github.com/mhmtszr/concurrent-swiss-map@v1.0.8/README.md (about) 1 # Concurrent Swiss Map [![GoDoc][doc-img]][doc] [![Build Status][ci-img]][ci] [![Coverage Status][cov-img]][cov] [![Go Report Card][go-report-img]][go-report] 2 3 **Concurrent Swiss Map** is an open-source Go library that provides a high-performance, thread-safe generic concurrent hash map implementation designed to handle concurrent access efficiently. It's built with a focus on simplicity, speed, and reliability, making it a solid choice for scenarios where concurrent access to a hash map is crucial. 4 5 Uses [dolthub/swiss](https://github.com/dolthub/swiss) map implementation under the hood. 6 7 ## Installation 8 9 Supports 1.18+ Go versions because of Go Generics 10 11 ``` 12 go get github.com/mhmtszr/concurrent-swiss-map 13 ``` 14 15 ## Usage 16 17 New functions will be added soon... 18 19 ```go 20 package main 21 22 import ( 23 "hash/fnv" 24 25 csmap "github.com/mhmtszr/concurrent-swiss-map" 26 ) 27 28 func main() { 29 myMap := csmap.Create[string, int]( 30 // set the number of map shards. the default value is 32. 31 csmap.WithShardCount[string, int](32), 32 33 // if don't set custom hasher, use the built-in maphash. 