github.com/true-sqn/fabric@v2.1.1+incompatible/docs/source/developapps/connectionoptions.md (about) 1 # Connection Options 2 3 **Audience**: Architects, administrators, application and smart contract 4 developers 5 6 Connection options are used in conjunction with a connection profile to control 7 *precisely* how a gateway interacts with a network. Using a gateway allows an 8 application to focus on business logic rather than network topology. 9 10 In this topic, we're going to cover: 11 12 * [Why connection options are important](#scenario) 13 * [How an application uses connection options](#usage) 14 * [What each connection option does](#options) 15 * [When to use a particular connection option](#considerations) 16 17 ## Scenario 18 19 A connection option specifies a particular aspect of a gateway's behaviour. 20 Gateways are important for [many reasons](./gateway.html), the primary being to 21 allow an application to focus on business logic and smart contracts, while it 22 manages interactions with the many components of a network. 23 24 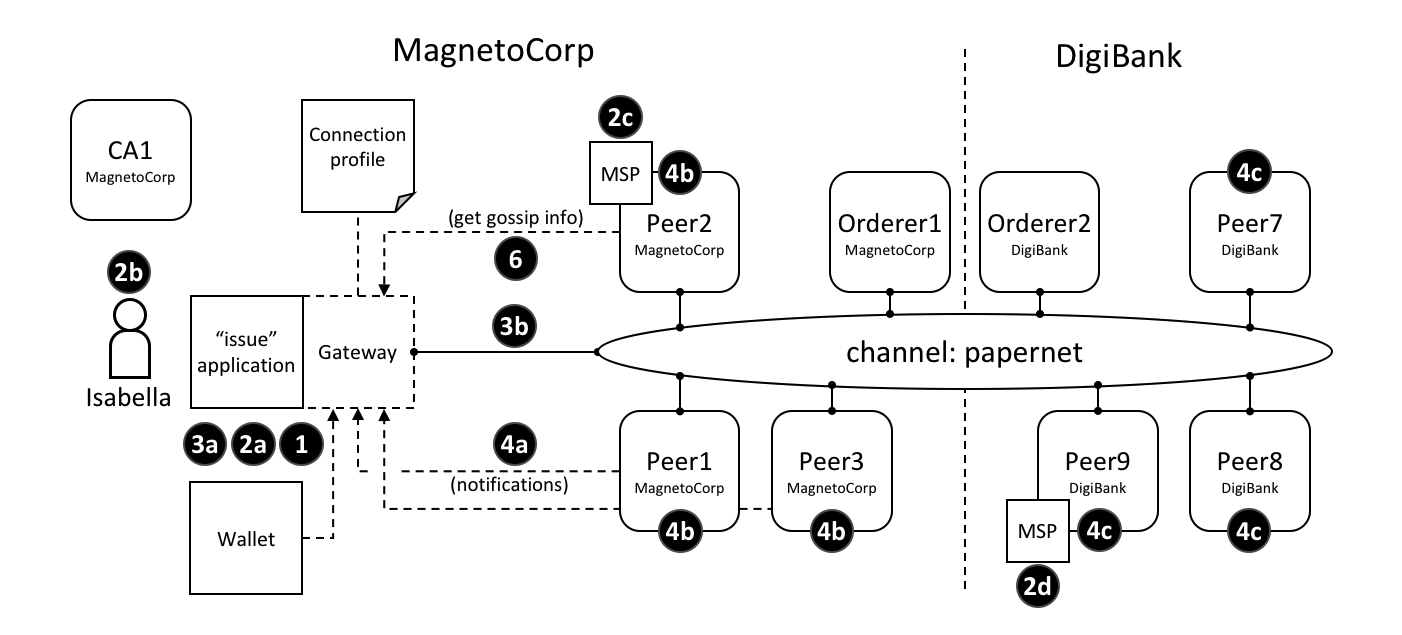 *The different interaction points 25 where connection options control behaviour. These options are explained fully in 26 the text.* 27 28 One example of a connection option might be to specify that the gateway used by 29 the `issue` application should use identity `Isabella` to submit transactions to 30 the `papernet` network. Another might be that a gateway should wait for all 31 three nodes from MagnetoCorp to confirm a transaction has been committed 32 returning control. Connection options allow applications to specify the precise 33 behaviour of a gateway's interaction with the network. Without a gateway, 34 applications need to do a lot more work; gateways save you time, make your 35 application more readable, and less error prone. 36 37 ## Usage 38 39 We'll describe the [full set](#options) of connection options available to an 40 application in a moment; let's first see how they are specified by the 41 sample MagnetoCorp `issue` application: 42 43 ```javascript 44 const userName = 'User1@org1.example.com'; 45 const wallet = new FileSystemWallet('../identity/user/isabella/wallet'); 46 47 const connectionOptions = { 48 identity: userName, 49 wallet: wallet, 50 eventHandlerOptions: { 51 commitTimeout: 100, 52 strategy: EventStrategies.MSPID_SCOPE_ANYFORTX 53 } 54 }; 55 56 await gateway.connect(connectionProfile, connectionOptions); 57 ``` 58 59 See how the `identity` and `wallet` options are simple properties of the 60 `connectionOptions` object. They have values `userName` and `wallet` 61 respectively, which were set earlier in the code. Contrast these options with 62 the `eventHandlerOptions` option which is an object in its own right. It has 63 two properties: `commitTimeout: 100` (measured in seconds) and `strategy: 64 EventStrategies.MSPID_SCOPE_ANYFORTX`. 65 66 See how `connectionOptions` is passed to a gateway as a complement to 67 `connectionProfile`; the network is identified by the connection profile and 68 the options specify precisely how the gateway should interact with it. Let's now 69 look at the available options. 70 71 ## Options 72 73 Here's a list of the available options and what they do. 74 75 * `wallet` identifies the wallet that will be used by the gateway on behalf of 76 the application. See interaction **1**; the wallet is specified by the 77 application, but it's actually the gateway that retrieves identities from it. 78 79 A wallet must be specified; the most important decision is the 80 [type](./wallet.html#type) of wallet to use, whether that's file system, 81 in-memory, HSM or database. 82 83 84 * `identity` is the user identity that the application will use from `wallet`. 85 See interaction **2a**; the user identity is specified by the application and 86 represents the user of the application, Isabella, **2b**. The identity is 87 actually retrieved by the gateway. 88 89 In our example, Isabella's identity will be used by different MSPs (**2c**, 90 **2d**) to identify her as being from MagnetoCorp, and having a particular 91 role within it. These two facts will correspondingly determine her permission 92 over resources, such as being able to read and write the ledger, for example. 