github.com/nibnait/go-learn@v0.0.0-20220227013611-dfa47ea6d2da/chapter/ch4_并发编程.md (about) 1 # ch4 并发编程 2 3 ## Thead vs. Groutine 4 5 1. 创建时默认的 stack 的⼤⼩ 6 - JDK5 以后 Java Thread stack 默认为1M 7 - Groutine 的 Stack 初始化⼤⼩为2K 8 9 10 2. 和 KSE (Kernel Space Entity) 的对应关系 11 - Java Thread 是 1:1 12 - Groutine 是 M:N 13 14 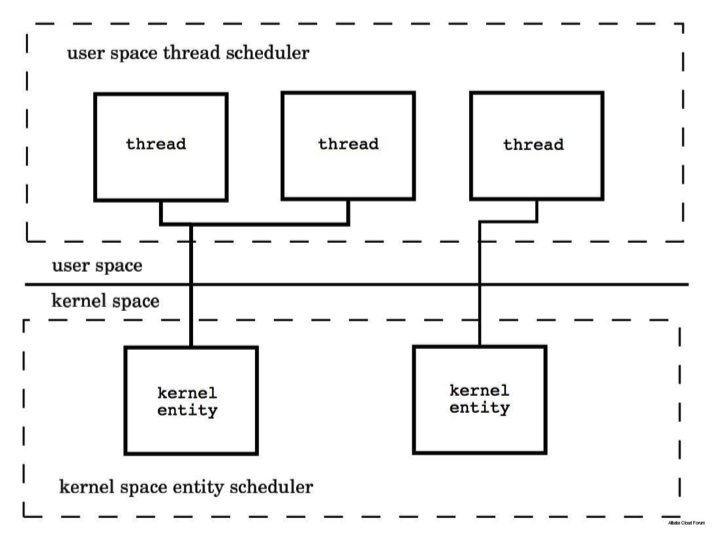 15 16 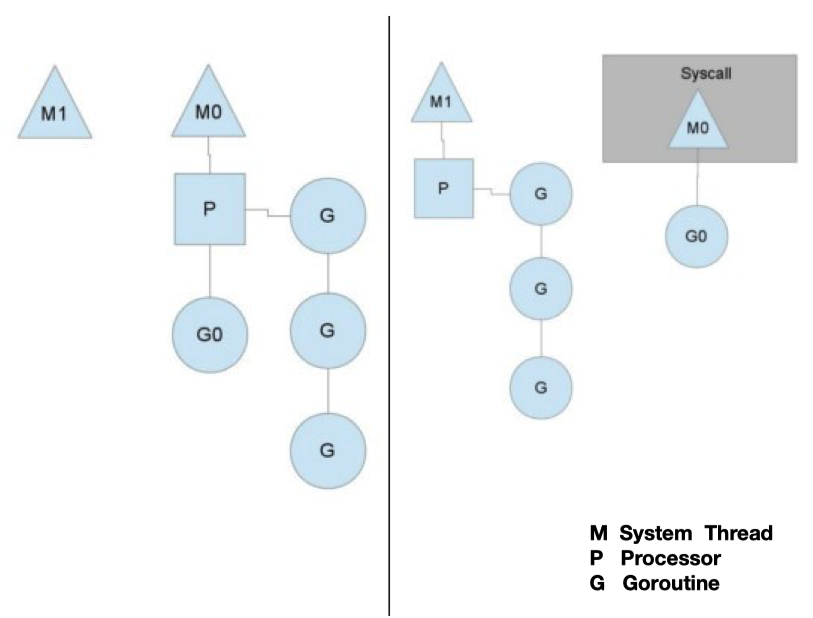 17 18 计数器:procesor 完成的 协程的数量。。长时间不变,直接中断 19 20 ## 协程 21 22 ```go 23 func TestGroutine(t *testing.T) { 24 for i := 0; i < 10; i++ { 25 go func(i int) { 26 fmt.Println(i) 27 }(i) 28 } 29 time.Sleep(time.Millisecond * 50) 30 } 31 ``` 32 33 ## CSP(Communicating sequential processes) 并发机制 34 35 类似于 Java 中的 Future 36 37 [26_async_service_test.go](../src/test/chapter/ch4/26_async_service_test.go) 38 39 ## 多路选择 和 超时控制 40 41 [27_select_test.go](../src/test/chapter/ch4/27_select_test.go) 42 43 ## Channel 的关闭和广播 44 45 [28_select_close_test](../src/test/chapter/ch4/28_select_close_test.go) 46 47 - 向关闭的 channel 发送数据,会导致 panic 48 - v, ok <-ch; ok 为 bool 值,true 表示正常接受,false 表示通道关闭 49 - 所有的 channel 接收者都会在 channel 关闭时,⽴刻从阻塞等待中返回且上 述 ok 值为 false。这个⼴播机制常被利⽤,进⾏向多个订阅者同时发送信号。 如:退出信号。 50 51 ## Context 与 任务取消 52 53 [29_cancel_test](../src/test/chapter/ch4/29_cancel_test.go) 54 55 [30_cancel_by_context_test.go](../src/test/chapter/ch4/30_cancel_by_context_test.go) 56 57 Context 58 59 - 根 Context:通过 context.Background () 创建 60 - ⼦ Context:context.WithCancel(parentContext) 创建 61 - ctx, cancel := context.WithCancel(context.Background()) 62 - 当前 Context 被取消时,基于他的⼦ context 都会被取消 63 - 接收取消通知 <- ctx.Done() 64 65 ## 常见并发任务 66 67 ### 仅执行一次 68 69 单例模式(懒汉式、线程安全) 70 71 [31_once_test.go](../src/test/chapter/ch4/31_once_test.go) 72 73 ```go 74 var singleInstance *Singleton 75 var once sync.Once 76 77 func GetSingletonObj() *Singleton { 78 once.Do(func() { 79 fmt.Println("create obj") 80 singleInstance = new(Singleton) 81 }) 82 return singleInstance 83 } 84 ``` 85 86 ### 仅需任意任务完成 87 88 [32_first_response_test](src/test/chapter/ch4/32_first_response_test) 89 90 ### 必须所有任务完成 91 92 [33_util_all_done_test](src/test/chapter/ch4/33_util_all_done_test) 93 94 ## 对象池 95 96 ### 使⽤buffered channel实现对象池 97 98 [34_obj_pool_test.go](src/test/chapter/ch4/34_obj_pool_test.go) 99 100 ### sync.Pool 101 102 - 适合于通过复⽤,降低复杂对象的创建和 GC 代价 103 - 协程安全,会有锁的开销 104 - ⽣命周期受 GC 影响,不适合于做连接池等,需⾃⼰管理⽣命周期的资源的池化