github.com/lalkh/containerd@v1.4.3/README.md (about) 1  2 3 [](https://godoc.org/github.com/containerd/containerd) 4 [](https://github.com/containerd/containerd/actions?query=workflow%3ACI) 5 [](https://ci.appveyor.com/project/mlaventure/containerd-3g73f?branch=master) 6 [](https://github.com/containerd/containerd/actions?query=workflow%3ANightly) 7 [](https://app.fossa.io/projects/git%2Bhttps%3A%2F%2Fgithub.com%2Fcontainerd%2Fcontainerd?ref=badge_shield) 8 [](https://goreportcard.com/report/github.com/containerd/containerd) 9 [](https://bestpractices.coreinfrastructure.org/projects/1271) 10 11 containerd is an industry-standard container runtime with an emphasis on simplicity, robustness and portability. It is available as a daemon for Linux and Windows, which can manage the complete container lifecycle of its host system: image transfer and storage, container execution and supervision, low-level storage and network attachments, etc. 12 13 containerd is designed to be embedded into a larger system, rather than being used directly by developers or end-users. 14 15 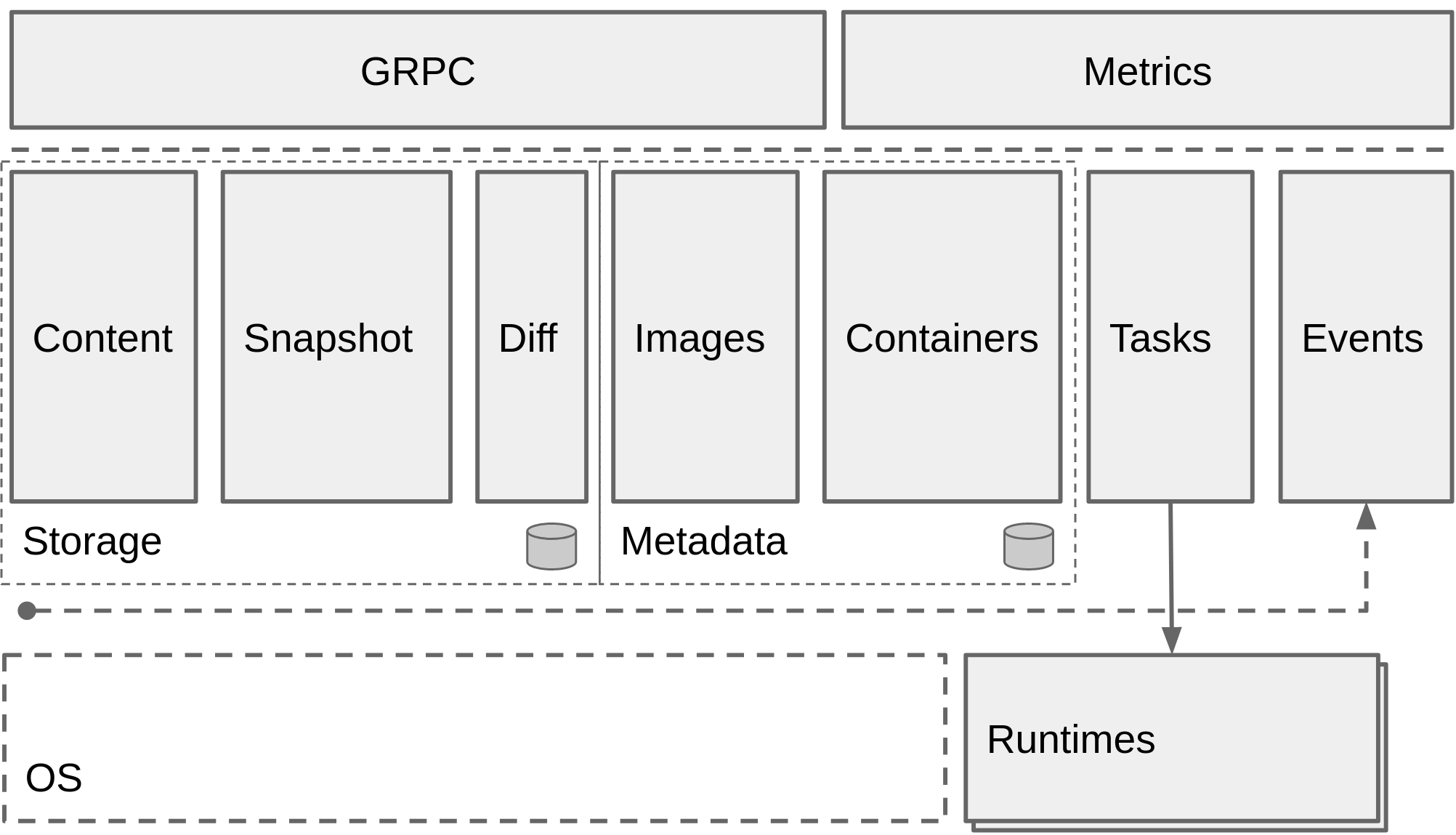 16 17 ## Getting Started 18 19 See our documentation on [containerd.io](https://containerd.io): 20 * [for ops and admins](docs/ops.md) 21 * [namespaces](docs/namespaces.md) 22 * [client options](docs/client-opts.md) 23 24 See how to build containerd from source at [BUILDING](BUILDING.md). 25 26 If you are interested in trying out containerd see our example at [Getting Started](docs/getting-started.md). 27 28 ## Nightly builds 29 30 There are nightly builds available for download [here](https://github.com/containerd/containerd/actions?query=workflow%3ANightly). 31 Binaries are generated from `master` branch every night for `Linux` and `Windows`. 32 33 Please be aware: nightly builds might have critical bugs, it's not recommended for use in prodution and no support provided. 34 35 ## Runtime Requirements 36 37 Runtime requirements for containerd are very minimal. Most interactions with 38 the Linux and Windows container feature sets are handled via [runc](https://github.com/opencontainers/runc) and/or 39 OS-specific libraries (e.g. [hcsshim](https://github.com/Microsoft/hcsshim) for Microsoft). The current required version of `runc` is always listed in [RUNC.md](/RUNC.md). 40 41 There are specific features 42 used by containerd core code and snapshotters that will require a minimum kernel 43 version on Linux. With the understood caveat of distro kernel versioning, a 44 reasonable starting point for Linux is a minimum 4.x kernel version. 45 46 The overlay filesystem snapshotter, used by default, uses features that were 47 finalized in the 4.x kernel series. If you choose to use btrfs, there may 48 be more flexibility in kernel version (minimum recommended is 3.18), but will 49 require the btrfs kernel module and btrfs tools to be installed on your Linux 50 distribution. 51 52 To use Linux checkpoint and restore features, you will need `criu` installed on 53 your system. See more details in [Checkpoint and Restore](#checkpoint-and-restore). 