github.com/erda-project/erda-infra@v1.0.10-0.20240327085753-f3a249292aeb/README.md (about) 1 # Erda Infra 2 3 [](https://pkg.go.dev/github.com/erda-project/erda-infra) 4 [](https://www.apache.org/licenses/LICENSE-2.0.html) 5 [](https://codecov.io/gh/erda-project/erda-infra) 6 7 Translations: [English](README.md) | [简体中文](README_zh.md) 8 9 Erda Infra is a lightweight microservices framework implements by golang, which offers many useful modules and tools to help you quickly build a module-driven application. 10 11 Many Go projects are built using Erda Infra including: 12 * [Erda](https://github.com/erda-project/erda) 13 14 ## Features 15 * modular design to drive the implementation of the application system, and each module is pluggable. 16 * each module is configurable, supports setting defaults、reading from files (YAML, HCL,、JSON、TOML、.env file)、environment、flags. 17 * manage the lifecycle of the module, includes initialization, startup, and shutdown. 18 * manage dependencies between modules. 19 * support Dependency Injection between modules. 20 * offers many commonly modules that can be used directly. 21 * support define APIs and models in protobuf file to expose both gRPC and HTTP APIs. 22 * offers tools to help you quickly build a module. 23 * etc. 24 25 ## Concept 26 * Service, represents a function. 27 * Provider, service provider, equivalent to module, provide some services. It can also depend on other services, witch provide by other provider. 28 * ProviderDefine, describe a provider, includes provider's name, constructor function of provider, services, etc. Register it by *servicehub.RegisterProvider* function. 29 * Hub, is a container for all providers, and manage the life cycle of all loaded providers. 30 31 A configuration is used to determine whether all registered Providers are loaded, and the Hub initializes, starts, and closes the loaded Providers. 32 33 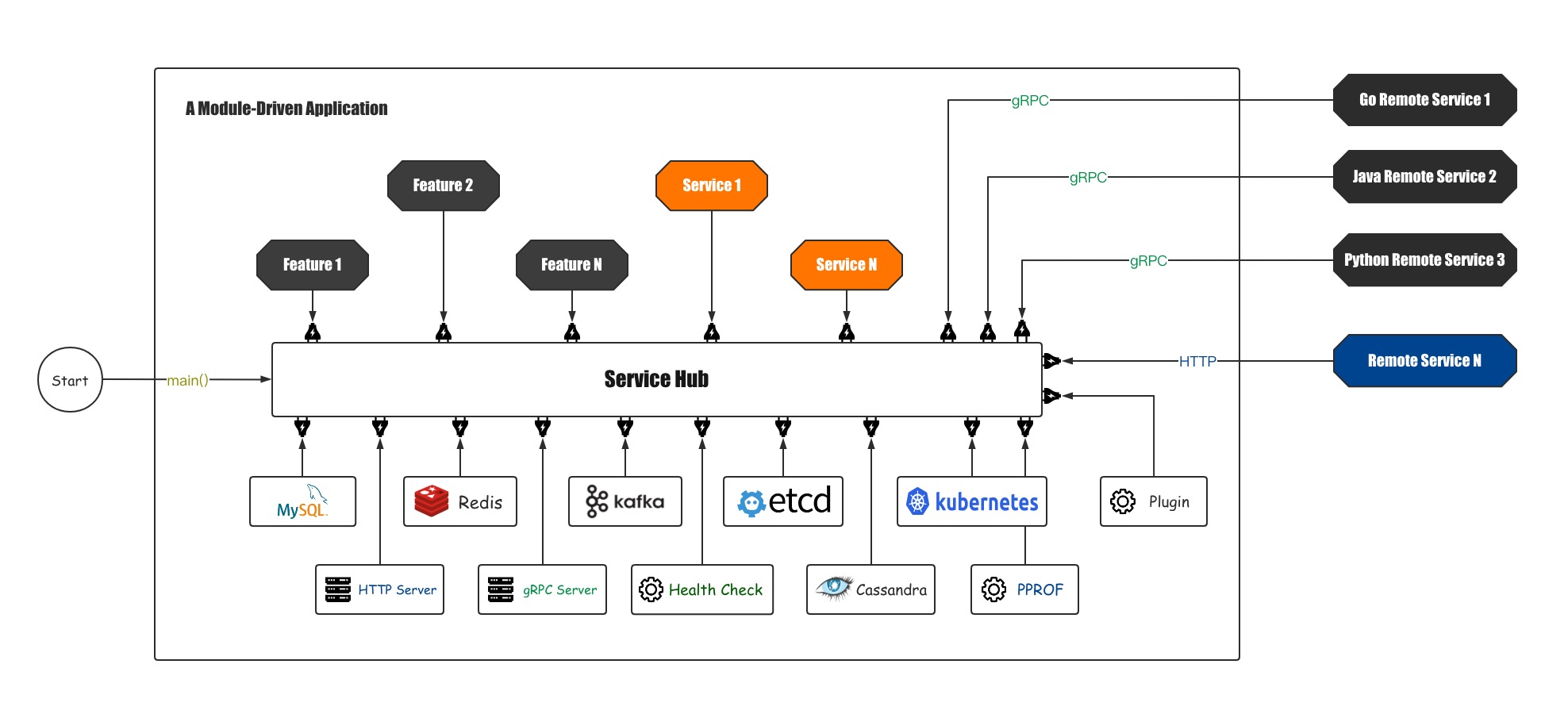 34 35 ## Define Provider 36 Define a provider by implementing the *servicehub.ProviderDefine* interface, and register it through the *servicehub.RegisterProvider* function. 37 38 But, it is simpler to describe a provider through *servicehub.Spec* and register it through the *servicehub.Register* function. 39 40 [Examples](./base/servicehub/examples) 41 42 ## Quick Start 43 ### Create a Provider 44 **Step 1**, Create a Provider 45 ```sh 46 ➜ gohub init -o helloworld 47 Input Service Provider Name: helloworld 48 ➜ tree helloworld 49 helloworld 50 ├── provider.go 51 └── provider_test.go 52 ``` 53 54 **Step 2**, Create *main.go* 55 ```go 56 package main 57 58 import ( 59 "github.com/erda-project/erda-infra/base/servicehub" 