github.com/kaituanwang/hyperledger@v2.0.1+incompatible/docs/source/developapps/contractname.md (about) 1 # Contract names 2 3 **Audience**: Architects, application and smart contract developers, 4 administrators 5 6 A chaincode is a generic container for deploying code to a Hyperledger Fabric 7 blockchain network. One or more related smart contracts are defined within a 8 chaincode. Every smart contract has a name that uniquely identifies it within a 9 chaincode. Applications access a particular smart contract within a chaincode 10 using its contract name. 11 12 In this topic, we're going to cover: 13 * [How a chaincode contains multiple smart contracts](#chaincode) 14 * [How to assign a smart contract name](#name) 15 * [How to use a smart contract from an application](#application) 16 * [The default smart contract](#default-contract) 17 18 ## Chaincode 19 20 In the [Developing Applications](./developing_applications.html) topic, we can 21 see how the Fabric SDKs provide high level programming abstractions which help 22 application and smart contract developers to focus on their business problem, 23 rather than the low level details of how to interact with a Fabric network. 24 25 Smart contracts are one example of a high level programming abstraction, and it 26 is possible to define smart contracts within in a chaincode container. When a 27 chaincode is installed on your peer and deployed to a channel, all the smart 28 contracts within it are made available to your applications. 29 30 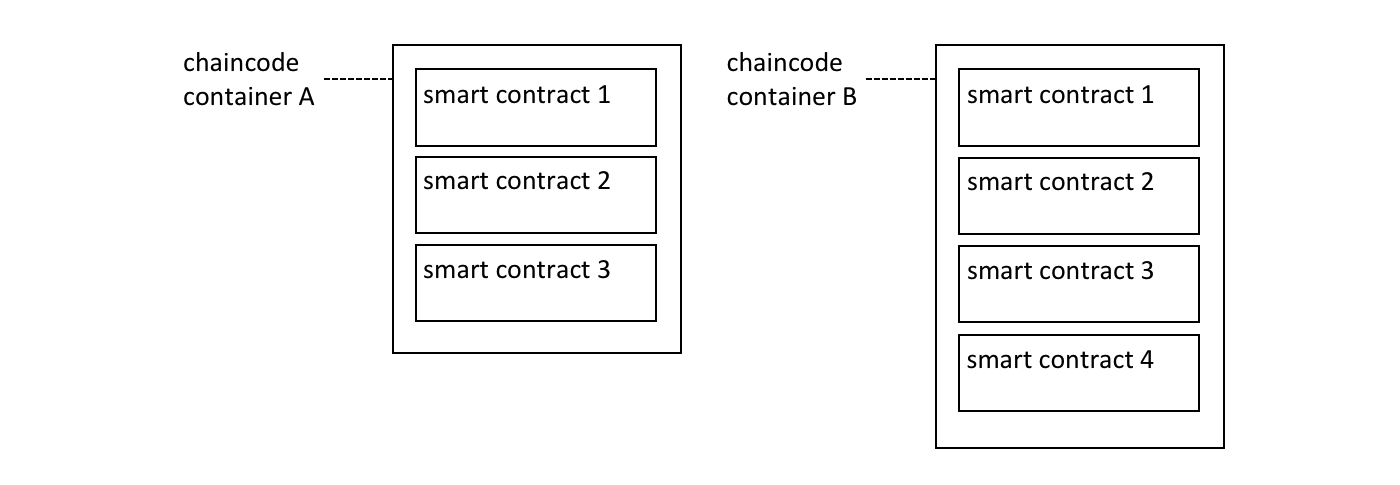 *Multiple smart contracts can be 31 defined within a chaincode. Each is uniquely identified by their name within a 32 chaincode.* 33 34 In the diagram [above](#chaincode), chaincode A has three smart contracts 35 defined within it, whereas chaincode B has four smart contracts. See how the 36 chaincode name is used to fully qualify a particular smart contract. 37 38 The ledger structure is defined by a set of deployed smart contracts. That's 39 because the ledger contains facts about the business objects of interest to the 40 network (such as commercial paper within PaperNet), and these business objects 41 are moved through their lifecycle (e.g. issue, buy, redeem) by the transaction 42 functions defined within a smart contract. 43 44 In most cases, a chaincode will only have one smart contract defined within it. 45 However, it can make sense to keep related smart contracts together in a single 46 chaincode. For example, commercial papers denominated in different currencies 47 might have contracts `EuroPaperContract`, `DollarPaperContract`, 48 `YenPaperContract` which might need to be kept synchronized with each other in 49 the channel to which they are deployed. 50 51 ## Name 52 53 Each smart contract within a chaincode is uniquely identified by its contract 54 name. A smart contract can explicitly assign this name when the class is 55 constructed, or let the `Contract` class implicitly assign a default name. 56 57 Examine the `papercontract.js` chaincode 58 [file](https://github.com/hyperledger/fabric-samples/blob/master/commercial-paper/organization/magnetocorp/contract/lib/papercontract.js#L31): 59 60 ```javascript 61 class CommercialPaperContract extends Contract { 62 63 constructor() { 64 // Unique name when multiple contracts per chaincode file 65 super('org.papernet.commercialpaper'); 66 } 67 ``` 68 69 See how the `CommercialPaperContract` constructor specifies the contract name as 70 `org.papernet.commercialpaper`. The result is that within the `papercontract` 71 chaincode, this smart contract is now associated with the contract name 72 `org.papernet.commercialpaper`. 73 74 If an explicit contract name is not specified, then a default name is assigned 75 -- the name of the class. In our example, the default contract name would be 76 `CommercialPaperContract`. 