github.com/kerryoscer/gqlgen@v0.17.29/README.md (about) 1 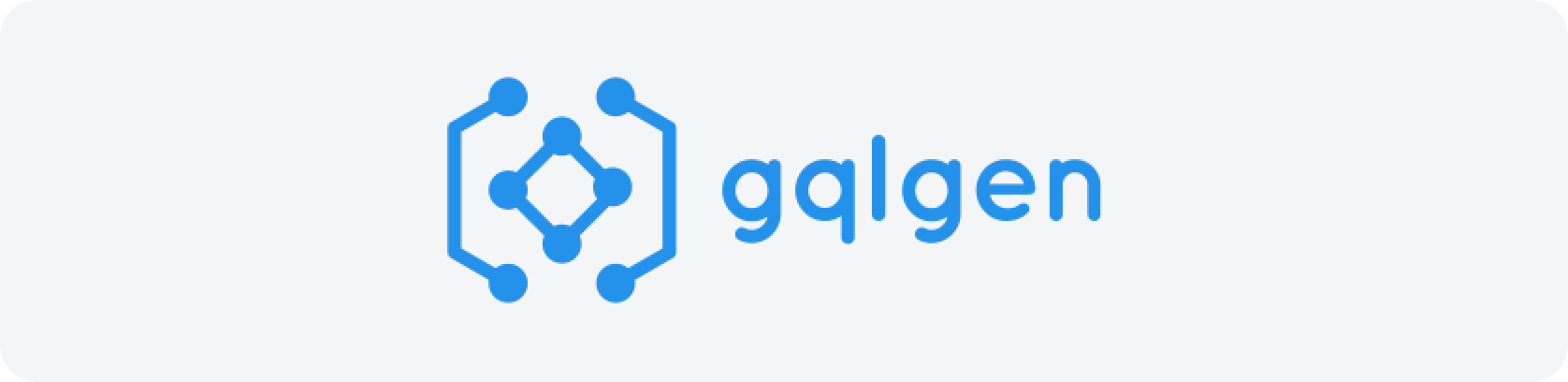 2 3 4 # gqlgen [](https://github.com/99designs/gqlgen/actions) [](https://coveralls.io/github/99designs/gqlgen?branch=master) [](https://goreportcard.com/report/github.com/99designs/gqlgen) [](https://pkg.go.dev/github.com/99designs/gqlgen) [](http://gqlgen.com/) 5 6 ## What is gqlgen? 7 8 [gqlgen](https://github.com/99designs/gqlgen) is a Go library for building GraphQL servers without any fuss.<br/> 9 10 - **gqlgen is based on a Schema first approach** — You get to Define your API using the GraphQL [Schema Definition Language](http://graphql.org/learn/schema/). 11 - **gqlgen prioritizes Type safety** — You should never see `map[string]interface{}` here. 12 - **gqlgen enables Codegen** — We generate the boring bits, so you can focus on building your app quickly. 13 14 Still not convinced enough to use **gqlgen**? Compare **gqlgen** with other Go graphql [implementations](https://gqlgen.com/feature-comparison/) 15 16 ## Quick start 17 1. [Initialise a new go module](https://golang.org/doc/tutorial/create-module) 18 19 mkdir example 20 cd example 21 go mod init example 22 23 2. Add `github.com/99designs/gqlgen` to your [project's tools.go](https://github.com/golang/go/wiki/Modules#how-can-i-track-tool-dependencies-for-a-module) 24 25 printf '// +build tools\npackage tools\nimport (_ "github.com/kerryoscer/gqlgen"\n _ "github.com/kerryoscer/gqlgen/graphql/introspection")' | gofmt > tools.go 26 27 go mod tidy 28 29 3. Initialise gqlgen config and generate models 30 31 go run github.com/99designs/gqlgen init 32 33 4. Start the graphql server 34 35 go run server.go 36 37 More help to get started: 38 - [Getting started tutorial](https://gqlgen.com/getting-started/) - a comprehensive guide to help you get started 39 - [Real-world examples](https://github.com/99designs/gqlgen/tree/master/_examples) show how to create GraphQL applications 40 - [Reference docs](https://pkg.go.dev/github.com/99designs/gqlgen) for the APIs 41 42 ## Reporting Issues 43 44 If you think you've found a bug, or something isn't behaving the way you think it should, please raise an [issue](https://github.com/99designs/gqlgen/issues) on GitHub. 45 46 ## Contributing 47 48 We welcome contributions, Read our [Contribution Guidelines](https://github.com/99designs/gqlgen/blob/master/CONTRIBUTING.md) to learn more about contributing to **gqlgen** 49 ## Frequently asked questions 50 51 ### How do I prevent fetching child objects that might not be used? 52 53 When you have nested or recursive schema like this: 54 55 ```graphql 56 type User { 57 id: ID! 58 name: String! 59 friends: [User!]! 