github.com/mstephano/gqlgen-schemagen@v0.0.0-20230113041936-dd2cd4ea46aa/README.md (about) 1 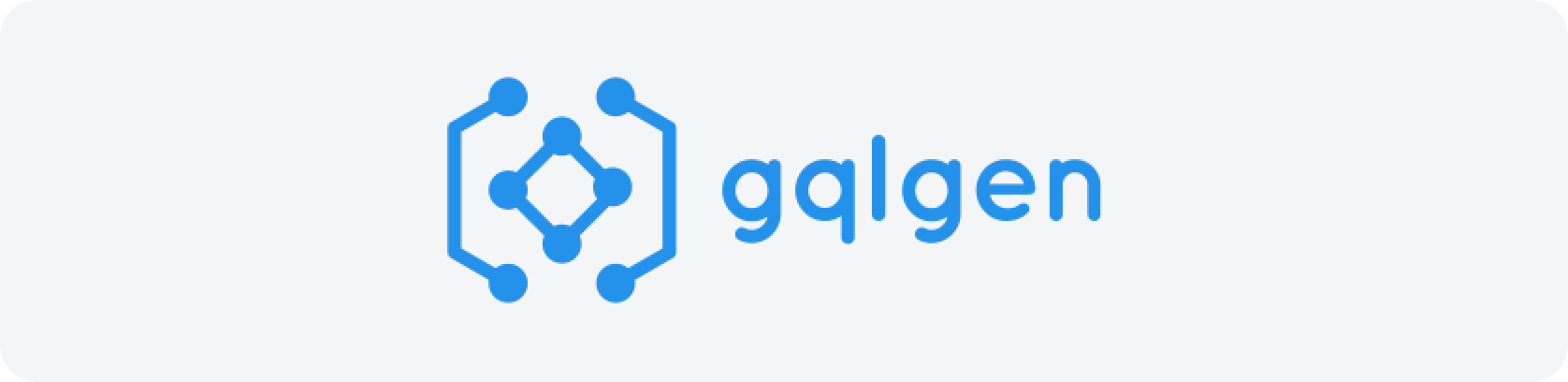 2 3 # gqlgen [](https://github.com/mstephano/gqlgen-schemagen/actions) [](https://coveralls.io/github/mstephano/gqlgen-schemagen?branch=master) [](https://goreportcard.com/report/github.com/mstephano/gqlgen-schemagen) [](https://pkg.go.dev/github.com/mstephano/gqlgen-schemagen) [](http://gqlgen.com/) 4 5 ## What is gqlgen? 6 7 [gqlgen](https://github.com/mstephano/gqlgen-schemagen) is a Go library for building GraphQL servers without any fuss.<br/> 8 9 - **gqlgen is based on a Schema first approach** — You get to Define your API using the GraphQL [Schema Definition Language](http://graphql.org/learn/schema/). 10 - **gqlgen prioritizes Type safety** — You should never see `map[string]interface{}` here. 11 - **gqlgen enables Codegen** — We generate the boring bits, so you can focus on building your app quickly. 12 13 Still not convinced enough to use **gqlgen**? Compare **gqlgen** with other Go graphql [implementations](https://gqlgen.com/feature-comparison/) 14 15 ## Quick start 16 17 1. [Initialise a new go module](https://golang.org/doc/tutorial/create-module) 18 19 ```console 20 mkdir example 21 cd example 22 go mod init example 23 ``` 24 25 2. Initialise gqlgen config and generate models 26 27 ```console 28 go get github.com/mstephano/gqlgen-schemagen 29 go run github.com/mstephano/gqlgen-schemagen init 30 go mod tidy 31 ``` 32 33 3. Start the graphql server 34 35 ```console 36 go run server.go 37 ``` 38 39 More help to get started: 40 41 - [Getting started tutorial](https://gqlgen.com/getting-started/) - a comprehensive guide to help you get started 42 - [Real-world examples](https://github.com/mstephano/gqlgen-schemagen/tree/master/_examples) show how to create GraphQL applications 43 - [Reference docs](https://pkg.go.dev/github.com/mstephano/gqlgen-schemagen) for the APIs 44 45 ## Reporting Issues 46 47 If you think you've found a bug, or something isn't behaving the way you think it should, please raise an [issue](https://github.com/mstephano/gqlgen-schemagen/issues) on GitHub. 48 49 ## Contributing 50 51 We welcome contributions, Read our [Contribution Guidelines](https://github.com/mstephano/gqlgen-schemagen/blob/master/CONTRIBUTING.md) to learn more about contributing to **gqlgen** 52 53 ## Frequently asked questions 54 55 ### How do I prevent fetching child objects that might not be used? 56 57 When you have nested or recursive schema like this: 58 59 ```graphql 60 type User { 61 id: ID! 62 name: String! 63 friends: [User!]! 