github.com/sams1990/dockerrepo@v17.12.1-ce-rc2+incompatible/docs/contributing/set-up-dev-env.md (about) 1 ### Work with a development container 2 3 In this section, you learn to develop like the Moby Engine core team. 4 The `moby/moby` repository includes a `Dockerfile` at its root. This file defines 5 Moby's development environment. The `Dockerfile` lists the environment's 6 dependencies: system libraries and binaries, Go environment, Go dependencies, 7 etc. 8 9 Moby's development environment is itself, ultimately a Docker container. 10 You use the `moby/moby` repository and its `Dockerfile` to create a Docker image, 11 run a Docker container, and develop code in the container. 12 13 If you followed the procedures that [set up Git for contributing](./set-up-git.md), you should have a fork of the `moby/moby` 14 repository. You also created a branch called `dry-run-test`. In this section, 15 you continue working with your fork on this branch. 16 17 ## Task 1. Remove images and containers 18 19 Moby developers run the latest stable release of the Docker software. They clean their local hosts of 20 unnecessary Docker artifacts such as stopped containers or unused images. 21 Cleaning unnecessary artifacts isn't strictly necessary, but it is good 22 practice, so it is included here. 23 24 To remove unnecessary artifacts: 25 26 1. Verify that you have no unnecessary containers running on your host. 27 28 ```none 29 $ docker ps -a 30 ``` 31 32 You should see something similar to the following: 33 34 ```none 35 CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 36 ``` 37 38 There are no running or stopped containers on this host. A fast way to 39 remove old containers is the following: 40 41 You can now use the `docker system prune` command to achieve this: 42 43 ```none 44 $ docker system prune -a 45 ``` 46 47 Older versions of the Docker Engine should reference the command below: 48 49 ```none 50 $ docker rm $(docker ps -a -q) 51 ``` 52 53 This command uses `docker ps` to list all containers (`-a` flag) by numeric 54 IDs (`-q` flag). Then, the `docker rm` command removes the resulting list. 55 If you have running but unused containers, stop and then remove them with 56 the `docker stop` and `docker rm` commands. 57 58 2. Verify that your host has no dangling images. 59 60 ```none 61 $ docker images 62 ``` 63 64 You should see something similar to the following: 65 66 ```none 67 REPOSITORY TAG IMAGE ID CREATED SIZE 68 ``` 69 70 This host has no images. You may have one or more _dangling_ images. A 71 dangling image is not used by a running container and is not an ancestor of 72 another image on your system. A fast way to remove dangling image is 73 the following: 74 75 ```none 76 $ docker rmi -f $(docker images -q -a -f dangling=true) 77 ``` 78 79 This command uses `docker images` to list all images (`-a` flag) by numeric 80 IDs (`-q` flag) and filter them to find dangling images (`-f dangling=true`). 81 Then, the `docker rmi` command forcibly (`-f` flag) removes 82 the resulting list. If you get a "docker: "rmi" requires a minimum of 1 argument." 83 message, that means there were no dangling images. To remove just one image, use the 84 `docker rmi ID` command. 85 86 ## Task 2. Start a development container 87 88 If you followed the last procedure, your host is clean of unnecessary images and 89 containers. In this section, you build an image from the Engine development 90 environment and run it in the container. Both steps are automated for you by the 91 Makefile in the Engine code repository. The first time you build an image, it 92 can take over 15 minutes to complete. 93 94 1. Open a terminal. 95 96 For [Docker Toolbox](../../toolbox/overview.md) users, use `docker-machine status your_vm_name` to make sure your VM is running. You 97 may need to run `eval "$(docker-machine env your_vm_name)"` to initialize your 98 shell environment. If you use Docker for Mac or Docker for Windows, you do not need 99 to use Docker Machine. 100 101 2. Change into the root of the `moby-fork` repository. 