github.com/oam-dev/kubevela@v1.9.11/docs/examples/multicluster/deploy.md (about) 1 # Advanced examples for multi-cluster deployment 2 3 The below features are introduced in KubeVela v1.3. 4 5 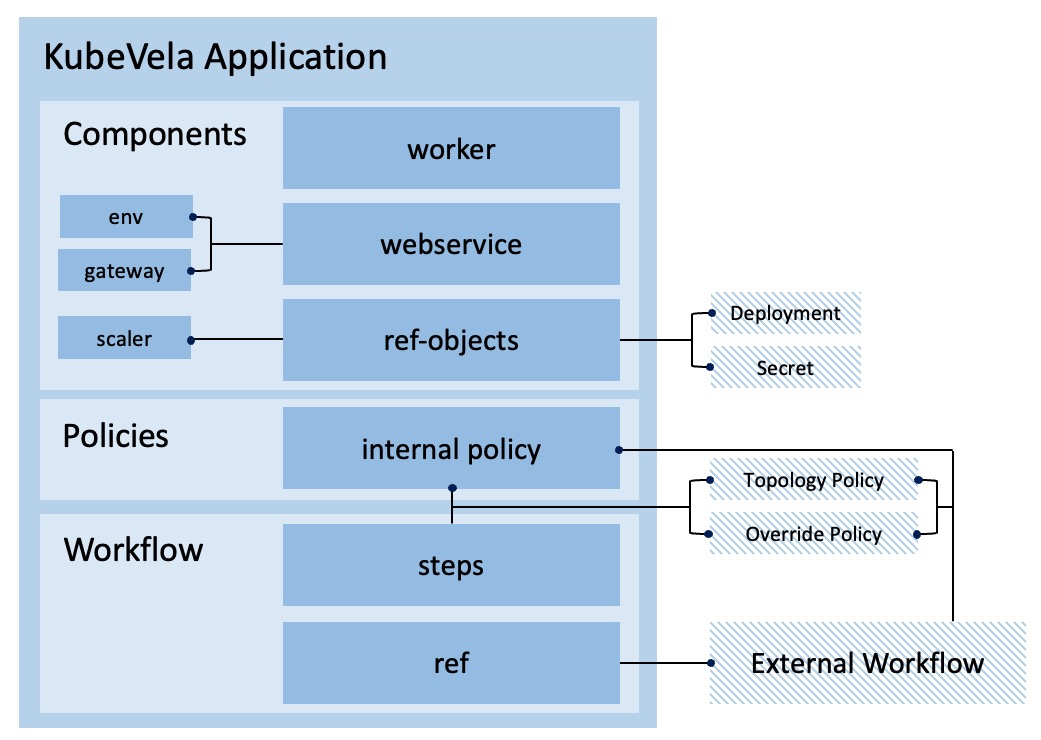 6 7 ## Topology Policy 8 9 Topology policy is a policy used to describe the location where application component should be deployed and managed. 10 11 The most straight forward way is directly specifying the names of clusters to be deployed. 12 In the following example, the nginx webservice will be deployed to the `examples` namespace in both `hangzhou-1` and `hangzhou-2` clusters concurrently. 13 After nginx in both clusters are ready, the application will finish running workflow and becomes healthy. 14 15 ```yaml 16 apiVersion: core.oam.dev/v1beta1 17 kind: Application 18 metadata: 19 name: basic-topology 20 namespace: examples 21 spec: 22 components: 23 - name: nginx-basic 24 type: webservice 25 properties: 26 image: nginx 27 policies: 28 - name: topology-hangzhou-clusters 29 type: topology 30 properties: 31 clusters: ["hangzhou-1", "hangzhou-2"] 32 ``` 33 34 The clusters in the topology can also be selected by labels instead of names. 35 36 ```yaml 37 apiVersion: core.oam.dev/v1beta1 38 kind: Application 39 metadata: 40 name: label-selector-topology 41 namespace: examples 42 spec: 43 components: 44 - name: nginx-label-selector 45 type: webservice 46 properties: 47 image: nginx 48 policies: 49 - name: topology-hangzhou-clusters 50 type: topology 51 properties: 52 clusterLabelSelector: 53 region: hangzhou 54 ``` 55 56 If you want to deploy application components into the control plane cluster, you can use the `local` cluster. 57 Besides, you can also deploy your application components in another namespace other than the application's namespace. 58 59 ```yaml 60 apiVersion: core.oam.dev/v1beta1 61 kind: Application 62 metadata: 63 name: local-ns-topology 64 namespace: examples 65 spec: 66 components: 67 - name: nginx-local-ns 68 type: webservice 69 properties: 70 image: nginx 71 policies: 72 - name: topology-local 73 type: topology 74 properties: 75 clusters: ["local"] 76 namespace: examples-alternative 77 ``` 78 79 ## Deploy WorkflowStep 80 81 By default, if you declare multiple topology policies in the application, the application components will be deployed in all destinations following the order of the policies. 82 83 If you want to manipulate the process of deploying them, for example, changing the order or adding manual-approval, you can use the `deploy` workflow step explicitly in the workflow to achieve that. 84 85 ```yaml 86 apiVersion: core.oam.dev/v1beta1 87 kind: Application 88 metadata: 89 name: deploy-workflowstep 90 namespace: examples 91 spec: 92 components: 93 - name: nginx-deploy-workflowstep 94 type: webservice 95 properties: 96 image: nginx 97 policies: 98 - name: topology-hangzhou-clusters 99 type: topology 100 properties: 101 clusterLabelSelector: 102 region: hangzhou 103 - name: topology-local 104 type: topology 105 properties: 106 clusters: ["local"] 107 namespace: examples-alternative 108 workflow: 109 steps: 110 - type: deploy 111 name: deploy-local 112 properties: 113 policies: ["topology-local"] 114 - type: deploy 115 name: deploy-hangzhou 116 properties: 117 # require manual approval before running this step 118 auto: false 119 policies: ["topology-hangzhou-clusters"] 120 ``` 121 122 You can also deploy application components with different topology policies concurrently, by filling these topology policies in on `deploy` step. 123 124 ```yaml 125 apiVersion: core.oam.dev/v1beta1 126 kind: Application 127 metadata: 128 name: deploy-concurrently 129 namespace: examples 130 spec: 131 components: 132 - name: nginx-deploy-concurrently 133 type: webservice 134 properties: 135 image: nginx 136 policies: 137 - name: topology-hangzhou-clusters 138 type: topology 139 properties: 140 clusterLabelSelector: 141 region: hangzhou 142 - name: topology-local 143 type: topology 144 properties: 145 clusters: ["local"] 146 namespace: examples-alternative 147 workflow: 148 steps: 149 - type: deploy 150 name: deploy-all 151 properties: 152 policies: ["topology-local", "topology-hangzhou-clusters"] 153 ``` 154 155 ## Override Policy 156 157 Override policy helps you to customize the application components in different clusters. For example, using a different container image or changing the default number of replicas. The override policy should be used together with the topology policy in the `deploy` workflow step. 158 159 ```yaml 160 apiVersion: core.oam.dev/v1beta1 161 kind: Application 162 metadata: 163 name: deploy-with-override 164 namespace: examples 165 spec: 166 components: 167 - name: nginx-with-override 168 type: webservice 169 properties: 170 image: nginx 171 policies: 172 - name: topology-hangzhou-clusters 173 type: topology 174 properties: 175 clusterLabelSelector: 176 region: hangzhou 177 - name: topology-local 178 type: topology 179 properties: 180 clusters: ["local"] 181 namespace: examples-alternative 182 - name: override-nginx-legacy-image 183 type: override 184 properties: 185 components: 186 - name: nginx-with-override 187 properties: 188 image: nginx:1.20 189 - name: override-high-availability 190 type: override 191 properties: 192 components: 193 - type: webservice 194 traits: 195 - type: scaler 196 properties: 197 replicas: 3 198 workflow: 199 steps: 200 - type: deploy 201 name: deploy-local 202 properties: 203 policies: ["topology-local"] 204 - type: deploy 205 name: deploy-hangzhou 206 properties: 207 policies: ["topology-hangzhou-clusters", "override-nginx-legacy-image", "override-high-availability"] 208 ``` 209 210 The override policy has many advanced capabilities, such as adding new component or selecting components to use. 211 The following example will deploy `nginx:1.20` to local cluster. `nginx` and `nginx:stable` will be deployed to hangzhou clusters. 212 213 ```yaml 214 apiVersion: core.oam.dev/v1beta1 215 kind: Application 216 metadata: 217 name: advance-override 218 namespace: examples 219 spec: 220 components: 221 - name: nginx-advance-override-legacy 222 type: webservice 223 properties: 224 image: nginx:1.20 225 - name: nginx-advance-override-latest 226 type: webservice 227 properties: 228 image: nginx 229 policies: 230 - name: topology-hangzhou-clusters 231 type: topology 232 properties: 233 clusterLabelSelector: 234 region: hangzhou 235 - name: topology-local 236 type: topology 237 properties: 238 clusters: ["local"] 239 namespace: examples-alternative 240 - name: override-nginx-legacy 241 type: override 242 properties: 243 selector: ["nginx-advance-override-legacy"] 244 - name: override-nginx-latest 245 type: override 246 properties: 247 selector: ["nginx-advance-override-latest", "nginx-advance-override-stable"] 248 components: 249 - name: nginx-advance-override-stable 250 type: webservice 251 properties: 252 image: nginx:stable 253 workflow: 254 steps: 255 - type: deploy 256 name: deploy-local 257 properties: 258 policies: ["topology-local", "override-nginx-legacy"] 259 - type: deploy 260 name: deploy-hangzhou 261 properties: 262 policies: ["topology-hangzhou-clusters", "override-nginx-latest"] 263 ``` 264 265 ## Ref-object Component 266 267 Sometimes, you may want to copy resources from one place to other places, such as copying secrets from the control plane cluster into managed clusters. 268 You can use the `ref-object` typed component to achieve that. 269 270 ```yaml 271 apiVersion: core.oam.dev/v1beta1 272 kind: Application 273 metadata: 274 name: ref-objects-example 275 namespace: examples 276 spec: 277 components: 278 - name: image-pull-secrets 279 type: ref-objects 280 properties: 281 objects: 282 - resource: secret 283 name: image-credential-to-copy 284 policies: 285 - name: topology-hangzhou-clusters 286 type: topology 287 properties: 288 clusterLabelSelector: 289 region: hangzhou 290 ``` 291 292 You can also select resources by labels and duplicate them from one cluster into another cluster. 293 294 ```yaml 295 apiVersion: core.oam.dev/v1beta1 296 kind: Application 297 metadata: 298 name: ref-objects-duplicate-deployments 299 namespace: examples 300 spec: 301 components: 302 - name: duplicate-deployment 303 type: ref-objects 304 properties: 305 objects: 306 - resource: deployment 307 cluster: hangzhou-1 308 # select all deployment in the `examples` namespace in cluster `hangzhou-1` that matches the labelSelector 309 labelSelector: 310 need-duplicate: "true" 311 policies: 312 - name: topology-hangzhou-2 313 type: topology 314 properties: 315 clusters: ["hangzhou-2"] 316 ``` 317 318 You can also form a component by multiple referenced resources and even attach traits to the main workload. 319 320 ```yaml 321 apiVersion: core.oam.dev/v1beta1 322 kind: Application 323 metadata: 324 name: ref-objects-multiple-resources 325 namespace: examples 326 spec: 327 components: 328 - name: nginx-ref-multiple-resources 329 type: ref-objects 330 properties: 331 objects: 332 - resource: deployment 333 - resource: service 334 traits: 335 - type: scaler 336 properties: 337 replicas: 3 338 policies: 339 - name: topology-hangzhou-clusters 340 type: topology 341 properties: 342 clusterLabelSelector: 343 region: hangzhou 344 ``` 345 346 ## External Policies and Workflow 347 348 Sometimes, you may want to use the same policy across multiple applications or reuse previous workflow to deploy different resources. 349 To reduce the repeated code, you can leverage the external policies and workflow and refer to them in your applications. 350 351 > NOTE: you can only refer to Policy and Workflow within your application's namespace. 352 353 ```yaml 354 apiVersion: core.oam.dev/v1alpha1 355 kind: Policy 356 metadata: 357 name: topology-hangzhou-clusters 358 namespace: examples 359 type: topology 360 properties: 361 clusterLabelSelector: 362 region: hangzhou 363 --- 364 apiVersion: core.oam.dev/v1alpha1 365 kind: Policy 366 metadata: 367 name: override-high-availability-webservice 368 namespace: examples 369 type: override 370 properties: 371 components: 372 - type: webservice 373 traits: 374 - type: scaler 375 properties: 376 replicas: 3 377 --- 378 apiVersion: core.oam.dev/v1alpha1 379 kind: Workflow 380 metadata: 381 name: make-release-in-hangzhou 382 namespace: examples 383 steps: 384 - type: deploy 385 name: deploy-hangzhou 386 properties: 387 auto: false 388 policies: ["override-high-availability-webservice", "topology-hangzhou-clusters"] 389 ``` 390 391 ```yaml 392 apiVersion: core.oam.dev/v1beta1 393 kind: Application 394 metadata: 395 name: external-policies-and-workflow 396 namespace: examples 397 spec: 398 components: 399 - name: nginx-external-policies-and-workflow 400 type: webservice 401 properties: 402 image: nginx 403 workflow: 404 ref: make-release-in-hangzhou 405 ``` 406 407 > NOTE: The internal policies will be loaded first. External policies will only be used when there is no corresponding policy inside the application. In the following example, we can reuse `tology-hangzhou-clusters` policy and `make-release-in-hangzhou` workflow but modify the `override-high-availability-webservice` by injecting the same-named policy inside the new application. 408 409 ```yaml 410 apiVersion: core.oam.dev/v1beta1 411 kind: Application 412 metadata: 413 name: nginx-stable-ultra 414 namespace: examples 415 spec: 416 components: 417 - name: nginx-stable-ultra 418 type: webservice 419 properties: 420 image: nginx:stable 421 policies: 422 - name: override-high-availability-webservice 423 type: override 424 properties: 425 components: 426 - type: webservice 427 traits: 428 - type: scaler 429 properties: 430 replicas: 5 431 workflow: 432 ref: make-release-in-hangzhou 433 ```