github.com/rancher/moq@v0.0.0-20200712062324-13d1f37d2d77/README.md (about) 1 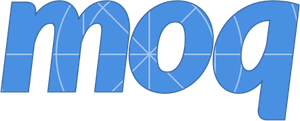 [](https://travis-ci.org/matryer/moq) [](https://goreportcard.com/report/github.com/matryer/moq) 2 3 Interface mocking tool for go generate. 4 5 By [Mat Ryer](https://twitter.com/matryer) and [David Hernandez](https://github.com/dahernan), with ideas lovingly stolen from [Ernesto Jimenez](https://github.com/ernesto-jimenez). 6 7 ### What is Moq? 8 9 Moq is a tool that generates a struct from any interface. The struct can be used in test code as a mock of the interface. 10 11 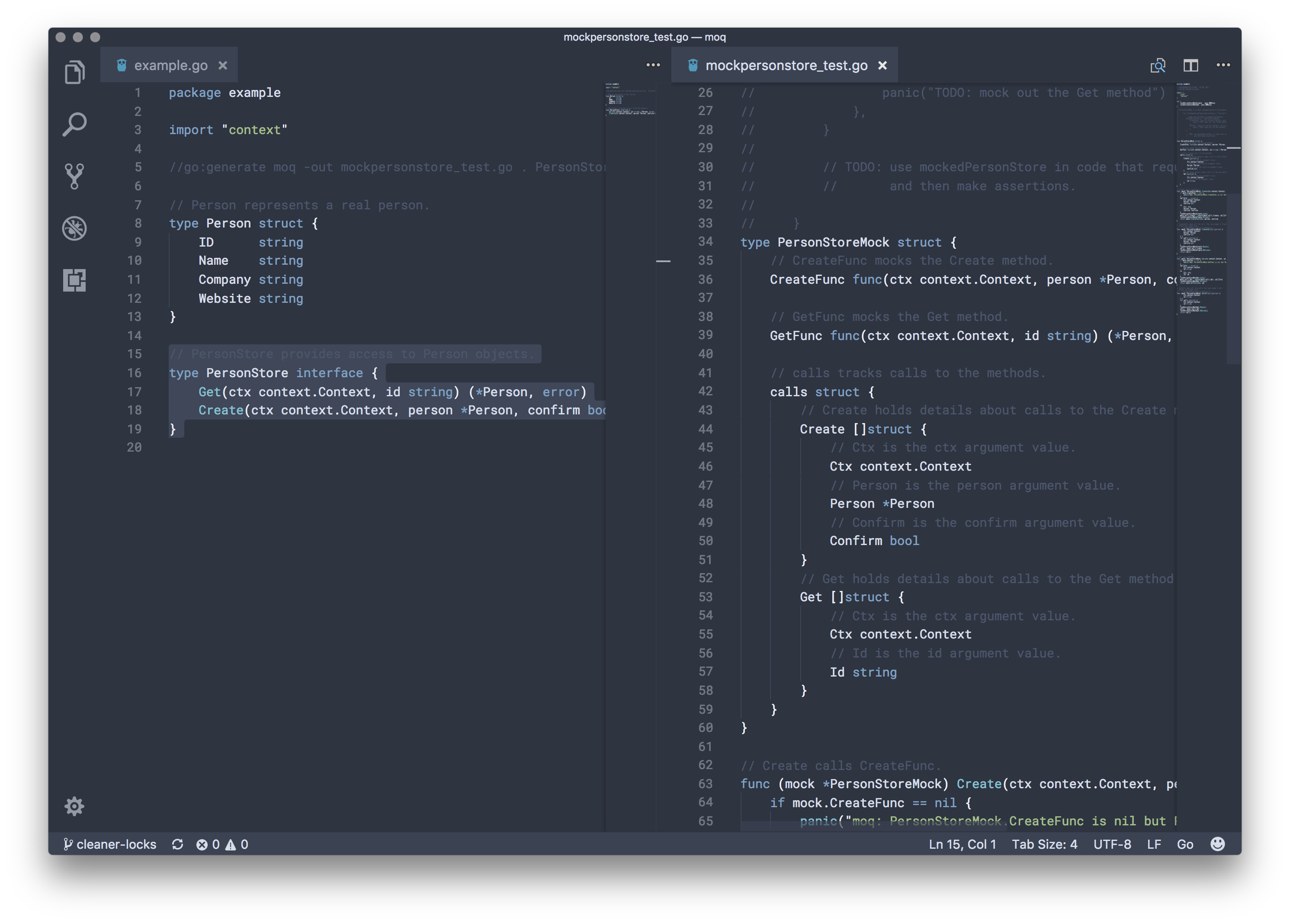 12 13 above: Moq generates the code on the right. 14 15 You can read more in the [Meet Moq blog post](http://bit.ly/meetmoq). 16 17 ### Installing 18 19 To start using Moq, just run go get: 20 ``` 21 $ go get github.com/matryer/moq 22 ``` 23 24 ### Usage 25 26 ``` 27 moq [flags] source-dir interface [interface2 [interface3 [...]]] 28 -fmt string 29 go pretty-printer: gofmt (default) or goimports 30 -out string 31 output file (default stdout) 32 -pkg string 33 package name (default will infer) 34 Specifying an alias for the mock is also supported with the format 'interface:alias' 35 Ex: moq -pkg different . MyInterface:MyMock 36 ``` 37 38 **NOTE:** `source-dir` is the directory where the source code (definition) of the target interface is located. 39 It needs to be a path to a directory and not the import statement for a Go package. 40 41 In a command line: 42 43 ``` 44 $ moq -out mocks_test.go . MyInterface 45 ``` 46 47 In code (for go generate): 48 49 ```go 50 package my 51 52 //go:generate moq -out myinterface_moq_test.go . MyInterface 53 54 type MyInterface interface { 55 Method1() error 56 Method2(i int) 57 } 58 ``` 59 60 Then run `go generate` for your package. 