102 103 ```none 104 $ cd ~/repos/moby-fork 105 ``` 106 107 If you are following along with this guide, you created a `dry-run-test` 108 branch when you [set up Git for contributing](./set-up-git.md). 109 110 3. Ensure you are on your `dry-run-test` branch. 111 112 ```none 113 $ git checkout dry-run-test 114 ``` 115 116 If you get a message that the branch doesn't exist, add the `-b` flag (`git checkout -b dry-run-test`) so the 117 command both creates the branch and checks it out. 118 119 4. Use `make` to build a development environment image and run it in a container. 120 121 ```none 122 $ make BIND_DIR=. shell 123 ``` 124 125 Using the instructions in the 126 `Dockerfile`, the build may need to download and / or configure source and other images. On first build this process may take between 5 - 15 minutes to create an image. The command returns informational messages as it runs. A 127 successful build returns a final message and opens a Bash shell into the 128 container. 129 130 ```none 131 Successfully built 3d872560918e 132 docker run --rm -i --privileged -e BUILDFLAGS -e KEEPBUNDLE -e DOCKER_BUILD_GOGC -e DOCKER_BUILD_PKGS -e DOCKER_CLIENTONLY -e DOCKER_DEBUG -e DOCKER_EXPERIMENTAL -e DOCKER_GITCOMMIT -e DOCKER_GRAPHDRIVER=devicemapper -e DOCKER_INCREMENTAL_BINARY -e DOCKER_REMAP_ROOT -e DOCKER_STORAGE_OPTS -e DOCKER_USERLANDPROXY -e TESTDIRS -e TESTFLAGS -e TIMEOUT -v "home/ubuntu/repos/docker/bundles:/go/src/github.com/moby/moby/bundles" -t "docker-dev:dry-run-test" bash 133 root@f31fa223770f:/go/src/github.com/docker/docker# 134 ``` 135 136 At this point, your prompt reflects the container's BASH shell. 137 138 5. List the contents of the current directory (`/go/src/github.com/moby/moby`). 139 140 You should see the image's source from the `/go/src/github.com/docker/docker` 141 directory. 142 143 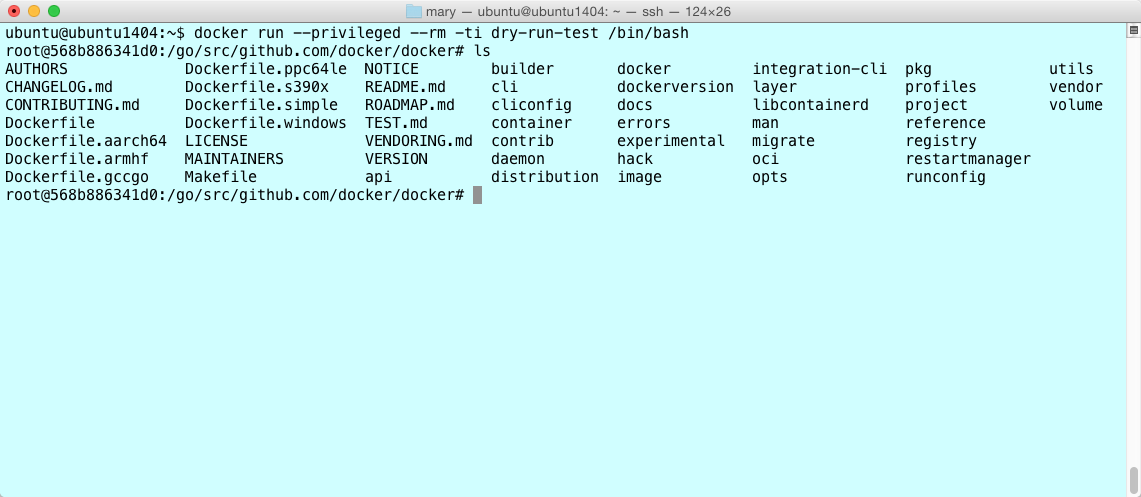 144 145 6. Make a `dockerd` binary. 146 147 ```none 148 root@a8b2885ab900:/go/src/github.com/docker/docker# hack/make.sh binary 149 Removing bundles/ 150 151 ---> Making bundle: binary (in bundles/binary) 152 Building: bundles/binary-daemon/dockerd-17.06.0-dev 153 Created binary: bundles/binary-daemon/dockerd-17.06.0-dev 154 Copying nested executables into bundles/binary-daemon 155 156 ``` 157 158 7. Run `make install`, which copies the binary to the container's 159 `/usr/local/bin/` directory. 160 161 ```none 162 root@a8b2885ab900:/go/src/github.com/docker/docker# make install 163 ``` 164 165 8. Start the Engine daemon running in the background. 166 167 ```none 168 root@a8b2885ab900:/go/src/github.com/docker/docker# dockerd -D & 169 ...output snipped... 170 DEBU[0001] Registering POST, /networks/{id:.*}/connect 171 DEBU[0001] Registering POST, /networks/{id:.*}/disconnect 172 DEBU[0001] Registering DELETE, /networks/{id:.*} 173 INFO[0001] API listen on /var/run/docker.sock 174 DEBU[0003] containerd connection state change: READY 175 ``` 176 177 The `-D` flag starts the daemon in debug mode. The `&` starts it as a 178 background process. You'll find these options useful when debugging code 179 development. You will need to hit `return` in order to get back to your shell prompt. 180 181 > **Note**: The following command automates the `build`, 182 > `install`, and `run` steps above. Once the command below completes, hit `ctrl-z` to suspend the process, then run `bg 1` and hit `enter` to resume the daemon process in the background and get back to your shell prompt. 183 184 ```none 185 hack/make.sh binary install-binary run 186 ``` 187 188 9. Inside your container, check your Docker version. 189 190 ```none 191 root@5f8630b873fe:/go/src/github.com/docker/docker# docker --version 192 Docker version 1.12.0-dev, build 6e728fb 193 ``` 194 195 Inside the container you are running a development version. This is the version 196 on the current branch. It reflects the value of the `VERSION` file at the 197 root of your `docker-fork` repository. 198 199 10. Run the `hello-world` image. 200 201 ```none 202 root@5f8630b873fe:/go/src/github.com/docker/docker# docker run hello-world 203 ``` 204 205 11. List the image you just downloaded. 