github.com/sams1990/dockerrepo@v17.12.1-ce-rc2+incompatible/docs/contributing/test.md (about) 1 ### Run tests 2 3 Contributing includes testing your changes. If you change the Moby code, you 4 may need to add a new test or modify an existing test. Your contribution could 5 even be adding tests to Moby. For this reason, you need to know a little 6 about Moby's test infrastructure. 7 8 This section describes tests you can run in the `dry-run-test` branch of your Docker 9 fork. If you have followed along in this guide, you already have this branch. 10 If you don't have this branch, you can create it or simply use another of your 11 branches. 12 13 ## Understand how to test Moby 14 15 Moby tests use the Go language's test framework. In this framework, files 16 whose names end in `_test.go` contain test code; you'll find test files like 17 this throughout the Moby repo. Use these files for inspiration when writing 18 your own tests. For information on Go's test framework, see <a 19 href="http://golang.org/pkg/testing/" target="_blank">Go's testing package 20 documentation</a> and the <a href="http://golang.org/cmd/go/#hdr-Test_packages" 21 target="_blank">go test help</a>. 22 23 You are responsible for _unit testing_ your contribution when you add new or 24 change existing Moby code. A unit test is a piece of code that invokes a 25 single, small piece of code (_unit of work_) to verify the unit works as 26 expected. 27 28 Depending on your contribution, you may need to add _integration tests_. These 29 are tests that combine two or more work units into one component. These work 30 units each have unit tests and then, together, integration tests that test the 31 interface between the components. The `integration` and `integration-cli` 32 directories in the Docker repository contain integration test code. 33 34 Testing is its own specialty. If you aren't familiar with testing techniques, 35 there is a lot of information available to you on the Web. For now, you should 36 understand that, the Docker maintainers may ask you to write a new test or 37 change an existing one. 38 39 ## Run tests on your local host 40 41 Before submitting a pull request with a code change, you should run the entire 42 Moby Engine test suite. The `Makefile` contains a target for the entire test 43 suite, named `test`. Also, it contains several targets for 44 testing: 45 46 | Target | What this target does | 47 | ---------------------- | ---------------------------------------------- | 48 | `test` | Run the unit, integration, and docker-py tests | 49 | `test-unit` | Run just the unit tests | 50 | `test-integration` | Run the integration tests | 51 | `test-docker-py` | Run the tests for the Docker API client | 52 53 Running the entire test suite on your current repository can take over half an 54 hour. To run the test suite, do the following: 55 56 1. Open a terminal on your local host. 57 58 2. Change to the root of your Docker repository. 59 60 ```bash 61 $ cd moby-fork 62 ``` 63 64 3. Make sure you are in your development branch. 65 66 ```bash 67 $ git checkout dry-run-test 68 ``` 69 70 4. Run the `make test` command. 71 72 ```bash 73 $ make test 74 ``` 75 76 This command does several things, it creates a container temporarily for 77 testing. Inside that container, the `make`: 78 79 * creates a new binary 80 * cross-compiles all the binaries for the various operating systems 81 * runs all the tests in the system 82 83 It can take approximate one hour to run all the tests. The time depends 84 on your host performance. The default timeout is 60 minutes, which is 85 defined in `hack/make.sh` (`${TIMEOUT:=60m}`). You can modify the timeout 86 value on the basis of your host performance. When they complete 87 successfully, you see the output concludes with something like this: 88 89 ```none 90 Ran 68 tests in 79.135s 91 ``` 92 93 ## Run targets inside a development container 94 95 If you are working inside a development container, you use the 96 `hack/make.sh` script to run tests. The `hack/make.sh` script doesn't 97 have a single target that runs all the tests. Instead, you provide a single 98 command line with multiple targets that does the same thing. 99 100 Try this now. 101 102 1. Open a terminal and change to the `moby-fork` root. 103 104 2. Start a Moby development image. 