54 55 Build requirements for developers are listed in [BUILDING](BUILDING.md). 56 57 ## Features 58 59 ### Client 60 61 containerd offers a full client package to help you integrate containerd into your platform. 62 63 ```go 64 65 import ( 66 "github.com/containerd/containerd" 67 "github.com/containerd/containerd/cio" 68 ) 69 70 71 func main() { 72 client, err := containerd.New("/run/containerd/containerd.sock") 73 defer client.Close() 74 } 75 76 ``` 77 78 ### Namespaces 79 80 Namespaces allow multiple consumers to use the same containerd without conflicting with each other. It has the benefit of sharing content but still having separation with containers and images. 81 82 To set a namespace for requests to the API: 83 84 ```go 85 context = context.Background() 86 // create a context for docker 87 docker = namespaces.WithNamespace(context, "docker") 88 89 containerd, err := client.NewContainer(docker, "id") 90 ``` 91 92 To set a default namespace on the client: 93 94 ```go 95 client, err := containerd.New(address, containerd.WithDefaultNamespace("docker")) 96 ``` 97 98 ### Distribution 99 100 ```go 101 // pull an image 102 image, err := client.Pull(context, "docker.io/library/redis:latest") 103 104 // push an image 105 err := client.Push(context, "docker.io/library/redis:latest", image.Target()) 106 ``` 107 108 ### Containers 109 110 In containerd, a container is a metadata object. Resources such as an OCI runtime specification, image, root filesystem, and other metadata can be attached to a container. 111 112 ```go 113 redis, err := client.NewContainer(context, "redis-master") 114 defer redis.Delete(context) 115 ``` 116 117 ### OCI Runtime Specification 118 119 containerd fully supports the OCI runtime specification for running containers. We have built in functions to help you generate runtime specifications based on images as well as custom parameters. 120 121 You can specify options when creating a container about how to modify the specification. 122 123 ```go 124 redis, err := client.NewContainer(context, "redis-master", containerd.WithNewSpec(oci.WithImageConfig(image))) 125 ``` 126 127 ### Root Filesystems 128 129 containerd allows you to use overlay or snapshot filesystems with your containers. It comes with builtin support for overlayfs and btrfs. 130 131 ```go 132 // pull an image and unpack it into the configured snapshotter 133 image, err := client.Pull(context, "docker.io/library/redis:latest", containerd.WithPullUnpack) 134 135 // allocate a new RW root filesystem for a container based on the image 136 redis, err := client.NewContainer(context, "redis-master", 137 containerd.WithNewSnapshot("redis-rootfs", image), 138 containerd.WithNewSpec(oci.WithImageConfig(image)), 139 ) 140 141 // use a readonly filesystem with multiple containers 142 for i := 0; i < 10; i++ { 143 id := fmt.Sprintf("id-%s", i) 144 container, err := client.NewContainer(ctx, id, 145 containerd.WithNewSnapshotView(id, image), 146 containerd.WithNewSpec(oci.WithImageConfig(image)), 147 ) 148 } 149 ``` 150 151 ### Tasks 152 153 Taking a container object and turning it into a runnable process on a system is done by creating a new `Task` from the container. A task represents the runnable object within containerd. 