github.com/secoba/wails/v2@v2.6.4/internal/frontend/desktop/windows/winc/README.md (about) 1 # winc 2 3 ** This is a fork of [tadvi/winc](https://github.com/tadvi/winc) for the sole purpose of integration 4 with [Wails](https://github.com/wailsapp/wails). This repository comes with ***no support*** ** 5 6 Common library for Go GUI apps on Windows. It is for Windows OS only. This makes library smaller than some other UI 7 libraries for Go. 8 9 Design goals: minimalism and simplicity. 10 11 ## Dependencies 12 13 No other dependencies except Go standard library. 14 15 ## Building 16 17 If you want to package icon files and other resources into binary **rsrc** tool is recommended: 18 19 rsrc -manifest app.manifest -ico=app.ico,application_edit.ico,application_error.ico -o rsrc.syso 20 21 Here app.manifest is XML file in format: 22 23 ``` 24 <?xml version="1.0" encoding="UTF-8" standalone="yes"?> 25 <assembly xmlns="urn:schemas-microsoft-com:asm.v1" manifestVersion="1.0"> 26 <assemblyIdentity version="1.0.0.0" processorArchitecture="*" name="App" type="win32"/> 27 <dependency> 28 <dependentAssembly> 29 <assemblyIdentity type="win32" name="Microsoft.Windows.Common-Controls" version="6.0.0.0" processorArchitecture="*" publicKeyToken="6595b64144ccf1df" language="*"/> 30 </dependentAssembly> 31 </dependency> 32 </assembly> 33 ``` 34 35 Most Windows applications do not display command prompt. Build your Go project with flag to indicate that it is Windows 36 GUI binary: 37 38 go build -ldflags="-H windowsgui" 39 40 ## Samples 41 42 Best way to learn how to use the library is to look at the included **examples** projects. 43 44 ## Setup 45 46 1. Make sure you have a working Go installation and build environment, see more for details on page below. 47 http://golang.org/doc/install 48 49 2. go get github.com/wailsapp/wails/v2/internal/frontend/desktop/windows/winc 50 51 ## Icons 52 53 When rsrc is used to pack icons into binary it displays IDs of the packed icons. 54 55 ``` 56 rsrc -manifest app.manifest -ico=app.ico,lightning.ico,edit.ico,application_error.ico -o rsrc.syso 57 Manifest ID: 1 58 Icon app.ico ID: 10 59 Icon lightning.ico ID: 13 60 Icon edit.ico ID: 16 61 Icon application_error.ico ID: 19 62 ``` 63 64 Use IDs to reference packed icons. 65 66 ``` 67 const myIcon = 13 68 69 btn.SetResIcon(myIcon) // Set icon on the button. 70 ``` 71 72 Included source **examples** use basic building via `release.bat` files. Note that icon IDs are order dependent. So if 73 you change they order in -ico flag then icon IDs will be different. If you want to keep order the same, just add new 74 icons to the end of -ico comma separated list. 75 76 ## Layout Manager 77 78 SimpleDock is default layout manager. 79 80 Current design of docking and split views allows building simple apps but if you need to have multiple split views in 81 few different directions you might need to create your own layout manager. 82 83 Important point is to have **one** control inside SimpleDock set to dock as **Fill**. Controls that are not set to any 84 docking get placed using SetPos() function. So you can have Panel set to dock at the Top and then have another dock to 85 arrange controls inside that Panel or have controls placed using SetPos() at fixed positions. 86 87 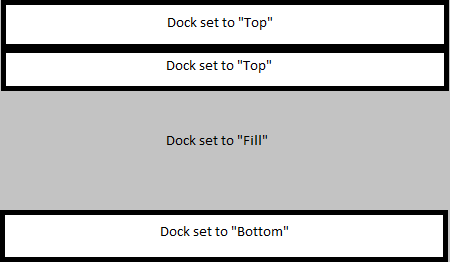 88 89 This is basic layout. Instead of toolbars and status bar you can have Panel or any other control that can resize. Panel 90 can have its own internal Dock that will arrange other controls inside of it. 91 92 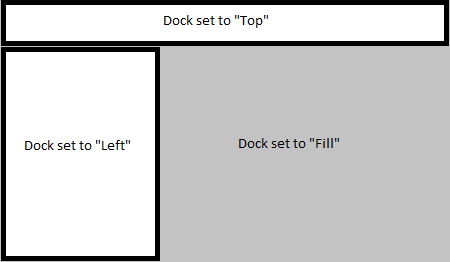 93 94 This is layout with extra control(s) on the left. Left side is usually treeview or listview. 95 96 The rule is simple: you either dock controls using SimpleDock OR use SetPos() to set them at fixed positions. That's it. 97 98 At some point **winc** may get more sophisticated layout manager. 99 100 ## Dialog Screens 101 102 Dialog screens are not based on Windows resource files (.rc). They are just windows with controls placed at fixed 103 coordinates. This works fine for dialog screens up to 10-14 controls. 104 105 # Minimal Demo 106 107 ``` 108 package main 109 110 import ( 111 "github.com/wailsapp/wails/v2/internal/frontend/desktop/windows/winc" 112 ) 113 114 func main() { 115 mainWindow := winc.NewForm(nil) 116 mainWindow.SetSize(400, 300) // (width, height) 117 mainWindow.SetText("Hello World Demo") 118 119 edt := winc.NewEdit(mainWindow) 120 edt.SetPos(10, 20) 121 // Most Controls have default size unless SetSize is called. 122 edt.SetText("edit text") 123 124 btn := winc.NewPushButton(mainWindow) 125 btn.SetText("Show or Hide") 126 btn.SetPos(40, 50) // (x, y) 127 btn.SetSize(100, 40) // (width, height) 128 btn.OnClick().Bind(func(e *winc.Event) { 129 if edt.Visible() { 130 edt.Hide() 131 } else { 132 edt.Show() 133 } 134 }) 135 136 mainWindow.Center() 137 mainWindow.Show() 138 mainWindow.OnClose().Bind(wndOnClose) 139 140 winc.RunMainLoop() // Must call to start event loop. 141 } 142 143 func wndOnClose(arg *winc.Event) { 144 winc.Exit() 145 } 146 ``` 147 148  149 150 Result of running sample_minimal. 151 152 ## Create Your Own 153 154 It is good practice to create your own controls based on existing structures and event model. Library contains some of 155 the controls built that way: IconButton (button.go), ErrorPanel (panel.go), MultiEdit (edit.go), etc. Please look at 156 existing controls as examples before building your own. 157 158 When designing your own controls keep in mind that types have to be converted from Go into Win32 API and back. This is 159 usually due to string UTF8 and UTF16 conversions. But there are other types of conversions too. 160 161 When developing your own controls you might also need to: 162 163 import "github.com/wailsapp/wails/v2/internal/frontend/desktop/windows/winc/w32" 164 165 w32 has Win32 API low level constants and functions. 166 167 Look at **sample_control** for example of custom built window. 168 169 ## Companion Package 170 171 [Go package for Windows Systray icon, menu and notifications](https://github.com/tadvi/systray) 172 173 ## Credits 174 175 This library is built on 176 177 [AllenDang/gform Windows GUI framework for Go](https://github.com/AllenDang/gform) 178 179 **winc** takes most design decisions from **gform** and adds many more controls and code samples to it. 180 181