34 csmap.WithCustomHasher[string, int](func(key string) uint64 { 35 hash := fnv.New64a() 36 hash.Write([]byte(key)) 37 return hash.Sum64() 38 }), 39 40 // set the total capacity, every shard map has total capacity/shard count capacity. the default value is 0. 41 csmap.WithSize[string, int](1000), 42 ) 43 44 key := "swiss-map" 45 myMap.Store(key, 10) 46 47 val, ok := myMap.Load(key) 48 println("load val:", val, "exists:", ok) 49 50 deleted := myMap.Delete(key) 51 println("deleted:", deleted) 52 53 ok = myMap.Has(key) 54 println("has:", ok) 55 56 empty := myMap.IsEmpty() 57 println("empty:", empty) 58 59 myMap.SetIfAbsent(key, 11) 60 61 myMap.Range(func(key string, value int) (stop bool) { 62 println("range:", key, value) 63 return true 64 }) 65 66 count := myMap.Count() 67 println("count:", count) 68 69 // Output: 70 // load val: 10 exists: true 71 // deleted: true 72 // has: false 73 // empty: true 74 // range: swiss-map 11 75 // count: 1 76 } 77 ``` 78 79 ## Basic Architecture 80 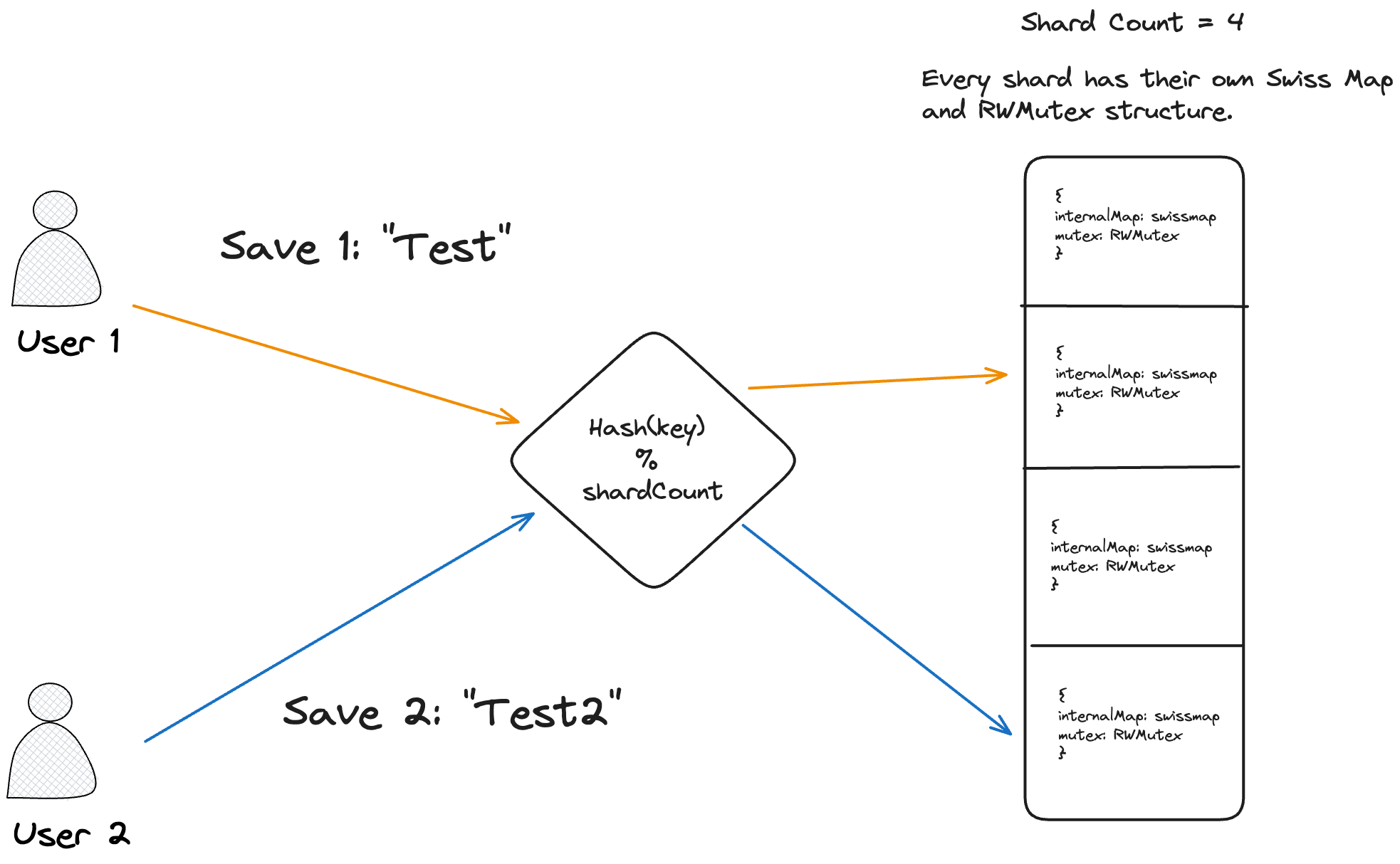 81 82 ## Benchmark Test 83 Benchmark was made on: 84 - Apple M1 Max 85 - 32 GB memory 86 87 Benchmark test results can be obtained by running [this file](concurrent_swiss_map_benchmark_test.go) on local computers. 