77 78 Choose your names carefully. It's not just that each smart contract must have a 79 unique name; a well-chosen name is illuminating. Specifically, using an explicit 80 DNS-style naming convention is recommended to help organize clear and meaningful 81 names; `org.papernet.commercialpaper` conveys that the PaperNet network has 82 defined a standard commercial paper smart contract. 83 84 Contract names are also helpful to disambiguate different smart contract 85 transaction functions with the same name in a given chaincode. This happens when 86 smart contracts are closely related; their transaction names will tend to be the 87 same. We can see that a transaction is uniquely defined within a channel by the 88 combination of its chaincode and smart contract name. 89 90 Contract names must be unique within a chaincode file. Some code editors will 91 detect multiple definitions of the same class name before deployment. Regardless 92 the chaincode will return an error if multiple classes with the same contract 93 name are explicitly or implicitly specified. 94 95 ## Application 96 97 Once a chaincode has been installed on a peer and deployed to a channel, the 98 smart contracts in it are accessible to an application: 99 100 ```javascript 101 const network = await gateway.getNetwork(`papernet`); 102 103 const contract = await network.getContract('papercontract', 'org.papernet.commercialpaper'); 104 105 const issueResponse = await contract.submitTransaction('issue', 'MagnetoCorp', '00001', '2020-05-31', '2020-11-30', '5000000'); 106 ``` 107 108 See how the application accesses the smart contract with the 109 `network.getContract()` method. The `papercontract` chaincode name 110 `org.papernet.commercialpaper` returns a `contract` reference which can be 111 used to submit transactions to issue commercial paper with the 112 `contract.submitTransaction()` API. 113 114 ## Default contract 115 116 The first smart contract defined in a chaincode is called the *default* 117 smart contract. A default is helpful because a chaincode will usually have one 118 smart contract defined within it; a default allows the application to access 119 those transactions directly -- without specifying a contract name. 120 121 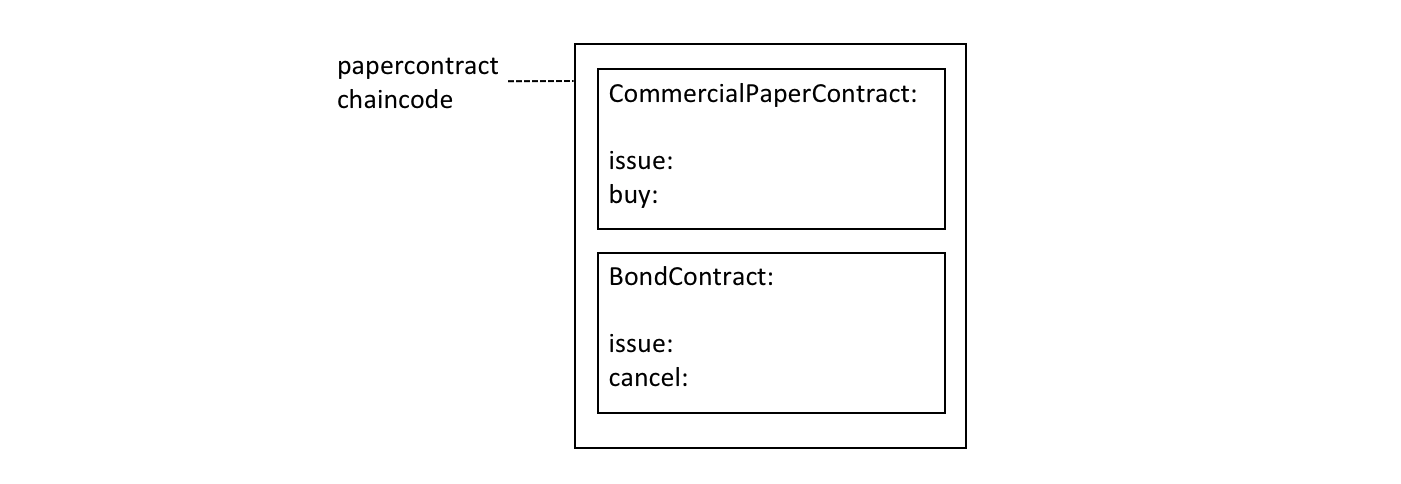 *A default smart contract is the 122 first contract defined in a chaincode.* 123 124 In this diagram, `CommercialPaperContract` is the default smart contract. Even 125 though we have two smart contracts, the default smart contract makes our 126 [previous](#application) example easier to write: 127 128 ```javascript 129 const network = await gateway.getNetwork(`papernet`); 130 131 const contract = await network.getContract('papercontract'); 132 133 const issueResponse = await contract.submitTransaction('issue', 'MagnetoCorp', '00001', '2020-05-31', '2020-11-30', '5000000'); 134 ``` 135 136 This works because the default smart contract in `papercontract` is 137 `CommercialPaperContract` and it has an `issue` transaction. Note that the 138 `issue` transaction in `BondContract` can only be invoked by explicitly 139 addressing it. Likewise, even though the `cancel` transaction is unique, because 140 `BondContract` is *not* the default smart contract, it must also be explicitly 141 addressed. 142 143 In most cases, a chaincode will only contain a single smart contract, so careful 144 naming of the chaincode can reduce the need for developers to care about 145 chaincode as a concept. In the example code [above](#default-contract) it feels 146 like `papercontract` is a smart contract. 147 148 In summary, contract names are a straightforward mechanism to identify 149 individual smart contracts within a given chaincode. Contract names make it easy 150 for applications to find a particular smart contract and use it to access the 151 ledger. 152 153 <!--- Licensed under Creative Commons Attribution 4.0 International License 154 https://creativecommons.org/licenses/by/4.0/ -->