github.com/mncinnovation/ponzu@v0.11.1-0.20200102001432-9bc41b703131/README.md (about) 1 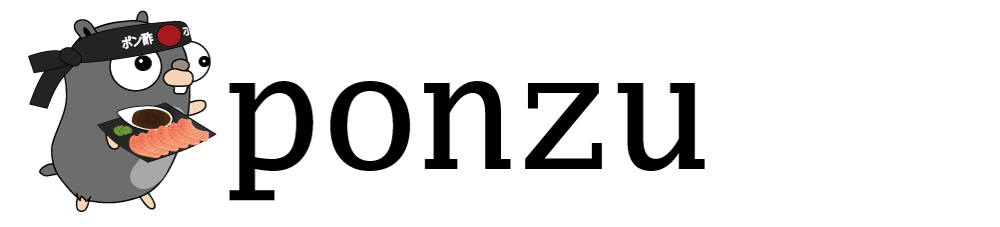 2 3 # Ponzu 4 [](https://github.com/ponzu-cms/ponzu/releases/latest) 5 [](https://godoc.org/github.com/ponzu-cms/ponzu) 6 [](https://circleci.com/gh/ponzu-cms/ponzu/tree/master) 7 8 > Watch the [**video introduction**](https://www.youtube.com/watch?v=T_1ncPoLgrg) 9 10 Ponzu is a powerful and efficient open-source HTTP server framework and CMS. It 11 provides automatic, free, and secure HTTP/2 over TLS (certificates obtained via 12 [Let's Encrypt](https://letsencrypt.org)), a useful CMS and scaffolding to 13 generate content editors, and a fast HTTP API on which to build modern applications. 14 15 Ponzu is released under the BSD-3-Clause license (see LICENSE). 16 (c) [Boss Sauce Creative, LLC](https://bosssauce.it) 17 18 ## Why? 19 With the rise in popularity of web/mobile apps connected to JSON HTTP APIs, better 20 tools to support the development of content servers and management systems are necessary. 21 Ponzu fills the void where you want to reach for Wordpress to get a great CMS, or Rails for 22 rapid development, but need a fast JSON response in a high-concurrency environment. 23 24 **Because you want to turn this:** 25 ```bash 26 $ ponzu gen content song title:"string" artist:"string" rating:"int" opinion:"string":richtext spotify_url:"string" 27 ``` 28 29 **Into this:** 30 31  32 33 34 **What's inside** 35 - Automatic & Free SSL/TLS<sup id="a1">[1](#f1)</sup> 36 - HTTP/2 and Server Push 37 - Rapid development with CLI-controlled code generators 38 - User-friendly, extensible CMS and administration dashboard 39 - Simple deployment - single binary + assets, embedded DB ([BoltDB](https://github.com/boltdb/bolt)) 40 - Fast, helpful framework while maintaining control 41 42 <sup id="f1">1</sup> *TLS*: 43 - Development: self-signed certificates auto-generated 44 - Production: auto-renewing certificates fetched from [Let's Encrypt](https://letsencrypt.org) 45 46 [↩](#a1) 47 48 ## Documentation 49 For more detailed documentation, check out the [docs](https://docs.ponzu-cms.org) 50 51 ## Installation 52 53 ``` 54 $ go get -u github.com/ponzu-cms/ponzu/... 55 ``` 56 57 ## Requirements 58 Go 1.8+ 59 60 Since HTTP/2 Server Push is used, Go 1.8+ is required. However, it is not 61 required of clients connecting to a Ponzu server to make HTTP/2 requests. 62 63 ## Usage 64 65 ```bash 66 $ ponzu command [flags] <params> 67 ``` 68 69 ## Commands 70 71 ### new 72 73 Creates a project directory of the name supplied as a parameter immediately 74 following the 'new' option in the $GOPATH/src directory. Note: 'new' depends on 75 the program 'git' and possibly a network connection. If there is no local 76 repository to clone from at the local machine's $GOPATH, 'new' will attempt to 77 clone the 'github.com/ponzu-cms/ponzu' package from over the network. 78 79 Example: 80 ```bash 81 $ ponzu new github.com/nilslice/proj 82 > New ponzu project created at $GOPATH/src/github.com/nilslice/proj 83 ``` 84 85 Errors will be reported, but successful commands return nothing. 86 87 --- 88 89 ### generate, gen, g 90 91 Generate boilerplate code for various Ponzu components, such as `content`. 92 93 Example: 94 ```bash 95 generator struct fields and built-in types... 96 | | 97 v v 98 $ ponzu gen content review title:"string" body:"string":richtext rating:"int" 99 ^ ^ 100 | | 101 struct type (optional) input view specifier 102 ``` 103 104 The command above will generate the file `content/review.go` with boilerplate 105 methods, as well as struct definition, and corresponding field tags like: 106 107 ```go 108 type Review struct { 109 Title string `json:"title"` 110 Body string `json:"body"` 111 Rating int `json:"rating"` 112 } 113 ``` 114 115 The generate command will intelligently parse more sophisticated field names 116 such as 'field_name' and convert it to 'FieldName' and vice versa, only where 117 appropriate as per common Go idioms. Errors will be reported, but successful 118 generate commands return nothing. 