github.com/servernoj/jade@v0.0.0-20231225191405-efec98d19db1/README.md (about) 1 # Jade.go - template engine for Go (golang) 2 Package jade (github.com/Joker/jade) is a simple and fast template engine implementing Jade/Pug template. 3 Jade precompiles templates to Go code or generates html/template. 4 Now Jade-lang is renamed to [Pug template engine](https://pugjs.org/language/tags.html). 5 6 [](https://pkg.go.dev/github.com/Joker/jade#section-documentation) [](https://goreportcard.com/report/github.com/Joker/jade) 7 8 ## Jade/Pug syntax 9 example: 10 11 ```jade 12 //- :go:func Index(pageTitle string, youAreUsingJade bool) 13 14 mixin for(golang) 15 #cmd Precompile jade templates to #{golang} code. 16 17 doctype html 18 html(lang="en") 19 head 20 title= pageTitle 21 script(type='text/javascript'). 22 if(question){ 23 answer(40 + 2) 24 } 25 body 26 h1 Jade - template engine 27 +for('Go') 28 29 #container.col 30 if youAreUsingJade 31 p You are amazing 32 else 33 p Get on it! 34 p. 35 Jade/Pug is a terse and simple 36 templating language with 37 a #[strong focus] on performance 38 and powerful features. 39 ``` 40 41 becomes 42 43 ```html 44 <!DOCTYPE html> 45 <html lang="en"> 46 <head> 47 <title>Jade.go</title> 48 <script type="text/javascript"> 49 if(question){ 50 answer(40 + 2) 51 } 52 </script> 53 </head> 54 <body> 55 <h1>Jade - template engine 56 <div id="cmd">Precompile jade templates to Go code.</div> 57 </h1> 58 <div id="container" class="col"> 59 <p>You are amazing</p> 60 <p> 61 Jade/Pug is a terse and simple 62 templating language with 63 a <strong>focus</strong> on performance 64 and powerful features. 65 </p> 66 </div> 67 </body> 68 </html> 69 ``` 70 71 Here are additional [examples](https://github.com/Joker/jade/tree/master/example) and [test cases](https://github.com/Joker/jade/tree/master/testdata/v2). 72 73 ## Installation 74 Install [jade compiler](https://github.com/Joker/jade/tree/master/cmd/jade) 75 ```console 76 go install github.com/Joker/jade/cmd/jade@latest 77 ``` 78 or github.com/Joker/jade package 79 ```console 80 go get -u github.com/Joker/jade 81 ``` 82 83 ## Example usage 84 85 ### jade compiler 86 ```console 87 jade -writer -pkg=main hello.jade 88 ``` 89 90 jade command[^1] precompiles _hello.jade_ to _hello.jade.go_ 91 92 `hello.jade` 93 ``` 94 :go:func(arg) word string 95 doctype 5 96 html 97 body 98 p Hello #{word}! 99 ``` 100 101 `hello.jade.go` 102 ```go 103 // Code generated by "jade.go"; DO NOT EDIT. 104 package main 105 106 import "io" 107 108 const ( 109 hello__0 = `<!DOCTYPE html><html><body><p>Hello ` 110 hello__1 = `!</p></body></html>` 111 ) 112 func Jade_hello(word string, wr io.Writer) { 113 buffer := &WriterAsBuffer{wr} 114 buffer.WriteString(hello__0) 115 WriteEscString(word, buffer) 116 buffer.WriteString(hello__1) 117 } 118 ``` 119 120 `main.go` 121 ```go 122 package main 123 //go:generate jade -pkg=main -writer hello.jade 124 125 import "net/http" 126 127 func main() { 128 http.HandleFunc("/", func(wr http.ResponseWriter, req *http.Request) { 129 Jade_hello("jade", wr) 130 }) 131 http.ListenAndServe(":8080", nil) 132 } 133 ``` 134 135 output at localhost:8080 136 ```html 137 <!DOCTYPE html><html><body><p>Hello jade!