github.com/true-sqn/fabric@v2.1.1+incompatible/docs/source/developapps/gateway.md (about) 1 # Gateway 2 3 **Audience**: Architects, application and smart contract developers 4 5 A gateway manages the network interactions on behalf of an application, allowing 6 it to focus on business logic. Applications connect to a gateway and then all 7 subsequent interactions are managed using that gateway's configuration. 8 9 In this topic, we're going to cover: 10 11 * [Why gateways are important](#scenario) 12 * [How applications use a gateway](#connect) 13 * [How to define a static gateway](#static) 14 * [How to define a dynamic gateway for service discovery](#dynamic) 15 * [Using multiple gateways](#multiple-gateways) 16 17 ## Scenario 18 19 A Hyperledger Fabric network channel can constantly change. The peer, orderer 20 and CA components, contributed by the different organizations in the network, 21 will come and go. Reasons for this include increased or reduced business demand, 22 and both planned and unplanned outages. A gateway relieves an application of 23 this burden, allowing it to focus on the business problem it is trying to solve. 24 25 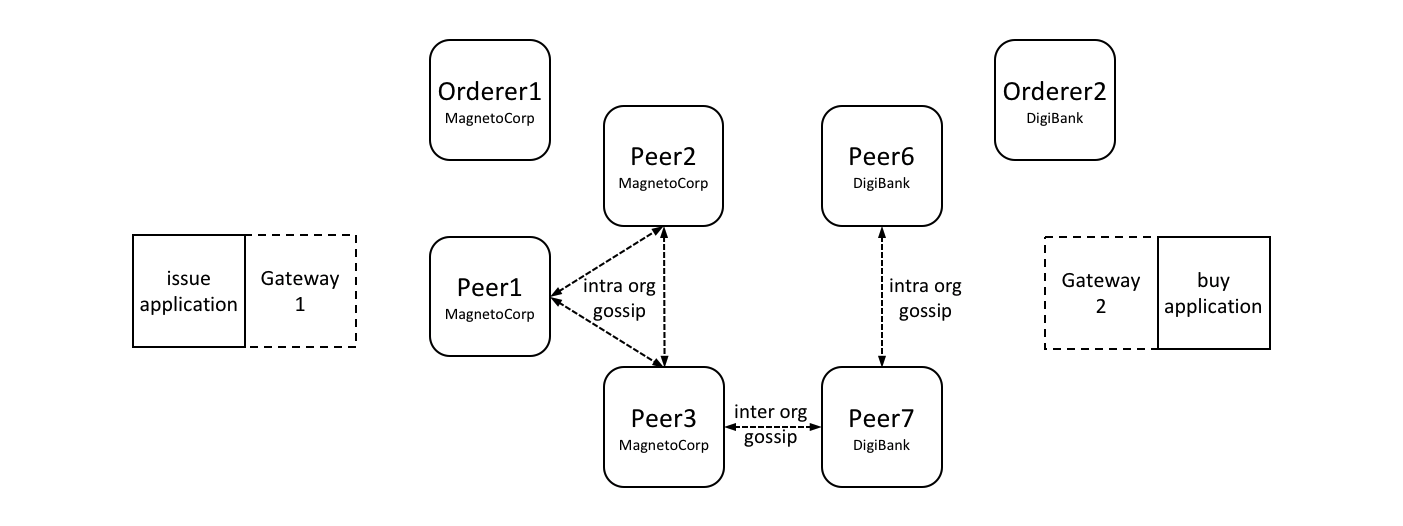 *A MagnetoCorp and DigiBank 26 applications (issue and buy) delegate their respective network interactions to 27 their gateways. Each gateway understands the network channel topology comprising 28 the multiple peers and orderers of two organizations MagnetoCorp and DigiBank, 29 leaving applications to focus on business logic. Peers can talk to each other 30 both within and across organizations using the gossip protocol.* 31 32 A gateway can be used by an application in two different ways: 33 34 * **Static**: The gateway configuration is *completely* defined in a [connection 35 profile](./connectionprofile.html). All the peers, orderers and CAs 36 available to an application are statically defined in the connection profile 37 used to configure the gateway. For peers, this includes their role as an 38 endorsing peer or event notification hub, for example. You can read more about 39 these roles in the connection profile [topic](./connectionprofile.html). 40 41 The SDK will use this static topology, in conjunction with gateway 42 [connection options](./connectionoptions), to manage the transaction 43 submission and notification processes. The connection profile must contain 44 enough of the network topology to allow a gateway to interact with the 45 network on behalf of the application; this includes the network channels, 46 organizations, orderers, peers and their roles. 47 48 49 * **Dynamic**: The gateway configuration is minimally defined in a connection 50 profile. Typically, one or two peers from the application's organization are 51 specified, and they use [service discovery](../discovery-overview.html) to 52 discover the available network topology. This includes peers, orderers, 53 channels, deployed smart contracts and their endorsement policies. (In 54 production environments, a gateway configuration should specify at least two 55 peers for availability.) 56 57 The SDK will use all of the static and discovered topology information, in 58 conjunction with gateway connection options, to manage the transaction 59 submission and notification processes. As part of this, it will also 60 intelligently use the discovered topology; for example, it will *calculate* 61 the minimum required endorsing peers using the discovered endorsement policy 62 for the smart contract. 63 64 You might ask yourself whether a static or dynamic gateway is better? The 65 trade-off is between predictability and responsiveness. Static networks will 66 always behave the same way, as they perceive the network as unchanging. In this 67 sense they are predictable -- they will always use the same peers and orderers 68 if they are available. Dynamic networks are more responsive as they understand 69 how the network changes -- they can use newly added peers and orderers, which 70 brings extra resilience and scalability, at potentially some cost in 71 predictability. In general it's fine to use dynamic networks, and indeed this 72 the default mode for gateways. 73 74 Note that the *same* connection profile can be used statically or dynamically. 75 Clearly, if a profile is going to be used statically, it needs to be 76 comprehensive, whereas dynamic usage requires only sparse population. 77 78 Both styles of gateway are transparent to the application; the application 79 program design does not change whether static or dynamic gateways are used. This 80 also means that some applications may use service discovery, while others may 81 not. In general using dynamic discovery means less definition and more 82 intelligence by the SDK; it is the default. 