60 } 61 ``` 62 63 You need to tell gqlgen that it should only fetch friends if the user requested it. There are two ways to do this; 64 65 - #### Using Custom Models 66 67 Write a custom model that omits the friends field: 68 69 ```go 70 type User struct { 71 ID int 72 Name string 73 } 74 ``` 75 76 And reference the model in `gqlgen.yml`: 77 78 ```yaml 79 # gqlgen.yml 80 models: 81 User: 82 model: github.com/you/pkg/model.User # go import path to the User struct above 83 ``` 84 85 - #### Using Explicit Resolvers 86 87 If you want to Keep using the generated model, mark the field as requiring a resolver explicitly in `gqlgen.yml` like this: 88 89 ```yaml 90 # gqlgen.yml 91 models: 92 User: 93 fields: 94 friends: 95 resolver: true # force a resolver to be generated 96 ``` 97 98 After doing either of the above and running generate we will need to provide a resolver for friends: 99 100 ```go 101 func (r *userResolver) Friends(ctx context.Context, obj *User) ([]*User, error) { 102 // select * from user where friendid = obj.ID 103 return friends, nil 104 } 105 ``` 106 107 You can also use inline config with directives to achieve the same result 108 109 ```graphql 110 directive @goModel(model: String, models: [String!]) on OBJECT 111 | INPUT_OBJECT 112 | SCALAR 113 | ENUM 114 | INTERFACE 115 | UNION 116 117 directive @goField(forceResolver: Boolean, name: String) on INPUT_FIELD_DEFINITION 118 | FIELD_DEFINITION 119 120 type User @goModel(model: "github.com/you/pkg/model.User") { 121 id: ID! @goField(name: "todoId") 122 friends: [User!]! @goField(forceResolver: true) 123 } 124 ``` 125 126 ### Can I change the type of the ID from type String to Type Int? 127 128 Yes! You can by remapping it in config as seen below: 129 130 ```yaml 131 models: 132 ID: # The GraphQL type ID is backed by 133 model: 134 - github.com/99designs/gqlgen/graphql.IntID # a go integer 135 - github.com/99designs/gqlgen/graphql.ID # or a go string 136 ``` 137 138 This means gqlgen will be able to automatically bind to strings or ints for models you have written yourself, but the 139 first model in this list is used as the default type and it will always be used when: 140 141 - Generating models based on schema 142 - As arguments in resolvers 143 144 There isn't any way around this, gqlgen has no way to know what you want in a given context. 145 146 ### Why do my interfaces have getters? Can I disable these? 147 These were added in v0.17.14 to allow accessing common interface fields without casting to a concrete type. 148 However, certain fields, like Relay-style Connections, cannot be implemented with simple getters. 149 150 If you'd prefer to not have getters generated in your interfaces, you can add the following in your `gqlgen.yml`: 151 ```yaml 152 # gqlgen.yml 153 omit_getters: true 154 ``` 155 156 ## Other Resources 157 158 - [Christopher Biscardi @ Gophercon UK 2018](https://youtu.be/FdURVezcdcw) 159 - [Introducing gqlgen: a GraphQL Server Generator for Go](https://99designs.com.au/blog/engineering/gqlgen-a-graphql-server-generator-for-go/) 160 - [Dive into GraphQL by Iván Corrales Solera](https://medium.com/@ivan.corrales.solera/dive-into-graphql-9bfedf22e1a) 161 - [Sample Project built on gqlgen with Postgres by Oleg Shalygin](https://github.com/oshalygin/gqlgen-pg-todo-example) 162 - [Hackernews GraphQL Server with gqlgen by Shayegan Hooshyari](https://www.howtographql.com/graphql-go/0-introduction/)