93 94 A user identity must be specified. As you can see, this identity is 95 fundamental to the idea that Hyperledger Fabric is a *permissioned* network -- 96 all actors have an identity, including applications, peers and orderers, which 97 determines their control over resources. You can read more about this idea in the membership services [topic](../membership/membership.html). 98 99 100 * `clientTlsIdentity` is the identity that is retrieved from a wallet (**3a**) 101 and used for secure communications (**3b**) between the gateway and different 102 channel components, such as peers and orderers. 103 104 Note that this identity is different to the user identity. Even though 105 `clientTlsIdentity` is important for secure communications, it is not as 106 foundational as the user identity because its scope does not extend beyond 107 secure network communications. 108 109 `clientTlsIdentity` is optional. You are advised to set it in production 110 environments. You should always use a different `clientTlsIdentity` to 111 `identity` because these identities have very different meanings and 112 lifecycles. For example, if your `clientTlsIdentity` was compromised, then so 113 would your `identity`; it's more secure to keep them separate. 114 115 116 * `eventHandlerOptions.commitTimeout` is optional. It specifies, in seconds, the 117 maximum amount of time the gateway should wait for a transaction to be 118 committed by any peer (**4a**) before returning control to the application. 119 The set of peers to use for notification is determined by the 120 `eventHandlerOptions.strategy` option. If a commitTimeout is not 121 specified, the gateway will use a timeout of 300 seconds. 122 123 124 * `eventHandlerOptions.strategy` is optional. It identifies the set of peers 125 that a gateway should use to listen for notification that a transaction has 126 been committed. For example, whether to listen for a single peer, or all 127 peers, from its organization. It can take one of the following values: 128 129 * `EventStrategies.MSPID_SCOPE_ANYFORTX` Listen for **any** peer within the 130 user's organization. In our example, see interaction points **4b**; any of 131 peer 1, peer 2 or peer 3 from MagnetoCorp can notify the gateway. 132 133 * `EventStrategies.MSPID_SCOPE_ALLFORTX` **This is the default value**. Listen 134 for **all** peers within the user's organization. In our example peer, see 135 interaction point **4b**. All peers from MagnetoCorp must all have notified 136 the gateway; peer 1, peer 2 and peer 3. Peers are only counted if they are 137 known/discovered and available; peers that are stopped or have failed are 138 not included. 139 140 * `EventStrategies.NETWORK_SCOPE_ANYFORTX` Listen for **any** peer within the 141 entire network channel. In our example, see interaction points **4b** and 142 **4c**; any of peer 1-3 from MagnetoCorp or peer 7-9 of DigiBank can notify 143 the gateway. 144 145 * `EventStrategies.NETWORK_SCOPE_ALLFORTX` Listen for **all** peers within the 146 entire network channel. In our example, see interaction points **4b** and 147 **4c**. All peers from MagnetoCorp and DigiBank must notify the gateway; 148 peers 1-3 and peers 7-9. Peers are only counted if they are known/discovered 149 and available; peers that are stopped or have failed are not included. 150 151 * <`PluginEventHandlerFunction`> The name of a user-defined event handler. 152 This allows a user to define their own logic for event handling. See how to 153 [define](https://hyperledger.github.io/fabric-sdk-node/{BRANCH}/tutorial-transaction-commit-events.html) 154 a plugin event handler, and examine a [sample 155 handler](https://github.com/hyperledger/fabric-sdk-node/blob/{BRANCH}/test/integration/network-e2e/sample-transaction-event-handler.js). 156 157 A user-defined event handler is only necessary if you have very specific 158 event handling requirements; in general, one of the built-in event 159 strategies will be sufficient. An example of a user-defined event handler 160 might be to wait for more than half the peers in an organization to confirm 161 a transaction has been committed. 162 163 If you do specify a user-defined event handler, it does not affect your 164 application logic; it is quite separate from it. The handler is called by 165 the SDK during processing; it decides when to call it, and uses its results 166 to select which peers to use for event notification. The application 167 receives control when the SDK has finished its processing. 