206 207 ```none 208 root@5f8630b873fe:/go/src/github.com/docker/docker# docker images 209 REPOSITORY TAG IMAGE ID CREATED SIZE 210 hello-world latest c54a2cc56cbb 3 months ago 1.85 kB 211 ``` 212 213 12. Open another terminal on your local host. 214 215 13. List the container running your development container. 216 217 ```none 218 ubuntu@ubuntu1404:~$ docker ps 219 CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 220 a8b2885ab900 docker-dev:dry-run-test "hack/dind bash" 43 minutes ago Up 43 minutes hungry_payne 221 ``` 222 223 Notice that the tag on the container is marked with the `dry-run-test` branch name. 224 225 226 ## Task 3. Make a code change 227 228 At this point, you have experienced the "Moby inception" technique. That is, 229 you have: 230 231 * forked and cloned the Moby Engine code repository 232 * created a feature branch for development 233 * created and started an Engine development container from your branch 234 * built a binary inside of your development container 235 * launched a `docker` daemon using your newly compiled binary 236 * called the `docker` client to run a `hello-world` container inside 237 your development container 238 239 Running the `make BIND_DIR=. shell` command mounted your local Docker repository source into 240 your Docker container. 241 242 > **Note**: Inspecting the `Dockerfile` shows a `COPY . /go/src/github.com/docker/docker` instruction, suggesting that dynamic code changes will _not_ be reflected in the container. However inspecting the `Makefile` shows that the current working directory _will_ be mounted via a `-v` volume mount. 243 244 When you start to develop code though, you'll 245 want to iterate code changes and builds inside the container. If you have 246 followed this guide exactly, you have a bash shell running a development 247 container. 248 249 Try a simple code change and see it reflected in your container. For this 250 example, you'll edit the help for the `attach` subcommand. 251 252 1. If you don't have one, open a terminal in your local host. 253 254 2. Make sure you are in your `moby-fork` repository. 255 256 ```none 257 $ pwd 258 /Users/mary/go/src/github.com/moxiegirl/moby-fork 259 ``` 260 261 Your location should be different because, at least, your username is 262 different. 263 264 3. Open the `cmd/dockerd/docker.go` file. 265 266 4. Edit the command's help message. 267 268 For example, you can edit this line: 269 270 ```go 271 Short: "A self-sufficient runtime for containers.", 272 ``` 273 274 And change it to this: 275 276 ```go 277 Short: "A self-sufficient and really fun runtime for containers.", 278 ``` 279 280 5. Save and close the `cmd/dockerd/docker.go` file. 281 282 6. Go to your running docker development container shell. 283 284 7. Rebuild the binary by using the command `hack/make.sh binary` in the docker development container shell. 285 286 8. Stop Docker if it is running. 287 288 9. Copy the binaries to **/usr/bin** by entering the following commands in the docker development container shell. 289 290 ``` 291 hack/make.sh binary install-binary 292 ``` 293 294 10. To view your change, run the `dockerd --help` command in the docker development container shell. 295 296 ```bash 297 root@b0cb4f22715d:/go/src/github.com/docker/docker# dockerd --help 298 299 Usage: dockerd COMMAND 300 301 A self-sufficient and really fun runtime for containers. 302 303 Options: 304 ... 305 306 ``` 307 308 You've just done the basic workflow for changing the Engine code base. You made 309 your code changes in your feature branch. Then, you updated the binary in your 310 development container and tried your change out. If you were making a bigger 311 change, you might repeat or iterate through this flow several times. 312 313 ## Where to go next 314 315 Congratulations, you have successfully achieved Docker inception. You've had a 316 small experience of the development process. You've set up your development 317 environment and verified almost all the essential processes you need to 318 contribute. Of course, before you start contributing, [you'll need to learn one 319 more piece of the development process, the test framework](test.md).