github.com/maeglindeveloper/gqlgen@v0.13.1-0.20210413081235-57808b12a0a0/docs/content/recipes/migration-0.11.md (about) 1 --- 2 title: "Migrating to 0.11" 3 description: Changes in gqlgen 0.11 4 linkTitle: Migrating to 0.11 5 menu: { main: { parent: 'recipes' } } 6 --- 7 8 ## Updated gqlparser 9 10 gqlparser had a breaking change, if you have any references to it in your project your going to need to update 11 them from `github.com/vektah/gqlparser` to `github.com/vektah/gqlparser/v2`. 12 13 ```bash 14 sed -i 's/github.com\/vektah\/gqlparser/github.com\/vektah\/gqlparser\/v2/' $(find -name '*.go') 15 ``` 16 17 ## Handler Refactor 18 19 The handler package has grown organically for a long time, 0.11 is a large cleanup of the handler package to make it 20 more modular and easier to maintain once we get to 1.0. 21 22 23 ### Transports 24 25 Transports are the first thing that run, they handle decoding the incoming http request, and encoding the graphql 26 response. Supported transports are: 27 28 - GET 29 - JSON POST 30 - Multipart form 31 - Websockets 32 33 new usage looks like this 34 ```go 35 srv := New(es) 36 37 srv.AddTransport(transport.Websocket{ 38 KeepAlivePingInterval: 10 * time.Second, 39 }) 40 srv.AddTransport(transport.Options{}) 41 srv.AddTransport(transport.GET{}) 42 srv.AddTransport(transport.POST{}) 43 srv.AddTransport(transport.MultipartForm{}) 44 ``` 45 46 ### New handler extension API 47 48 The core of this changes the handler package to be a set of composable extensions. The extensions implement a set of optional interfaces: 49 50 - **OperationParameterMutator** runs before creating a OperationContext (formerly RequestContext). allows manipulating the raw query before parsing. 51 - **OperationContextMutator** runs after creating the OperationContext, but before executing the root resolver. 52 - **OperationInterceptor** runs for each incoming query after parsing and validation, for basic requests the writer will be invoked once, for subscriptions it will be invoked multiple times. 53 - **ResponseInterceptor** runs around each graphql operation response. This can be called many times for a single operation the case of subscriptions. 54 - **FieldInterceptor** runs around each field 55 56 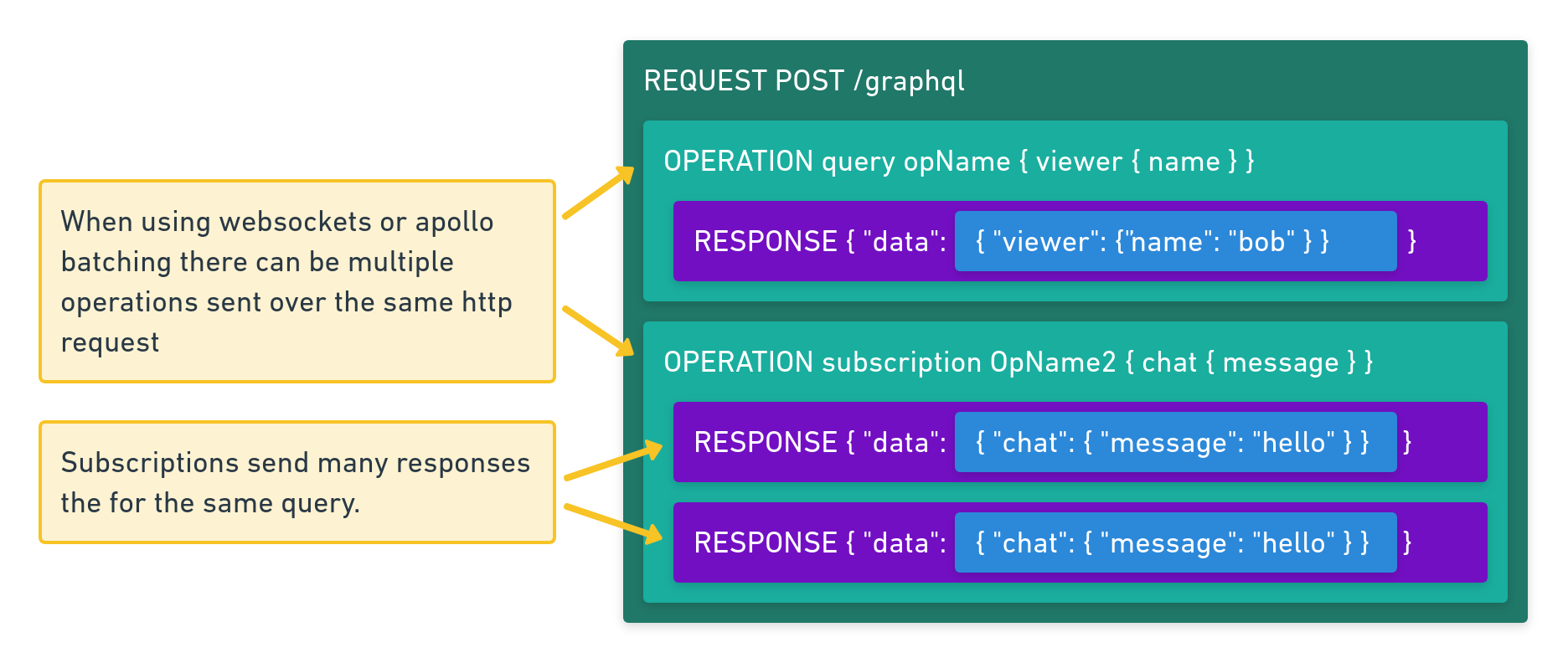 57 58 Users of an extension should not need to know which extension points are being used by a given extension, they are added to the server simply by calling `Use(extension)`. 59 60 There are a few convenience methods for defining middleware inline, instead of creating an extension 61 62 ```go 63 srv := handler.New(es) 64 srv.AroundFields(func(ctx context.Context, next graphql.Resolver) (res interface{}, err error) { 65 // this function will be called around every field. next() will evaluate the field and return 66 // its computed value. 67 return next(ctx) 68 }) 69 srv.AroundOperations(func(ctx context.Context, next graphql.OperationHandler) graphql.ResponseHandler { 70 // This function will be called around every operation, next() will return a function that when 71 // called will evaluate one response. Eventually next will return nil, signalling there are no 72 // more results to be returned by the server. 