61 62 ### How to use it 63 64 Mocking interfaces is a nice way to write unit tests where you can easily control the behaviour of the mocked object. 65 66 Moq creates a struct that has a function field for each method, which you can declare in your test code. 67 68 In this example, Moq generated the `EmailSenderMock` type: 69 70 ```go 71 func TestCompleteSignup(t *testing.T) { 72 73 var sentTo string 74 75 mockedEmailSender = &EmailSenderMock{ 76 SendFunc: func(to, subject, body string) error { 77 sentTo = to 78 return nil 79 }, 80 } 81 82 CompleteSignUp("me@email.com", mockedEmailSender) 83 84 callsToSend := len(mockedEmailSender.SendCalls()) 85 if callsToSend != 1 { 86 t.Errorf("Send was called %d times", callsToSend) 87 } 88 if sentTo != "me@email.com" { 89 t.Errorf("unexpected recipient: %s", sentTo) 90 } 91 92 } 93 94 func CompleteSignUp(to string, sender EmailSender) { 95 // TODO: this 96 } 97 ``` 98 99 The mocked structure implements the interface, where each method calls the associated function field. 100 101 ## Tips 102 103 * Keep mocked logic inside the test that is using it 104 * Only mock the fields you need 105 * It will panic if a nil function gets called 106 * Name arguments in the interface for a better experience 107 * Use closured variables inside your test function to capture details about the calls to the methods 108 * Use `.MethodCalls()` to track the calls 109 * Use `go:generate` to invoke the `moq` command 110 111 ## License 112 113 The Moq project (and all code) is licensed under the [MIT License](LICENSE). 114 115 The Moq logo was created by [Chris Ryer](http://chrisryer.co.uk) and is licensed under the [Creative Commons Attribution 3.0 License](https://creativecommons.org/licenses/by/3.0/).