60 _ "./helloworld" // your package import path 61 ) 62 63 func main() { 64 servicehub.Run(&servicehub.RunOptions{ 65 Content: ` 66 helloworld: 67 `, 68 }) 69 } 70 ``` 71 72 **Step 3**, Run 73 ```sh 74 ➜ go run main.go 75 INFO[2021-04-13 13:17:36.416] message: hi module=helloworld 76 INFO[2021-04-13 13:17:36.416] provider helloworld initialized 77 INFO[2021-04-13 13:17:36.416] signals to quit: [hangup interrupt terminated quit] 78 INFO[2021-04-13 13:17:36.426] provider helloworld running ... 79 INFO[2021-04-13 13:17:39.429] do something... module=helloworld 80 ``` 81 [Hello World](./examples/example) \( [helloworld/](./examples/example/helloworld) | [main.go](./examples/example/main.go) \) 82 83 ### Create HTTP/gRPC Service 84 These services can be called either remote or local Provider. 85 86 **Step 1**, Define the protocol, in the *.proto files, includes Message and Interface. 87 ```protobuf 88 syntax = "proto3"; 89 90 package erda.infra.example; 91 import "google/api/annotations.proto"; 92 option go_package = "github.com/erda-project/erda-infra/examples/service/protocol/pb"; 93 94 // the greeting service definition. 95 service GreeterService { 96 // say hello 97 rpc SayHello (HelloRequest) returns (HelloResponse) { 98 option (google.api.http) = { 99 get: "/api/greeter/{name}", 100 }; 101 } 102 } 103 104 message HelloRequest { 105 string name = 1; 106 } 107 108 message HelloResponse { 109 bool success = 1; 110 string data = 2; 111 } 112 ``` 113 114 **Step 2**, build protocol to codes 115 ```sh 116 ➜ gohub protoc protocol *.proto 117 ➜ tree 118 . 119 ├── client 120 │ ├── client.go 121 │ └── provider.go 122 ├── greeter.proto 123 └── pb 124 ├── greeter.form.pb.go 125 ├── greeter.http.pb.go 126 ├── greeter.pb.go 127 ├── greeter_grpc.pb.go 128 └── register.services.pb.go 129 ``` 130 131 **Step 3**, implement the interface 132 ```sh 133 ➜ gohub protoc imp *.proto --imp_out=../server/helloworld 134 ➜ tree ../server/helloworld 135 ../server/helloworld 136 ├── greeter.service.go 137 ├── greeter.service_test.go 138 └── provider.go 139 ``` 140 141 **Step 4**, Create *main.go* and run it 142 143 *main.go* 144 ``` 145 package main 146 147 import ( 148 "os" 149 150 "github.com/erda-project/erda-infra/base/servicehub" 151 152 // import all providers 153 _ "github.com/erda-project/erda-infra/examples/service/server/helloworld" 154 _ "github.com/erda-project/erda-infra/providers" 155 ) 156 157 func main() { 158 hub := servicehub.New() 159 hub.Run("server", "server.yaml", os.Args...) 160 } 161 ``` 162 163 *server.yaml* 164 ```yaml 165 # optional 166 http-server: 167 addr: ":8080" 168 grpc-server: 169 addr: ":7070" 170 service-register: 