github.com/moitias/moq@v0.0.0-20240223074357-5eb0f0ba4054/README.md (about) 1 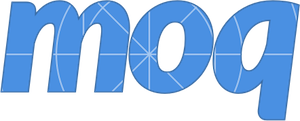 [](https://github.com/matryer/moq/actions?query=branch%3Amaster) [](https://goreportcard.com/report/github.com/matryer/moq) 2 3 Interface mocking tool for go generate. 4 5 ### What is Moq? 6 7 Moq is a tool that generates a struct from any interface. The struct can be used in test code as a mock of the interface. 8 9 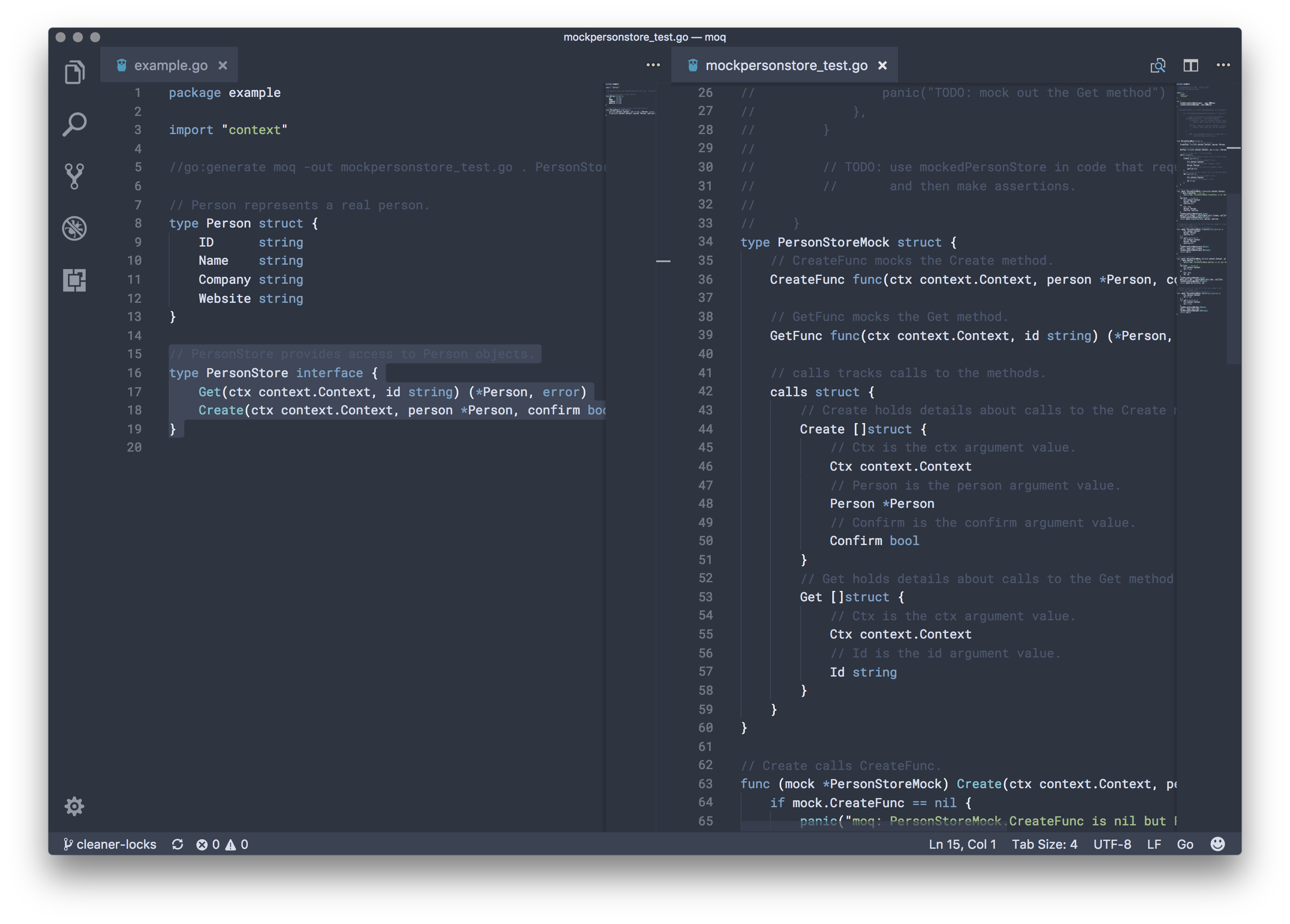 10 11 above: Moq generates the code on the right. 12 13 You can read more in the [Meet Moq blog post](http://bit.ly/meetmoq). 14 15 ### Installing 16 17 To start using latest released version of Moq, just run: 18 19 ``` 20 $ go install github.com/matryer/moq@latest 21 ``` 22 23 Note that Go 1.18+ is needed for installing from source. For using Moq with 24 older Go versions, use the pre-built binaries published with 25 [Moq releases](https://github.com/matryer/moq/releases). 26 27 ### Usage 28 29 ``` 30 moq [flags] source-dir interface [interface2 [interface3 [...]]] 31 -fmt string 32 go pretty-printer: gofmt, goimports or noop (default gofmt) 33 -out string 34 output file (default stdout) 35 -pkg string 36 package name (default will infer) 37 -rm 38 first remove output file, if it exists 39 -skip-ensure 40 suppress mock implementation check, avoid import cycle if mocks generated outside of the tested package 41 -stub 42 return zero values when no mock implementation is provided, do not panic 43 -version 44 show the version for moq 45 -with-resets 46 generate functions to facilitate resetting calls made to a mock 47 48 Specifying an alias for the mock is also supported with the format 'interface:alias' 49 50 Ex: moq -pkg different . MyInterface:MyMock 51 ``` 52 53 **NOTE:** `source-dir` is the directory where the source code (definition) of the target interface is located. 54 It needs to be a path to a directory and not the import statement for a Go package. 55 56 In a command line: 57 58 ``` 59 $ moq -out mocks_test.go . MyInterface 60 ``` 61 62 In code (for go generate): 63 64 ```go 65 package my 66 67 //go:generate moq -out myinterface_moq_test.go . MyInterface 68 69 type MyInterface interface { 70 Method1() error 71 Method2(i int) 72 } 73 ``` 74 75 Then run `go generate` for your package. 