73 return next(ctx) 74 }) 75 srv.AroundResponses(func(ctx context.Context, next graphql.ResponseHandler) *graphql.Response { 76 // This function will be called around each response in the operation. next() will evaluate 77 // and return a single response. 78 return next(ctx) 79 }) 80 ``` 81 82 83 Some of the features supported out of the box by handler extensions: 84 85 - APQ 86 - Query Complexity 87 - Error Presenters and Recover Func 88 - Introspection Query support 89 - Query AST cache 90 - Tracing API 91 92 They can be `Use`'d like this: 93 94 ```go 95 srv := handler.New(es) 96 srv.Use(extension.Introspection{}) 97 srv.Use(extension.AutomaticPersistedQuery{ 98 Cache: lru.New(100), 99 }) 100 srv.Use(apollotracing.Tracer{}) 101 ``` 102 103 ### Default server 104 105 We provide a set of default extensions and transports if you aren't ready to customize them yet. Simply: 106 ```go 107 handler.NewDefaultServer(es) 108 ``` 109 110 ### More consistent naming 111 112 As part of cleaning up the names the RequestContext has been renamed to OperationContext, as there can be multiple created during the lifecycle of a request. A new ResponseContext has also been created and error handling has been moved here. This allows each response in a subscription to have its own errors. I'm not sure what bugs this might have been causing before... 113 114 ### Removal of tracing 115 116 Many of the old interfaces collapse down into just a few extension points: 117 118  119 120 The tracing interface has also been removed, tracing stats are now measured in core (eg time to parse query) and made available on the operation/response contexts. Much of the old interface was designed so that users of a tracer dont need to know which extension points it was listening to, the new handler extensions have the same goal. 121 122 ### Backward compatibility 123 124 There is a backwards compatibility layer that keeps most of the original interface in place. There are a few places where BC is known to be broken: 125 126 - ResponseMiddleware: The signature used to be `func(ctx context.Context, next func(ctx context.Context) []byte) []byte` and is now `func(ctx context.Context) *Response`. We could maintain BC by marshalling to json before and after, but the change is pretty easy to make and is likely to cause less issues. 127 - The Tracer interface has been removed, any tracers will need to be reimplemented against the new extension interface. 128 129 ## New resolver layout 130 131 0.11 also added a new way to generate and layout resolvers on disk. We used to only generate resolver implementations 132 whenever the file didnt exist. This behaviour is still there for those that are already used to it, However there is a 133 new mode you can turn on in config: 134 135 ```yaml 136 resolver: 137 layout: follow-schema 138 dir: graph 139 ``` 140 141 This tells gqlgen to generate resolvers next to the schema file that declared the graphql field, which looks like this: 142 143 