119 120 **Input View Specifiers** _(optional)_ 121 122 The CLI can optionally parse a third parameter on the fields provided to generate 123 the type of HTML view an editor field is presented within. If no third parameter 124 is added, a plain text HTML input will be generated. In the example above, the 125 argument shown as `body:string:richtext` would show the Richtext input instead 126 of a plain text HTML input (as shown in the screenshot). The following input 127 view specifiers are implemented: 128 129 | CLI parameter | Generates | 130 |---------------|-----------| 131 | checkbox | `editor.Checkbox()` | 132 | custom | generates a pre-styled empty div to fill with HTML | 133 | file | `editor.File()` | 134 | hidden | `editor.Input()` + uses type=hidden | 135 | input, text | `editor.Input()` | 136 | richtext | `editor.Richtext()` | 137 | select | `editor.Select()` | 138 | textarea | `editor.Textarea()` | 139 | tags | `editor.Tags()` | 140 141 --- 142 143 ### build 144 145 From within your Ponzu project directory, running build will copy and move 146 the necessary files from your workspace into the vendored directory, and 147 will build/compile the project to then be run. 148 149 Optional flags: 150 - `--gocmd` sets the binary used when executing `go build` within `ponzu` build step 151 152 Example: 153 ```bash 154 $ ponzu build 155 (or) 156 $ ponzu build --gocmd=go1.8rc1 # useful for testing 157 ``` 158 159 Errors will be reported, but successful build commands return nothing. 160 161 --- 162 163 ### run 164 165 Starts the HTTP server for the JSON API, Admin System, or both. 166 The segments, separated by a comma, describe which services to start, either 167 'admin' (Admin System / CMS backend) or 'api' (JSON API), and, optionally, 168 if the server should utilize TLS encryption - served over HTTPS, which is 169 automatically managed using Let's Encrypt (https://letsencrypt.org) 170 171 Optional flags: 172 - `--port` sets the port on which the server listens for HTTP requests [defaults to 8080] 173 - `--https-port` sets the port on which the server listens for HTTPS requests [defaults to 443] 174 - `--https` enables auto HTTPS management via Let's Encrypt (port is always 443) 175 - `--dev-https` generates self-signed SSL certificates for development-only (port is 10443) 176 177 Example: 178 ```bash 179 $ ponzu run 180 (or) 181 $ ponzu run --port=8080 --https admin,api 182 (or) 183 $ ponzu run admin 184 (or) 185 $ ponzu run --port=8888 api 186 (or) 187 $ ponzu run --dev-https 188 ``` 189 Defaults to `$ ponzu run --port=8080 admin,api` (running Admin & API on port 8080, without TLS) 190 191 *Note:* 192 Admin and API cannot run on separate processes unless you use a copy of the 193 database, since the first process to open it receives a lock. If you intend 194 to run the Admin and API on separate processes, you must call them with the 195 'ponzu' command independently. 196 197 --- 198 199 ### upgrade 200 201 Will backup your own custom project code (like content, add-ons, uploads, etc) so 202 we can safely re-clone Ponzu from the latest version you have or from the network 203 if necessary. Before running `$ ponzu upgrade`, you should update the `ponzu` 204 package by running `$ go get -u github.com/ponzu-cms/ponzu/...` 205 206 Example: 207 ```bash 208 $ ponzu upgrade 209 ``` 210 211 --- 212 213 ### add, a 214 215 Downloads an add-on to GOPATH/src and copies it to the Ponzu project's ./addons directory. 216 Must be called from within a Ponzu project directory. 217 218 Example: 219 ```bash 220 $ ponzu add github.com/bosssauce/fbscheduler 221 ``` 222 223 Errors will be reported, but successful add commands return nothing. 224 225 --- 226 227 ### version, v 228 229 Prints the version of Ponzu your project is using. Must be called from within a 230 Ponzu project directory. By passing the `--cli` flag, the `version` command will 231 print the version of the Ponzu CLI you have installed. 