github.com/demonoid81/containerd@v1.3.4/README.md (about) 1  2 3 [](https://godoc.org/github.com/containerd/containerd) 4 [](https://travis-ci.org/containerd/containerd) 5 [](https://ci.appveyor.com/project/mlaventure/containerd-3g73f?branch=master) 6 [](https://app.fossa.io/projects/git%2Bhttps%3A%2F%2Fgithub.com%2Fcontainerd%2Fcontainerd?ref=badge_shield) 7 [](https://goreportcard.com/report/github.com/containerd/containerd) 8 [](https://bestpractices.coreinfrastructure.org/projects/1271) 9 10 containerd is an industry-standard container runtime with an emphasis on simplicity, robustness and portability. It is available as a daemon for Linux and Windows, which can manage the complete container lifecycle of its host system: image transfer and storage, container execution and supervision, low-level storage and network attachments, etc. 11 12 containerd is designed to be embedded into a larger system, rather than being used directly by developers or end-users. 13 14 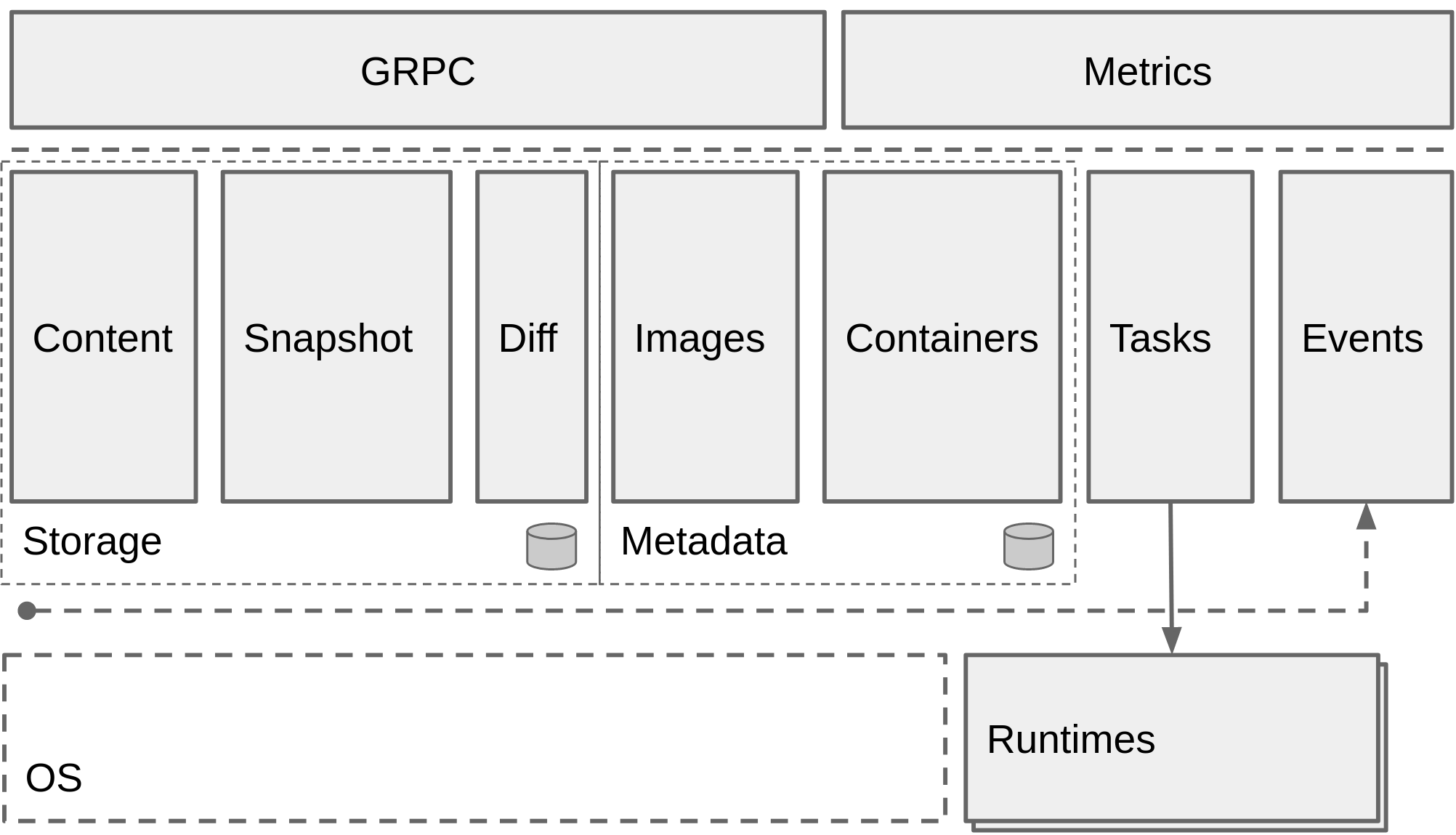 15 16 ## Getting Started 17 18 See our documentation on [containerd.io](https://containerd.io): 19 * [for ops and admins](docs/ops.md) 20 * [namespaces](docs/namespaces.md) 21 * [client options](docs/client-opts.md) 22 23 See how to build containerd from source at [BUILDING](BUILDING.md). 24 25 If you are interested in trying out containerd see our example at [Getting Started](docs/getting-started.md). 26 27 28 ## Runtime Requirements 29 30 Runtime requirements for containerd are very minimal. Most interactions with 31 the Linux and Windows container feature sets are handled via [runc](https://github.com/opencontainers/runc) and/or 32 OS-specific libraries (e.g. [hcsshim](https://github.com/Microsoft/hcsshim) for Microsoft). The current required version of `runc` is always listed in [RUNC.md](/RUNC.md). 33 34 There are specific features 35 used by containerd core code and snapshotters that will require a minimum kernel 36 version on Linux. With the understood caveat of distro kernel versioning, a 37 reasonable starting point for Linux is a minimum 4.x kernel version. 38 39 The overlay filesystem snapshotter, used by default, uses features that were 40 finalized in the 4.x kernel series. If you choose to use btrfs, there may 41 be more flexibility in kernel version (minimum recommended is 3.18), but will 42 require the btrfs kernel module and btrfs tools to be installed on your Linux 43 distribution. 44 45 To use Linux checkpoint and restore features, you will need `criu` installed on 46 your system. See more details in [Checkpoint and Restore](#checkpoint-and-restore). 47 48 Build requirements for developers are listed in [BUILDING](BUILDING.md). 49 50 ## Features 51 52 ### Client 53 54 containerd offers a full client package to help you integrate containerd into your platform. 55 56 ```go 57 58 import ( 59 "github.com/containerd/containerd" 60 "github.com/containerd/containerd/cio" 61 ) 62 63 64 func main() { 65 client, err := containerd.New("/run/containerd/containerd.sock") 66 defer client.Close() 67 } 68 69 ``` 70 71 ### Namespaces 72 73 Namespaces allow multiple consumers to use the same containerd without conflicting with each other. It has the benefit of sharing content but still having separation with containers and images. 