154 155 ```go 156 // create a new task 157 task, err := redis.NewTask(context, cio.NewCreator(cio.WithStdio)) 158 defer task.Delete(context) 159 160 // the task is now running and has a pid that can be use to setup networking 161 // or other runtime settings outside of containerd 162 pid := task.Pid() 163 164 // start the redis-server process inside the container 165 err := task.Start(context) 166 167 // wait for the task to exit and get the exit status 168 status, err := task.Wait(context) 169 ``` 170 171 ### Checkpoint and Restore 172 173 If you have [criu](https://criu.org/Main_Page) installed on your machine you can checkpoint and restore containers and their tasks. This allow you to clone and/or live migrate containers to other machines. 174 175 ```go 176 // checkpoint the task then push it to a registry 177 checkpoint, err := task.Checkpoint(context) 178 179 err := client.Push(context, "myregistry/checkpoints/redis:master", checkpoint) 180 181 // on a new machine pull the checkpoint and restore the redis container 182 checkpoint, err := client.Pull(context, "myregistry/checkpoints/redis:master") 183 184 redis, err = client.NewContainer(context, "redis-master", containerd.WithNewSnapshot("redis-rootfs", checkpoint)) 185 defer container.Delete(context) 186 187 task, err = redis.NewTask(context, cio.NewCreator(cio.WithStdio), containerd.WithTaskCheckpoint(checkpoint)) 188 defer task.Delete(context) 189 190 err := task.Start(context) 191 ``` 192 193 ### Snapshot Plugins 194 195 In addition to the built-in Snapshot plugins in containerd, additional external 196 plugins can be configured using GRPC. An external plugin is made available using 197 the configured name and appears as a plugin alongside the built-in ones. 198 199 To add an external snapshot plugin, add the plugin to containerd's config file 200 (by default at `/etc/containerd/config.toml`). The string following 201 `proxy_plugin.` will be used as the name of the snapshotter and the address 202 should refer to a socket with a GRPC listener serving containerd's Snapshot 203 GRPC API. Remember to restart containerd for any configuration changes to take 204 effect. 205 206 ``` 207 [proxy_plugins] 208 [proxy_plugins.customsnapshot] 209 type = "snapshot" 210 address = "/var/run/mysnapshotter.sock" 211 ``` 212 213 See [PLUGINS.md](PLUGINS.md) for how to create plugins 214 215 ### Releases and API Stability 216 217 Please see [RELEASES.md](RELEASES.md) for details on versioning and stability 218 of containerd components. 219 220 Downloadable 64-bit Intel/AMD binaries of all official releases are available on 221 our [releases page](https://github.com/containerd/containerd/releases), as well as 222 auto-published to the [cri-containerd-release storage bucket](https://console.cloud.google.com/storage/browser/cri-containerd-release?pli=1). 223 224 For other architectures and distribution support, you will find that many 225 Linux distributions package their own containerd and provide it across several 226 architectures, such as [Canonical's Ubuntu packaging](https://launchpad.net/ubuntu/bionic/+package/containerd). 