168 169 If a user-defined event handler is not specified then the default values for 170 `EventStrategies` are used. 171 172 173 * `discovery.enabled` is optional and has possible values `true` or `false`. The 174 default is `true`. It determines whether the gateway uses [service 175 discovery](../discovery-overview.html) to augment the network topology 176 specified in the connection profile. See interaction point **6**; peer's 177 gossip information used by the gateway. 178 179 This value will be overridden by the `INITIALIIZE-WITH-DISCOVERY` environment 180 variable, which can be set to `true` or `false`. 181 182 183 * `discovery.asLocalhost` is optional and has possible values `true` or `false`. 184 The default is `true`. It determines whether IP addresses found during service 185 discovery are translated from the docker network to the local host. 186 187 Typically developers will write applications that use docker containers for 188 their network components such as peers, orderers and CAs, but that do not run 189 in docker containers themselves. This is why `true` is the default; in 190 production environments, applications will likely run in docker containers in 191 the same manner as network components and therefore address translation is not 192 required. In this case, applications should either explicitly specify `false` 193 or use the environment variable override. 194 195 This value will be overridden by the `DISCOVERY-AS-LOCALHOST` environment 196 variable, which can be set to `true` or `false`. 197 198 ## Considerations 199 200 The following list of considerations is helpful when deciding how to choose 201 connection options. 202 203 * `eventHandlerOptions.commitTimeout` and `eventHandlerOptions.strategy` work 204 together. For example, `commitTimeout: 100` and `strategy: 205 EventStrategies.MSPID_SCOPE_ANYFORTX` means that the gateway will wait for up 206 to 100 seconds for *any* peer to confirm a transaction has been committed. In 207 contrast, specifying `strategy: EventStrategies.NETWORK_SCOPE_ALLFORTX` means 208 that the gateway will wait up to 100 seconds for *all* peers in *all* 209 organizations. 210 211 212 * The default value of `eventHandlerOptions.strategy: 213 EventStrategies.MSPID_SCOPE_ALLFORTX` will wait for all peers in the 214 application's organization to commit the transaction. This is a good default 215 because applications can be sure that all their peers have an up-to-date copy 216 of the ledger, minimizing concurrency 217 issues <!-- Add a link with more information explaining this topic--> 218 219 However, as the number of peers in an organization grows, it becomes a little 220 unnecessary to wait for all peers, in which case using a pluggable event 221 handler can provide a more efficient strategy. For example the same set of 222 peers could be used to submit transactions and listen for notifications, on 223 the safe assumption that consensus will keep all ledgers synchronized. 224 225 226 * Service discovery requires `clientTlsIdentity` to be set. That's because the 227 peers exchanging information with an application need to be confident that 228 they are exchanging information with entities they trust. If 229 `clientTlsIdentity` is not set, then `discovery` will not be obeyed, 230 regardless of whether or not it is set. 231 232 233 * Although applications can set connection options when they connect to the 234 gateway, it can be necessary for these options to be overridden by an 235 administrator. That's because options relate to network interactions, which 236 can vary over time. For example, an administrator trying to understand the 237 effect of using service discovery on network performance. 238 239 A good approach is to define application overrides in a configuration file 240 which is read by the application when it configures its connection to the 241 gateway. 242 243 Because the discovery options `enabled` and `asLocalHost` are most frequently 244 required to be overridden by administrators, the environment variables 245 `INITIALIIZE-WITH-DISCOVERY` and `DISCOVERY-AS-LOCALHOST` are provided for 246 convenience. The administrator should set these in the production runtime 247 environment of the application, which will most likely be a docker container. 248 249 <!--- Licensed under Creative Commons Attribution 4.0 International License 250 https://creativecommons.org/licenses/by/4.0/ -->