</p></body></html> 138 ``` 139 140 ### github.com/Joker/jade package 141 generate [`html/template`](https://pkg.go.dev/html/template#hdr-Introduction) at runtime 142 (This case is slightly slower and doesn't support[^2] all features of Jade.go) 143 144 ```go 145 package main 146 147 import ( 148 "fmt" 149 "html/template" 150 "net/http" 151 152 "github.com/Joker/hpp" // Prettify HTML 153 "github.com/Joker/jade" 154 ) 155 156 func handler(w http.ResponseWriter, r *http.Request) { 157 jadeTpl, _ := jade.Parse("jade", []byte("doctype 5\n html: body: p Hello #{.Word} !")) 158 goTpl, _ := template.New("html").Parse(jadeTpl) 159 160 fmt.Printf("output:%s\n\n", hpp.PrPrint(jadeTpl)) 161 goTpl.Execute(w, struct{ Word string }{"jade"}) 162 } 163 164 func main() { 165 http.HandleFunc("/", handler) 166 http.ListenAndServe(":8080", nil) 167 } 168 ``` 169 170 console output 171 ```html 172 <!DOCTYPE html> 173 <html> 174 <body> 175 <p>Hello {{.Word}} !</p> 176 </body> 177 </html> 178 ``` 179 180 output at localhost:8080 181 ```html 182 <!DOCTYPE html><html><body><p>Hello jade !</p></body></html> 183 ``` 184 185 ## Performance 186 The data of chart comes from [SlinSo/goTemplateBenchmark](https://github.com/SlinSo/goTemplateBenchmark). 187 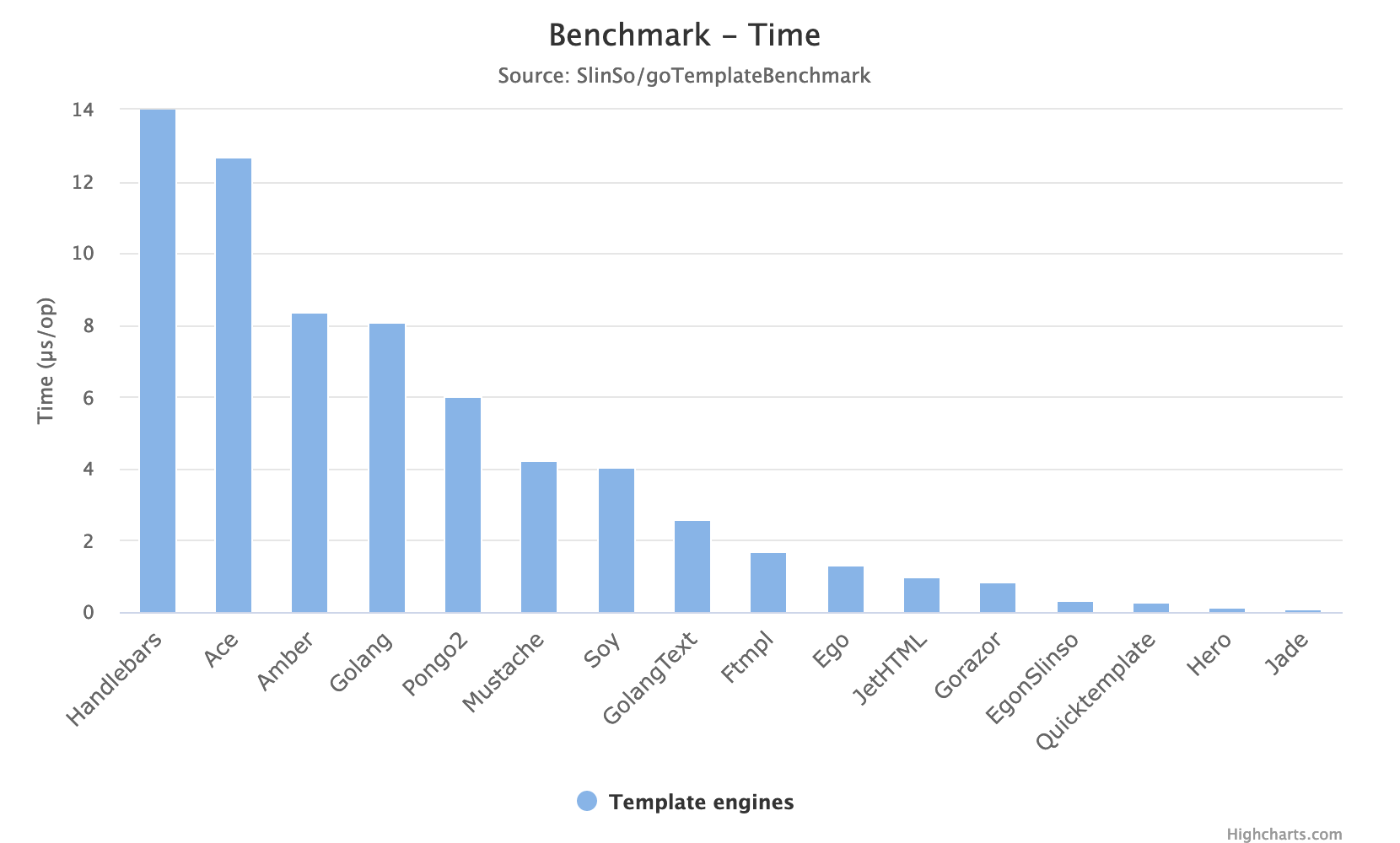 188 189 ## Custom filter :go 190 This filter is used as helper for command line tool 191 (to set imports, function name and parameters). 192 Filter may be placed at any nesting level. 193 When Jade used as library :go filter is not needed. 194 195 ### Nested filter :func 196 ``` 197 :go:func 198 CustomNameForTemplateFunc(any []int, input string, args map[string]int) 199 200 :go:func(name) 201 OnlyCustomNameForTemplateFunc 202 203 :go:func(args) 204 (only string, input float32, args uint) 205 ``` 206 207 ### Nested filter :import 208 ``` 209 :go:import 210 "github.com/Joker/jade" 211 github.com/Joker/hpp 212 ``` 213 214 #### note 215 [^1]: 216 `Usage: ./jade [OPTION]... [FILE]...` 217 ``` 218 -basedir string 219 base directory for templates (default "./") 220 -d string 221 directory for generated .go files (default "./") 222 -fmt 223 HTML pretty print output for generated functions 224 -inline 225 inline HTML in generated functions 226 -pkg string 227 package name for generated files (default "jade") 228 -stdbuf 229 use bytes.Buffer [default bytebufferpool.ByteBuffer] 230 -stdlib 231 use stdlib functions 232 -writer 233 use io.Writer for output 234 ``` 235 [^2]: 236 Runtime `html/template` generation doesn't support the following features: 237 `=>` means it generate the folowing template 238 ``` 239 for => "{{/* %s, %s */}}{{ range %s }}" 240 for if => "{{ if gt len %s 0 }}{{/* %s, %s */}}{{ range %s }}" 241 242 multiline code => "{{/* %s */}}" 243 inheritance block => "{{/* block */}}" 244 245 case statement => "{{/* switch %s */}}" 246 when => "{{/* case %s: */}}" 247 default => "{{/* default: */}}" 248 ``` 249 You can change this behaviour in [`config.go`](https://github.com/Joker/jade/blob/master/config.go#L24) file. 250 Partly this problem can be solved by [custom](https://pkg.go.dev/text/template#example-Template-Func) functions.