names"), "\n", "", -1), ","), 31 RemotePathToFileFilter: map[string][]string { 32 "/var/log/logstash":{"*"}, 33 "/etc/logstash/conf.d" : {"*"}, 34 }, 35 } 36 ``` 37 38 Once you've described what files you want, grabbing them from ASGs is simple with: 39 ```go 40 aws.FetchFilesFromAllAsgsE(t, awsRegion, logstashSpec) 41 ``` 42 43 or directly from EC2 instances with: 44 ```go 45 aws.FetchFilesFromInstance(t, awsRegion, sshUserName, keyPair, appServerInstanceId, true, appServerConfig, filepath.Join("/tmp", "logs", t.Name(), "app_server"), []string{"*.yml", "caFile", "*.key", "*.pem"}) 46 ``` 47 48 Finally, to put all of this together, in your go test you could do something like: 49 50 ```go 51 defer test_structure.RunTestStage(t, "grab_logs", func() { 52 if t.Failed() { 53 takeElkMultiClusterLogSnapshot(t, examplesDir, awsRegion, "ubuntu") 54 } 55 }) 56 ``` 57 58 The code above will run at the very end of your test and grab a snapshot of all of the log descriptors you've defined specifically when your tests fail. We usually like to defer the logging snapshot right before we defer the terraform teardown. This way, if our tests fail, we are able to grab a snapshot of all the relevant logs and config files across our whole deployment! 59 60 You can even take this a step further and pair this with your CI's artifact storage mechanism to have all of your logs and config files attached to your broken CI build when tests failed. For example, with CircleCI, you could do something like: 61 62 ```yml 63 - store_artifacts: 64 path: /tmp/logs 65 ``` 66 67 Now, to get at your logs when your tests fail, you will just be able to click the links right in your CI. 68 69 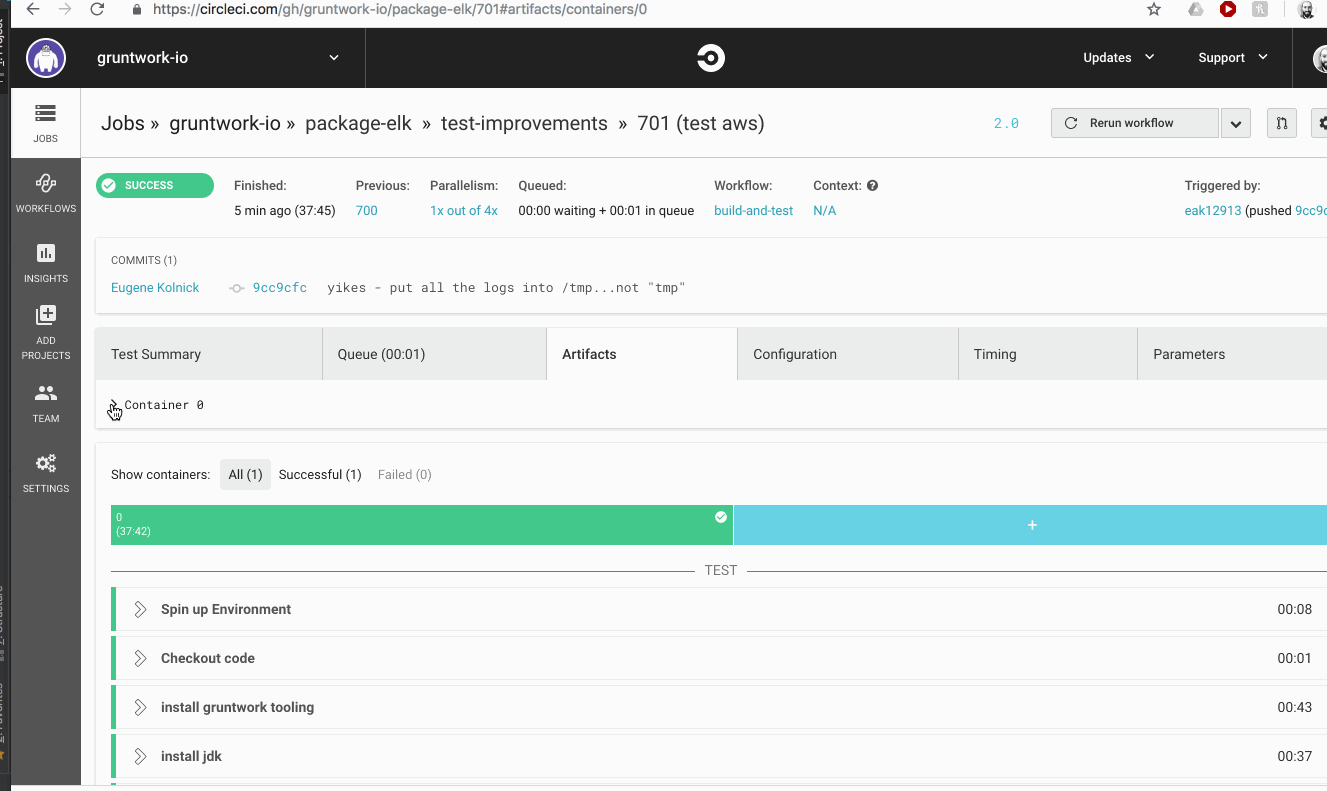 70 71 ## Running this module manually 72 73 1. Sign up for [AWS](https://aws.amazon.com/). 74 1. Configure your AWS credentials using one of the [supported methods for AWS CLI 75 tools](https://docs.aws.amazon.com/cli/latest/userguide/cli-chap-getting-started.html), such as setting the 76 `AWS_ACCESS_KEY_ID` and `AWS_SECRET_ACCESS_KEY` environment variables. If you're using the `~/.aws/config` file for profiles then export `AWS_SDK_LOAD_CONFIG` as "True". 77 1. Install [Terraform](https://www.terraform.io/) and make sure it's on your `PATH`. 78 1. Run `terraform init`. 79 1. Run `terraform apply`. 80 1. When you're done, run `terraform destroy`. 81 82 83 84 85 ## Running automated tests against this module 86 87 1. Sign up for [AWS](https://aws.amazon.com/). 88 1. Configure your AWS credentials using one of the [supported methods for AWS CLI 89 tools](https://docs.aws.amazon.com/cli/latest/userguide/cli-chap-getting-started.html), such as setting the 90 `AWS_ACCESS_KEY_ID` and `AWS_SECRET_ACCESS_KEY` environment variables. If you're using the `~/.aws/config` file for profiles then export `AWS_SDK_LOAD_CONFIG` as "True". 91 1. Install [Terraform](https://www.terraform.io/) and make sure it's on your `PATH`. 92 1. Install [Golang](https://golang.org/) and make sure this code is checked out into your `GOPATH`. 93 1. `cd test` 94 1. `dep ensure` 95 1. `go test -v -run TestTerraformScpExample`