74 75 To set a namespace for requests to the API: 76 77 ```go 78 context = context.Background() 79 // create a context for docker 80 docker = namespaces.WithNamespace(context, "docker") 81 82 containerd, err := client.NewContainer(docker, "id") 83 ``` 84 85 To set a default namespace on the client: 86 87 ```go 88 client, err := containerd.New(address, containerd.WithDefaultNamespace("docker")) 89 ``` 90 91 ### Distribution 92 93 ```go 94 // pull an image 95 image, err := client.Pull(context, "docker.io/library/redis:latest") 96 97 // push an image 98 err := client.Push(context, "docker.io/library/redis:latest", image.Target()) 99 ``` 100 101 ### Containers 102 103 In containerd, a container is a metadata object. Resources such as an OCI runtime specification, image, root filesystem, and other metadata can be attached to a container. 104 105 ```go 106 redis, err := client.NewContainer(context, "redis-master") 107 defer redis.Delete(context) 108 ``` 109 110 ### OCI Runtime Specification 111 112 containerd fully supports the OCI runtime specification for running containers. We have built in functions to help you generate runtime specifications based on images as well as custom parameters. 113 114 You can specify options when creating a container about how to modify the specification. 115 116 ```go 117 redis, err := client.NewContainer(context, "redis-master", containerd.WithNewSpec(oci.WithImageConfig(image))) 118 ``` 119 120 ### Root Filesystems 121 122 containerd allows you to use overlay or snapshot filesystems with your containers. It comes with builtin support for overlayfs and btrfs. 123 124 ```go 125 // pull an image and unpack it into the configured snapshotter 126 image, err := client.Pull(context, "docker.io/library/redis:latest", containerd.WithPullUnpack) 127 128 // allocate a new RW root filesystem for a container based on the image 129 redis, err := client.NewContainer(context, "redis-master", 130 containerd.WithNewSnapshot("redis-rootfs", image), 131 containerd.WithNewSpec(oci.WithImageConfig(image)), 132 ) 133 134 // use a readonly filesystem with multiple containers 135 for i := 0; i < 10; i++ { 136 id := fmt.Sprintf("id-%s", i) 137 container, err := client.NewContainer(ctx, id, 138 containerd.WithNewSnapshotView(id, image), 139 containerd.WithNewSpec(oci.WithImageConfig(image)), 140 ) 141 } 142 ``` 143 144 ### Tasks 145 146 Taking a container object and turning it into a runnable process on a system is done by creating a new `Task` from the container. A task represents the runnable object within containerd. 147 148 ```go 149 // create a new task 150 task, err := redis.NewTask(context, cio.Stdio) 151 defer task.Delete(context) 152 153 // the task is now running and has a pid that can be use to setup networking 154 // or other runtime settings outside of containerd 155 pid := task.Pid() 156 157 // start the redis-server process inside the container 158 err := task.Start(context) 159 160 // wait for the task to exit and get the exit status 161 status, err := task.Wait(context) 162 ``` 163 164 ### Checkpoint and Restore 165 166 If you have [criu](https://criu.org/Main_Page) installed on your machine you can checkpoint and restore containers and their tasks. This allow you to clone and/or live migrate containers to other machines. 