64 } 65 ``` 66 67 You need to tell gqlgen that it should only fetch friends if the user requested it. There are two ways to do this; 68 69 - #### Using Custom Models 70 71 Write a custom model that omits the friends field: 72 73 ```go 74 type User struct { 75 ID int 76 Name string 77 } 78 ``` 79 80 And reference the model in `gqlgen.yml`: 81 82 ```yaml 83 # gqlgen.yml 84 models: 85 User: 86 model: github.com/you/pkg/model.User # go import path to the User struct above 87 ``` 88 89 - #### Using Explicit Resolvers 90 91 If you want to Keep using the generated model, mark the field as requiring a resolver explicitly in `gqlgen.yml` like this: 92 93 ```yaml 94 # gqlgen.yml 95 models: 96 User: 97 fields: 98 friends: 99 resolver: true # force a resolver to be generated 100 ``` 101 102 After doing either of the above and running generate we will need to provide a resolver for friends: 103 104 ```go 105 func (r *userResolver) Friends(ctx context.Context, obj *User) ([]*User, error) { 106 // select * from user where friendid = obj.ID 107 return friends, nil 108 } 109 ``` 110 111 You can also use inline config with directives to achieve the same result 112 113 ```graphql 114 directive @goModel( 115 model: String 116 models: [String!] 117 ) on OBJECT | INPUT_OBJECT | SCALAR | ENUM | INTERFACE | UNION 118 119 directive @goField( 120 forceResolver: Boolean 121 name: String 122 ) on INPUT_FIELD_DEFINITION | FIELD_DEFINITION 123 124 type User @goModel(model: "github.com/you/pkg/model.User") { 125 id: ID! @goField(name: "todoId") 126 friends: [User!]! @goField(forceResolver: true) 127 } 128 ``` 129 130 ### Can I change the type of the ID from type String to Type Int? 131 132 Yes! You can by remapping it in config as seen below: 133 134 ```yaml 135 models: 136 ID: # The GraphQL type ID is backed by 137 model: 138 - github.com/mstephano/gqlgen-schemagen/graphql.IntID # a go integer 139 - github.com/mstephano/gqlgen-schemagen/graphql.ID # or a go string 140 ``` 141 142 This means gqlgen will be able to automatically bind to strings or ints for models you have written yourself, but the 143 first model in this list is used as the default type and it will always be used when: 144 145 - Generating models based on schema 146 - As arguments in resolvers 147 148 There isn't any way around this, gqlgen has no way to know what you want in a given context. 149 150 ### Why do my interfaces have getters? Can I disable these? 151 152 These were added in v0.17.14 to allow accessing common interface fields without casting to a concrete type. 153 However, certain fields, like Relay-style Connections, cannot be implemented with simple getters. 154 155 If you'd prefer to not have getters generated in your interfaces, you can add the following in your `gqlgen.yml`: 156 157 ```yaml 158 # gqlgen.yml 159 omit_getters: true 160 ``` 161 162 ## Other Resources 163 164 - [Christopher Biscardi @ Gophercon UK 2018](https://youtu.be/FdURVezcdcw) 165 - [Introducing gqlgen: a GraphQL Server Generator for Go](https://99designs.com.au/blog/engineering/gqlgen-a-graphql-server-generator-for-go/) 166 - [Dive into GraphQL by Iván Corrales Solera](https://medium.com/@ivan.corrales.solera/dive-into-graphql-9bfedf22e1a) 167 - [Sample Project built on gqlgen with Postgres by Oleg Shalygin](https://github.com/oshalygin/gqlgen-pg-todo-example) 168 - [Hackernews GraphQL Server with gqlgen by Shayegan Hooshyari](https://www.howtographql.com/graphql-go/0-introduction/)