76 77 ### How to use it 78 79 Mocking interfaces is a nice way to write unit tests where you can easily control the behaviour of the mocked object. 80 81 Moq creates a struct that has a function field for each method, which you can declare in your test code. 82 83 In this example, Moq generated the `EmailSenderMock` type: 84 85 ```go 86 func TestCompleteSignup(t *testing.T) { 87 88 var sentTo string 89 90 mockedEmailSender = &EmailSenderMock{ 91 SendFunc: func(to, subject, body string) error { 92 sentTo = to 93 return nil 94 }, 95 } 96 97 CompleteSignUp("me@email.com", mockedEmailSender) 98 99 callsToSend := len(mockedEmailSender.SendCalls()) 100 if callsToSend != 1 { 101 t.Errorf("Send was called %d times", callsToSend) 102 } 103 if sentTo != "me@email.com" { 104 t.Errorf("unexpected recipient: %s", sentTo) 105 } 106 107 } 108 109 func CompleteSignUp(to string, sender EmailSender) { 110 // TODO: this 111 } 112 ``` 113 114 The mocked structure implements the interface, where each method calls the associated function field. 115 116 ## Tips 117 118 * Keep mocked logic inside the test that is using it 119 * Only mock the fields you need 120 * It will panic if a nil function gets called 121 * Name arguments in the interface for a better experience 122 * Use closured variables inside your test function to capture details about the calls to the methods 123 * Use `.MethodCalls()` to track the calls 124 * Use `.ResetCalls()` to reset calls within an invidual mock's context 125 * Use `go:generate` to invoke the `moq` command 126 * If Moq fails with a `go/format` error, it indicates the generated code was not valid. 127 You can run the same command with `-fmt noop` to print the generated source code without attempting to format it. 128 This can aid in debugging the root cause. 129 130 ## License 131 132 The Moq project (and all code) is licensed under the [MIT License](LICENSE). 133 134 Moq was created by [Mat Ryer](https://twitter.com/matryer) and [David Hernandez](https://github.com/dahernan), with ideas lovingly stolen from [Ernesto Jimenez](https://github.com/ernesto-jimenez). Featuring a major refactor by @sudo-suhas, as well as lots of other contributors. 135 136 The Moq logo was created by [Chris Ryer](http://chrisryer.co.uk) and is licensed under the [Creative Commons Attribution 3.0 License](https://creativecommons.org/licenses/by/3.0/). 137