83 84 ## Connect 85 86 When an application connects to a gateway, two options are provided. These are 87 used in subsequent SDK processing: 88 89 ```javascript 90 await gateway.connect(connectionProfile, connectionOptions); 91 ``` 92 93 * **Connection profile**: `connectionProfile` is the gateway configuration that 94 will be used for transaction processing by the SDK, whether statically or 95 dynamically. It can be specified in YAML or JSON, though it must be converted 96 to a JSON object when passed to the gateway: 97 98 ```javascript 99 let connectionProfile = yaml.safeLoad(fs.readFileSync('../gateway/paperNet.yaml', 'utf8')); 100 ``` 101 102 Read more about [connection profiles](./connectionprofile.html) and how 103 to configure them. 104 105 106 * **Connection options**: `connectionOptions` allow an application to declare 107 rather than implement desired transaction processing behaviour. Connection 108 options are interpreted by the SDK to control interaction patterns with 109 network components, for example to select which identity to connect with, or 110 which peers to use for event notifications. These options significantly reduce 111 application complexity without compromising functionality. This is possible 112 because the SDK has implemented much of the low level logic that would 113 otherwise be required by applications; connection options control this logic 114 flow. 115 116 Read about the list of available [connection options](./connectionoptions.html) 117 and when to use them. 118 119 ## Static 120 121 Static gateways define a fixed view of a network. In the MagnetoCorp 122 [scenario](#scenario), a gateway might identify a single peer from MagnetoCorp, 123 a single peer from DigiBank, and a MagentoCorp orderer. Alternatively, a gateway 124 might define *all* peers and orderers from MagnetCorp and DigiBank. In both 125 cases, a gateway must define a view of the network sufficient to get commercial 126 paper transactions endorsed and distributed. 127 128 Applications can use a gateway statically by explicitly specifying the connect 129 option `discovery: { enabled:false }` on the `gateway.connect()` API. 130 Alternatively, the environment variable setting `FABRIC_SDK_DISCOVERY=false` 131 will always override the application choice. 132 133 Examine the [connection 134 profile](https://github.com/hyperledger/fabric-samples/blob/{BRANCH}/commercial-paper/organization/magnetocorp/gateway/networkConnection.yaml) 135 used by the MagnetoCorp issue application. See how all the peers, orderers and 136 even CAs are specified in this file, including their roles. 137 138 It's worth bearing in mind that a static gateway represents a view of a network 139 at a *moment in time*. As networks change, it may be important to reflect this 140 in a change to the gateway file. Applications will automatically pick up these 141 changes when they re-load the gateway file. 142 143 ## Dynamic 144 145 Dynamic gateways define a small, fixed *starting point* for a network. In the 146 MagnetoCorp [scenario](#scenario), a dynamic gateway might identify just a 147 single peer from MagnetoCorp; everything else will be discovered! (To provide 148 resiliency, it might be better to define two such bootstrap peers.) 149 150 If [service discovery](../discovery-overview.html) is selected by an 151 application, the topology defined in the gateway file is augmented with that 152 produced by this process. Service discovery starts with the gateway definition, 153 and finds all the connected peers and orderers within the MagnetoCorp 154 organization using the [gossip protocol](../gossip.html). If [anchor 155 peers](../glossary.html#anchor-peer) have been defined for a channel, then 156 service discovery will use the gossip protocol across organizations to discover 157 components within the connected organization. This process will also discover 158 smart contracts installed on peers and their endorsement policies defined at a 159 channel level. As with static gateways, the discovered network must be 160 sufficient to get commercial paper transactions endorsed and distributed. 161 162 Dynamic gateways are the default setting for Fabric applications. They can be 163 explicitly specified using the connect option `discovery: { enabled:true }` on 164 the `gateway.connect()` API. Alternatively, the environment variable setting 165 `FABRIC_SDK_DISCOVERY=true` will always override the application choice. 166 167 A dynamic gateway represents an up-to-date view of a network. As networks 168 change, service discovery will ensure that the network view is an accurate 169 reflection of the topology visible to the application. Applications will 170 automatically pick up these changes; they do not even need to re-load the 171 gateway file. 172 173 ## Multiple gateways 174 175 Finally, it is straightforward for an application to define multiple gateways, 176 both for the same or different networks. