github.com/operandinc/gqlgen@v0.16.1/README.md (about) 1 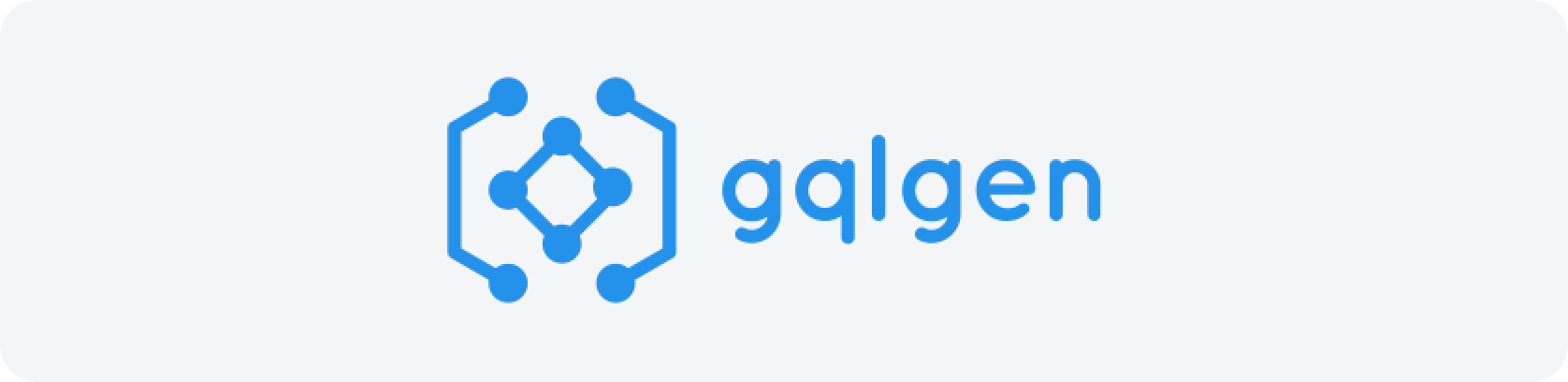 2 3 # gqlgen [](https://github.com/operandinc/gqlgen/actions) [](https://coveralls.io/github/99designs/gqlgen?branch=master) [](https://goreportcard.com/report/github.com/operandinc/gqlgen) [](https://pkg.go.dev/github.com/operandinc/gqlgen) [](http://gqlgen.com/) 4 5 ## What is gqlgen? 6 7 [gqlgen](https://github.com/operandinc/gqlgen) is a Go library for building GraphQL servers without any fuss.<br/> 8 9 - **gqlgen is based on a Schema first approach** — You get to Define your API using the GraphQL [Schema Definition Language](http://graphql.org/learn/schema/). 10 - **gqlgen prioritizes Type safety** — You should never see `map[string]interface{}` here. 11 - **gqlgen enables Codegen** — We generate the boring bits, so you can focus on building your app quickly. 12 13 Still not convinced enough to use **gqlgen**? Compare **gqlgen** with other Go graphql [implementations](https://gqlgen.com/feature-comparison/) 14 15 ## Quick start 16 17 1. [Initialise a new go module](https://golang.org/doc/tutorial/create-module) 18 19 mkdir example 20 cd example 21 go mod init example 22 23 2. Add `github.com/operandinc/gqlgen` to your [project's tools.go](https://github.com/golang/go/wiki/Modules#how-can-i-track-tool-dependencies-for-a-module) 24 25 printf '// +build tools\npackage tools\nimport \_ "github.com/operandinc/gqlgen"' | gofmt > tools.go 26 go mod tidy 27 28 3. Initialise gqlgen config and generate models 29 30 go run github.com/operandinc/gqlgen init 31 printf 'package model' | gofmt > graph/model/doc.go 32 33 4. Start the graphql server 34 35 go run server.go 36 37 More help to get started: 38 39 - [Getting started tutorial](https://gqlgen.com/getting-started/) - a comprehensive guide to help you get started 40 - [Real-world examples](https://github.com/operandinc/gqlgen/tree/master/_examples) show how to create GraphQL applications 41 - [Reference docs](https://pkg.go.dev/github.com/operandinc/gqlgen) for the APIs 42 43 ## Reporting Issues 44 45 If you think you've found a bug, or something isn't behaving the way you think it should, please raise an [issue](https://github.com/operandinc/gqlgen/issues) on GitHub. 46 47 ## Contributing 48 49 We welcome contributions, Read our [Contribution Guidelines](https://github.com/operandinc/gqlgen/blob/master/CONTRIBUTING.md) to learn more about contributing to **gqlgen** 50 51 ## Frequently asked questions 52 53 ### How do I prevent fetching child objects that might not be used? 54 55 When you have nested or recursive schema like this: 56 57 ```graphql 58 type User { 59 id: ID! 60 name: String! 61 friends: [User!]! 62 } 63 ``` 64 65 You need to tell gqlgen that it should only fetch friends if the user requested it. There are two ways to do this; 66 67 - #### Using Custom Models 68 69 Write a custom model that omits the friends field: 70 71 ```go 72 type User struct { 73 ID int 74 Name string 75 } 76 ``` 77 78 And reference the model in `gqlgen.yml`: 79 80 ```yaml 81 # gqlgen.yml 82 models: 83 User: 84 model: github.com/you/pkg/model.User # go import path to the User struct above 85 ``` 86 87 - #### Using Explicit Resolvers 88 89 If you want to Keep using the generated model, mark the field as requiring a resolver explicitly in `gqlgen.yml` like this: 90 91 ```yaml 92 # gqlgen.yml 93 models: 94 User: 95 fields: 96 friends: 97 resolver: true # force a resolver to be generated 98 ``` 99 100 After doing either of the above and running generate we will need to provide a resolver for friends: 101 102 ```go 103 func (r *userResolver) Friends(ctx context.Context, obj *User) ([]*User, error) { 104 // select * from user where friendid = obj.ID 105 return friends, nil 106 } 107 ``` 108 109 You can also use inline config with directives to achieve the same result 110 111 ```graphql 112 directive @goModel( 113 model: String 114 models: [String!] 115 ) on OBJECT | INPUT_OBJECT | SCALAR | ENUM | INTERFACE | UNION 116 117 directive @goField( 118 forceResolver: Boolean 119 name: String 120 ) on INPUT_FIELD_DEFINITION | FIELD_DEFINITION 121 122 type User @goModel(model: "github.com/you/pkg/model.User") { 123 id: ID! @goField(name: "todoId") 124 friends: [User!]! @goField(forceResolver: true) 125 } 126 ``` 127 128 ### Can I change the type of the ID from type String to Type Int? 129 130 Yes! You can by remapping it in config as seen below: 131 132 ```yaml 133 models: 134 ID: # The GraphQL type ID is backed by 135 model: 136 - github.com/operandinc/gqlgen/graphql.IntID # a go integer 137 - github.com/operandinc/gqlgen/graphql.ID # or a go string 138 ``` 139 140 This means gqlgen will be able to automatically bind to strings or ints for models you have written yourself, but the 141 first model in this list is used as the default type and it will always be used when: 142 143 - Generating models based on schema 144 - As arguments in resolvers 145 146 There isn't any way around this, gqlgen has no way to know what you want in a given context. 147 148 ## Other Resources 149 150 - [Christopher Biscardi @ Gophercon UK 2018](https://youtu.be/FdURVezcdcw) 151 - [Introducing gqlgen: a GraphQL Server Generator for Go](https://99designs.com.au/blog/engineering/gqlgen-a-graphql-server-generator-for-go/) 152 - [Dive into GraphQL by Iván Corrales Solera](https://medium.com/@ivan.corrales.solera/dive-into-graphql-9bfedf22e1a) 153 - [Sample Project built on gqlgen with Postgres by Oleg Shalygin](https://github.com/oshalygin/gqlgen-pg-todo-example)