232 233 Example: 234 ```bash 235 $ ponzu version 236 > Ponzu v0.8.2 237 (or) 238 $ ponzu version --cli 239 > Ponzu v0.9.2 240 ``` 241 242 --- 243 244 ## Contributing 245 246 1. Checkout branch ponzu-dev 247 2. Make code changes 248 3. Test changes to ponzu-dev branch 249 - make a commit to ponzu-dev 250 - to manually test, you will need to use a new copy (ponzu new path/to/code), but pass the --dev flag so that ponzu generates a new copy from the ponzu-dev branch, not master by default (i.e. `$ponzu new --dev /path/to/code`) 251 - build and run with $ ponzu build and $ ponzu run 252 4. To add back to master: 253 - first push to origin ponzu-dev 254 - create a pull request 255 - will then be merged into master 256 257 _A typical contribution workflow might look like:_ 258 ```bash 259 # clone the repository and checkout ponzu-dev 260 $ git clone https://github.com/ponzu-cms/ponzu path/to/local/ponzu # (or your fork) 261 $ git checkout ponzu-dev 262 263 # install ponzu with go get or from your own local path 264 $ go get github.com/ponzu-cms/ponzu/... 265 # or 266 $ cd /path/to/local/ponzu 267 $ go install ./... 268 269 # edit files, add features, etc 270 $ git add -A 271 $ git commit -m 'edited files, added features, etc' 272 273 # now you need to test the feature.. make a new ponzu project, but pass --dev flag 274 $ ponzu new --dev /path/to/new/project # will create $GOPATH/src/path/to/new/project 275 276 # build & run ponzu from the new project directory 277 $ cd /path/to/new/project 278 $ ponzu build && ponzu run 279 280 # push to your origin:ponzu-dev branch and create a PR at ponzu-cms/ponzu 281 $ git push origin ponzu-dev 282 # ... go to https://github.com/ponzu-cms/ponzu and create a PR 283 ``` 284 285 **Note:** if you intend to work on your own fork and contribute from it, you will 286 need to also pass `--fork=path/to/your/fork` (using OS-standard filepath structure), 287 where `path/to/your/fork` _must_ be within `$GOPATH/src`, and you are working from a branch 288 called `ponzu-dev`. 289 290 For example: 291 ```bash 292 # ($GOPATH/src is implied in the fork path, do not add it yourself) 293 $ ponzu new --dev --fork=github.com/nilslice/ponzu /path/to/new/project 294 ``` 295 296 297 ## Credits 298 - [golang.org/x/text/unicode/norm](https://golang.org/x/text/unicode/norm) 299 - [golang.org/x/text/transform](https://golang.org/x/text/transform) 300 - [golang.org/x/crypto/bcrypt](https://golang.org/x/crypto/bcrypt) 301 - [golang.org/x/net/http2](https://golang.org/x/net/http2) 302 - [github.com/blevesearch/bleve](https://github.com/blevesearch/bleve) 303 - [github.com/nilslice/jwt](https://github.com/nilslice/jwt) 304 - [github.com/nilslice/email](https://github.com/nilslice/email) 305 - [github.com/gorilla/schema](https://github.com/gorilla/schema) 306 - [github.com/gofrs/uuid](https://github.com/gofrs/uuid) 307 - [github.com/tidwall/gjson](https://github.com/tidwall/gjson) 308 - [github.com/tidwall/sjson](https://github.com/tidwall/sjson) 309 - [github.com/boltdb/bolt](https://github.com/boltdb/bolt) 310 - [github.com/spf13/cobra](github.com/spf13/cobra) 311 - [Materialnote Editor](https://github.com/Cerealkillerway/materialNote) 312 - [Materialize.css](https://github.com/Dogfalo/materialize) 313 - [jQuery](https://github.com/jquery/jquery) 314 - [Chart.js](https://github.com/chartjs/Chart.js) 315 316 317 ### Logo 318 The Go gopher was designed by Renee French. (http://reneefrench.blogspot.com) 319 The design is licensed under the Creative Commons 3.0 Attribution license. 320 Read this article for more details: http://blog.golang.org/gopher 321 322 The Go gopher vector illustration by Hugo Arganda [@argandas](https://twitter.com/argandas) (http://about.me/argandas) 323 324 "Gotoro", the sushi chef, is a modification of Hugo Arganda's illustration by Steve Manuel (https://github.com/nilslice).