github.com/machinebox/remoto@v0.1.2-0.20191024144331-eff21a7d321f/README.md (about) 1 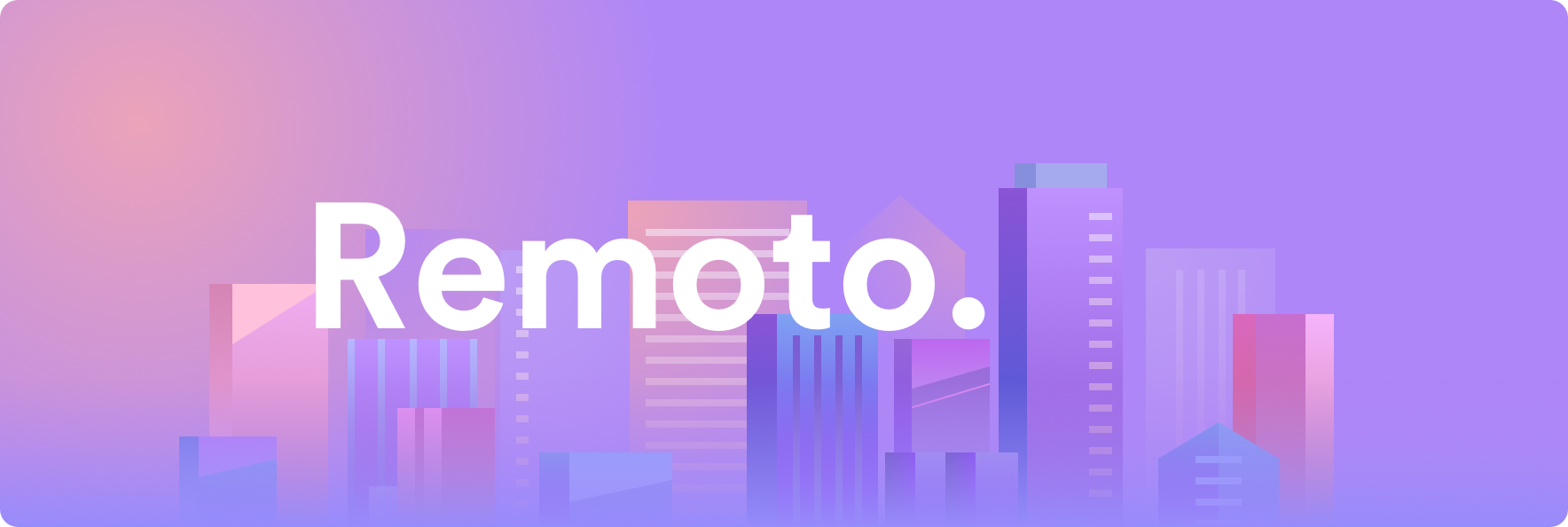 2 3 # Remoto 4 5 Ultra-simple, fast, complete RPC ecosystem designed for right now. 6 7 * Simple service definitions written in Go (interfaces and structs) 8 * Generates servers and clients that makes implementing/consuming easy 9 * Generates human-readable code 10 * Supports batch requests out of the box 11 * Lots of [templates](templates) to use today 12 * Modify templates or write your own 13 14 ## In this document 15 16 * [Introduction](#introduction) - Start learning about Remoto in the introduction 17 * [Remoto Definition Files](#remoto-definition-files) - An overview of the Remoto definition files 18 * [Remoto command](#remoto-command) - The `remoto` command line tool 19 * [remotohttp](#remotohttp) - Templates for Remoto powered HTTP servers and clients 20 21 # Introduction 22 23 Remoto is an RPC ecosystem and code generation tool. A powerful set of templates and 24 supporting code allows you to quickly spin up RPC services, and consume them with hand crafted 25 client libraries by experts in each particular language. 26 27 ## Who is Remoto for? 28 29 Remoto is for teams who want to: 30 31 * Deliver mobile and web RPC services 32 * Automate SDK and client library generation 33 * Stick with simple and familiar technology 34 35 # Remoto definition files 36 37 Definition files are Go source with `.remoto.go` file extension. 38 39 An example definition looks like this: 40 41 ```go 42 package project 43 44 // Greeter provides greeting services. 45 type Greeter interface { 46 // Greet generates a greeting. 47 Greet(GreetRequest) GreetResponse 48 } 49 50 // GreetRequest is the request for Greeter.GreetRequest. 51 type GreetRequest struct { 52 Name string 53 } 54 55 // GreetResponse is the response for Greeter.GreetRequest. 56 type GreetResponse struct { 57 Greeting string 58 } 59 ``` 60 61 * `package project` - package name can group services 62 * `type ServiceName interface` - describes an RPC service 63 * `Greet(GreetRequest) GreetResponse` - service method with request and response objects 64 * `type GreetRequest struct` - describes the request data 65 * `type GreetResponse struct` - describes the response data 66 67 ## Rules 68 69 * Each service is an `interface` 70 * Each method is an endpoint 71 * Methods must take a request object as its only argument 72 * Methods must return the response object as the result 73 * Only a subset of Go types are supported: `string`, `float64`, `int`, `bool`, and `struct` types 74 * Any arrays (slices) of the supported types are also allowed (e.g. `[]string`, `[]bool`, etc.) 75 * Comments describe the services, methods and types 76 * Do not import packages (apart from official Remoto ones), instead your definition files should be self contained 77 78 ## Special types 79 80 * Learn more about specially handled types in [remototypes](remototypes). 81 82 ## Tips 83 84 * Avoid importing common types - describe all the required types in a single `.remoto.go` file 85 86 # Remoto command 87 88 The `remoto` command can be built into your development pipeline to generate source code from 89 definition files. 90 91 ``` 92 usage: 93 remoto sub-command 94 ``` 95 96 ## Generate 97 98 The generate command generates source code from a given template. 99 100 ``` 101 usage: 102 remoto generate definition template -o output-file 103 ``` 104 105 * `definition` - Path to the definition file 106 * `template` - Path to the template to render 107 * `output-file` - Where to save the output (folders will be created and files will be overwritten without warning) 108 109 # remotohttp 110 111 As well as code generation, Remoto ships with a complete HTTP client/server implementation which you can generate from your definition files. 112 113 For more information, see the [remotohttp documentation](go/remotohttp).