171 # expose services and interface 172 erda.infra.example: 173 ``` 174 175 [Service](./examples/service) \( [Protocol](./examples/service/protocol) | [Implementation](./examples/service/server/helloworld) | [Server](./examples/service/server) | [Caller](./examples/service/caller) | [Client](./examples/service/client) \) 176 177 ## Useful Providers 178 Many available providers have been packaged in this project, it can be found in the [providers/](./providers) directory. 179 180 Under each module, there is an examples directory, which contains examples of the use of the module. 181 182 * elasticsearch, provide elasticsearch client APIs, and it easy to write batch data. 183 * etcd, provide etcd client APIs. 184 * etcd-mutex, distributed lock implemented by etcd. 185 * grpcserver, start a grpc server. 186 * grpcclient, provide gRPC client, and manage client's connection. 187 * health, provide health check API, and can register some health check function into this provider. 188 * httpserver, provide an HTTP server, support any form of handle function, interceptor, parameter binding, parameter verification, etc. 189 * i18n, provide internationalization support, manage i18n files, support templates. 190 * kafka, provide kafka sdk, and easy to produce and consume messages. 191 * kubernetes, provide kubernetes client APIs. 192 * mysql, provide mysql client APIs. 193 * pprof, expose pprof HTTP APIs By httpserver. 194 * redis, provide redis client APIs. 195 * zk-master-election, provide interface about master-slave election, it is implemented by zookeeper. 196 * zookeeper, provide zookeeper client. 197 * cassandra, provide cassandra APIs, and easy to write batch data 198 * serviceregister, use it to register services to expose gRPC and HTTP APIs. 199 200 # Tools 201 *gohub* is a CLI tool, which to help you quickly build a Provider. It can be installed as follows: 202 ```sh 203 go get -u github.com/erda-project/erda-infra/tools/gohub 204 ``` 205 206 You can also use *gohub* through a Docker container. 207 ```sh 208 ➜ docker run --rm -ti -v $(pwd):/go \ 209 registry.cn-hangzhou.aliyuncs.com/dice/erda-tools:1.0 gohub 210 Usage: 211 gohub [flags] 212 gohub [command] 213 214 Available Commands: 215 help Help about any command 216 init Initialize a provider with name 217 pkgpath Print the absolute path of go package 218 protoc ProtoBuf compiler tools 219 tools Tools 220 version Print the version number 221 222 Flags: 223 -h, --help help for gohub 224 225 Use "gohub [command] --help" for more information about a command. 226 ``` 227 228 ## License 229 Erda Infra is under the Apache 2.0 license. See the [LICENSE](/LICENSE) file for details.