227 228 #### Enabling command auto-completion 229 230 Starting with containerd 1.4, the urfave client feature for auto-creation of bash and zsh 231 autocompletion data is enabled. To use the autocomplete feature in a bash shell for example, source 232 the autocomplete/ctr file in your `.bashrc`, or manually like: 233 234 ``` 235 $ source ./contrib/autocomplete/ctr 236 ``` 237 238 #### Distribution of `ctr` autocomplete for bash and zsh 239 240 For bash, copy the `contrib/autocomplete/ctr` script into 241 `/etc/bash_completion.d/` and rename it to `ctr`. The `zsh_autocomplete` 242 file is also available and can be used similarly for zsh users. 243 244 Provide documentation to users to `source` this file into their shell if 245 you don't place the autocomplete file in a location where it is automatically 246 loaded for the user's shell environment. 247 248 ### Communication 249 250 For async communication and long running discussions please use issues and pull requests on the github repo. 251 This will be the best place to discuss design and implementation. 252 253 For sync communication we have a community slack with a #containerd channel that everyone is welcome to join and chat about development. 254 255 **Slack:** Catch us in the #containerd and #containerd-dev channels on dockercommunity.slack.com. 256 [Click here for an invite to docker community slack.](https://dockr.ly/slack) 257 258 ### Security audit 259 260 A third party security audit was performed by Cure53 in 4Q2018; the [full report](docs/SECURITY_AUDIT.pdf) is available in our docs/ directory. 261 262 ### Reporting security issues 263 264 __If you are reporting a security issue, please reach out discreetly at security@containerd.io__. 265 266 ## Licenses 267 268 The containerd codebase is released under the [Apache 2.0 license](LICENSE). 269 The README.md file, and files in the "docs" folder are licensed under the 270 Creative Commons Attribution 4.0 International License. You may obtain a 271 copy of the license, titled CC-BY-4.0, at http://creativecommons.org/licenses/by/4.0/. 272 273 ## Project details 274 275 **containerd** is the primary open source project within the broader containerd GitHub repository. 276 However, all projects within the repo have common maintainership, governance, and contributing 277 guidelines which are stored in a `project` repository commonly for all containerd projects. 278 279 Please find all these core project documents, including the: 280 * [Project governance](https://github.com/containerd/project/blob/master/GOVERNANCE.md), 281 * [Maintainers](https://github.com/containerd/project/blob/master/MAINTAINERS), 282 * and [Contributing guidelines](https://github.com/containerd/project/blob/master/CONTRIBUTING.md) 283 284 information in our [`containerd/project`](https://github.com/containerd/project) repository. 285 286 ## Adoption 287 288 Interested to see who is using containerd? Are you using containerd in a project? 289 Please add yourself via pull request to our [ADOPTERS.md](./ADOPTERS.md) file.