167 168 ```go 169 // checkpoint the task then push it to a registry 170 checkpoint, err := task.Checkpoint(context) 171 172 err := client.Push(context, "myregistry/checkpoints/redis:master", checkpoint) 173 174 // on a new machine pull the checkpoint and restore the redis container 175 checkpoint, err := client.Pull(context, "myregistry/checkpoints/redis:master") 176 177 redis, err = client.NewContainer(context, "redis-master", containerd.WithNewSnapshot("redis-rootfs", checkpoint)) 178 defer container.Delete(context) 179 180 task, err = redis.NewTask(context, cio.Stdio, containerd.WithTaskCheckpoint(checkpoint)) 181 defer task.Delete(context) 182 183 err := task.Start(context) 184 ``` 185 186 ### Snapshot Plugins 187 188 In addition to the built-in Snapshot plugins in containerd, additional external 189 plugins can be configured using GRPC. An external plugin is made available using 190 the configured name and appears as a plugin alongside the built-in ones. 191 192 To add an external snapshot plugin, add the plugin to containerd's config file 193 (by default at `/etc/containerd/config.toml`). The string following 194 `proxy_plugin.` will be used as the name of the snapshotter and the address 195 should refer to a socket with a GRPC listener serving containerd's Snapshot 196 GRPC API. Remember to restart containerd for any configuration changes to take 197 effect. 198 199 ``` 200 [proxy_plugins] 201 [proxy_plugins.customsnapshot] 202 type = "snapshot" 203 address = "/var/run/mysnapshotter.sock" 204 ``` 205 206 See [PLUGINS.md](PLUGINS.md) for how to create plugins 207 208 ### Releases and API Stability 209 210 Please see [RELEASES.md](RELEASES.md) for details on versioning and stability 211 of containerd components. 212 213 ### Communication 214 215 For async communication and long running discussions please use issues and pull requests on the github repo. 216 This will be the best place to discuss design and implementation. 217 218 For sync communication we have a community slack with a #containerd channel that everyone is welcome to join and chat about development. 219 220 **Slack:** Catch us in the #containerd and #containerd-dev channels on dockercommunity.slack.com. 221 [Click here for an invite to docker community slack.](https://dockr.ly/slack) 222 223 ### Security audit 224 225 A third party security audit was performed by Cure53 in 4Q2018; the [full report](docs/SECURITY_AUDIT.pdf) is available in our docs/ directory. 226 227 ### Reporting security issues 228 229 __If you are reporting a security issue, please reach out discreetly at security@containerd.io__. 230 231 ## Licenses 232 233 The containerd codebase is released under the [Apache 2.0 license](LICENSE.code). 234 The README.md file, and files in the "docs" folder are licensed under the 235 Creative Commons Attribution 4.0 International License. You may obtain a 236 copy of the license, titled CC-BY-4.0, at http://creativecommons.org/licenses/by/4.0/. 237 238 ## Project details 239 240 **containerd** is the primary open source project within the broader containerd GitHub repository. 241 However, all projects within the repo have common maintainership, governance, and contributing 242 guidelines which are stored in a `project` repository commonly for all containerd projects. 243 244 Please find all these core project documents, including the: 245 * [Project governance](https://github.com/containerd/project/blob/master/GOVERNANCE.md), 246 * [Maintainers](https://github.com/containerd/project/blob/master/MAINTAINERS), 247 * and [Contributing guidelines](https://github.com/containerd/project/blob/master/CONTRIBUTING.md) 248 249 information in our [`containerd/project`](https://github.com/containerd/project) repository. 250 251 ## Adoption 252 253 Interested to see who is using containerd? Are you using containerd in a project? 254 Please add yourself via pull request to our [ADOPTERS.md](./ADOPTERS.md) file.