105 106 If you are following along with this guide, you should have a 107 `dry-run-test` image. 108 109 ```bash 110 $ docker run --privileged --rm -ti -v `pwd`:/go/src/github.com/moby/moby dry-run-test /bin/bash 111 ``` 112 113 3. Run the tests using the `hack/make.sh` script. 114 115 ```bash 116 root@5f8630b873fe:/go/src/github.com/moby/moby# hack/make.sh dynbinary binary cross test-unit test-integration test-docker-py 117 ``` 118 119 The tests run just as they did within your local host. 120 121 Of course, you can also run a subset of these targets too. For example, to run 122 just the unit tests: 123 124 ```bash 125 root@5f8630b873fe:/go/src/github.com/moby/moby# hack/make.sh dynbinary binary cross test-unit 126 ``` 127 128 Most test targets require that you build these precursor targets first: 129 `dynbinary binary cross` 130 131 132 ## Run unit tests 133 134 We use golang standard [testing](https://golang.org/pkg/testing/) 135 package or [gocheck](https://labix.org/gocheck) for our unit tests. 136 137 You can use the `TESTDIRS` environment variable to run unit tests for 138 a single package. 139 140 ```bash 141 $ TESTDIRS='opts' make test-unit 142 ``` 143 144 You can also use the `TESTFLAGS` environment variable to run a single test. The 145 flag's value is passed as arguments to the `go test` command. For example, from 146 your local host you can run the `TestBuild` test with this command: 147 148 ```bash 149 $ TESTFLAGS='-test.run ^TestValidateIPAddress$' make test-unit 150 ``` 151 152 On unit tests, it's better to use `TESTFLAGS` in combination with 153 `TESTDIRS` to make it quicker to run a specific test. 154 155 ```bash 156 $ TESTDIRS='opts' TESTFLAGS='-test.run ^TestValidateIPAddress$' make test-unit 157 ``` 158 159 ## Run integration tests 160 161 We use [gocheck](https://labix.org/gocheck) for our integration-cli tests. 162 You can use the `TESTFLAGS` environment variable to run a single test. The 163 flag's value is passed as arguments to the `go test` command. For example, from 164 your local host you can run the `TestBuild` test with this command: 165 166 ```bash 167 $ TESTFLAGS='-check.f DockerSuite.TestBuild*' make test-integration 168 ``` 169 170 To run the same test inside your Docker development container, you do this: 171 172 ```bash 173 root@5f8630b873fe:/go/src/github.com/moby/moby# TESTFLAGS='-check.f TestBuild*' hack/make.sh binary test-integration 174 ``` 175 176 ## Test the Windows binary against a Linux daemon 177 178 This explains how to test the Windows binary on a Windows machine set up as a 179 development environment. The tests will be run against a daemon 180 running on a remote Linux machine. You'll use **Git Bash** that came with the 181 Git for Windows installation. **Git Bash**, just as it sounds, allows you to 182 run a Bash terminal on Windows. 183 184 1. If you don't have one open already, start a Git Bash terminal. 185 186 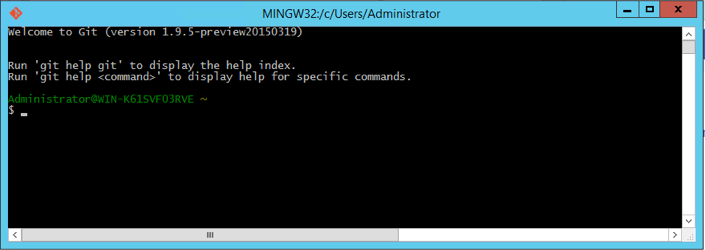 187 188 2. Change to the `moby` source directory. 189 190 ```bash 191 $ cd /c/gopath/src/github.com/moby/moby 192 ``` 193 194 3. Set `DOCKER_REMOTE_DAEMON` as follows: 195 196 ```bash 197 $ export DOCKER_REMOTE_DAEMON=1 198 ``` 199 200 4. Set `DOCKER_TEST_HOST` to the `tcp://IP_ADDRESS:2376` value; substitute your 201 Linux machines actual IP address. For example: 202 203 ```bash 204 $ export DOCKER_TEST_HOST=tcp://213.124.23.200:2376 205 ``` 206 207 5. Make the binary and run the tests: 208 209 ```bash 210 $ hack/make.sh binary test-integration 211 ``` 212 Some tests are skipped on Windows for various reasons. You can see which 213 tests were skipped by re-running the make and passing in the 214 `TESTFLAGS='-test.v'` value. For example 215 216 ```bash 217 $ TESTFLAGS='-test.v' hack/make.sh binary test-integration 218 ``` 219 220 Should you wish to run a single test such as one with the name 221 'TestExample', you can pass in `TESTFLAGS='-check.f TestExample'`. For 222 example 223 224 ```bash 225 $ TESTFLAGS='-check.f TestExample' hack/make.sh binary test-integration 226 ``` 227 228 You can now choose to make changes to the Moby source or the tests. If you 229 make any changes, just run these commands again. 230 231 ## Where to go next 232 233 Congratulations, you have successfully completed the basics you need to 234 understand the Moby test framework.