88 89 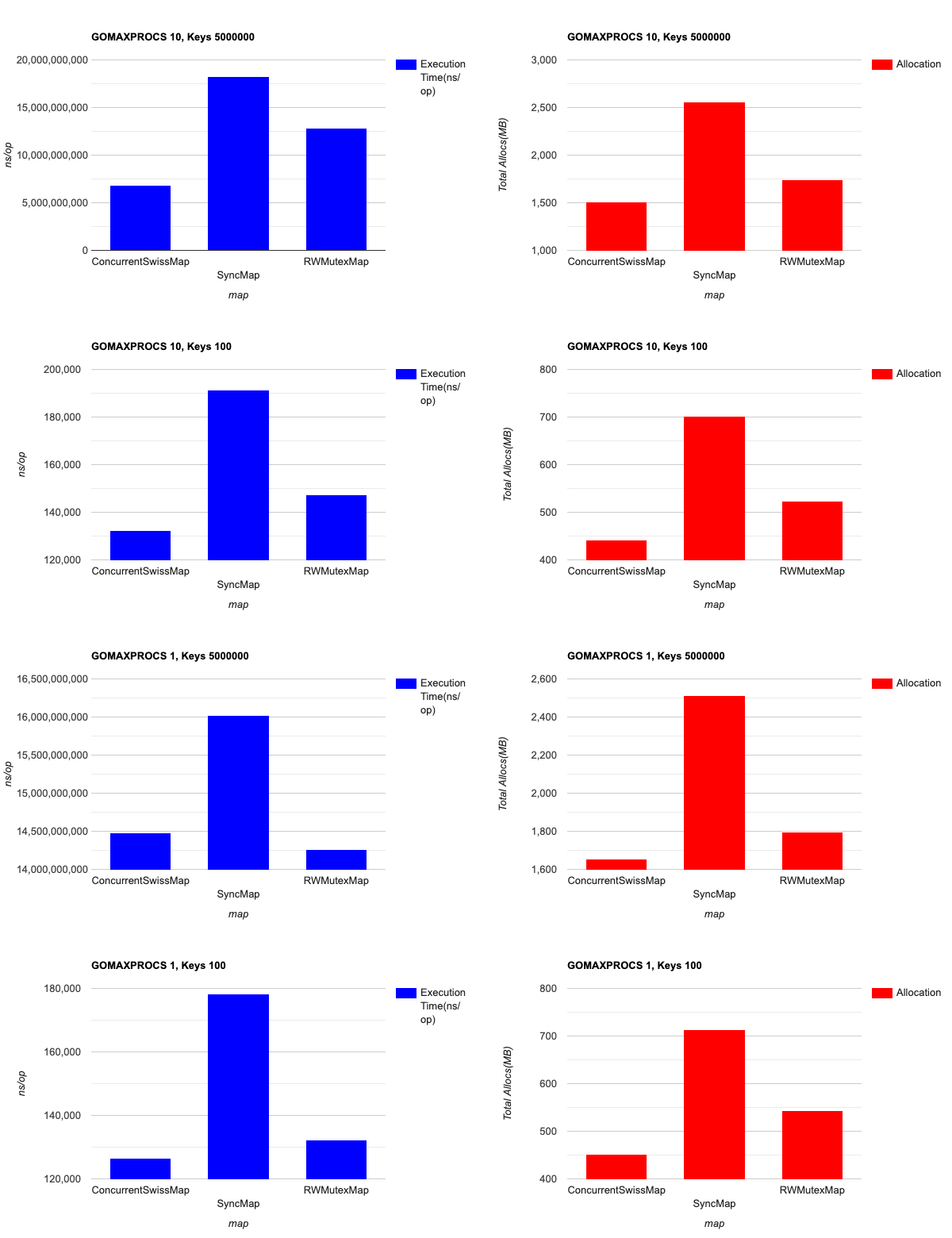 90 91 ### Benchmark Results 92 93 - Memory usage of the concurrent swiss map is better than other map implementations in all checked test scenarios. 94 - In high concurrent systems, the concurrent swiss map is faster, but in systems containing few concurrent operations, it works similarly to RWMutexMap. 95 96 [doc-img]: https://godoc.org/github.com/mhmtszr/concurrent-swiss-map?status.svg 97 [doc]: https://godoc.org/github.com/mhmtszr/concurrent-swiss-map 98 [ci-img]: https://github.com/mhmtszr/concurrent-swiss-map/actions/workflows/build-test.yml/badge.svg 99 [ci]: https://github.com/mhmtszr/concurrent-swiss-map/actions/workflows/build-test.yml 100 [cov-img]: https://codecov.io/gh/mhmtszr/concurrent-swiss-map/branch/master/graph/badge.svg 101 [cov]: https://codecov.io/gh/mhmtszr/concurrent-swiss-map 102 [go-report-img]: https://goreportcard.com/badge/github.com/mhmtszr/concurrent-swiss-map 103 [go-report]: https://goreportcard.com/report/github.com/mhmtszr/concurrent-swiss-map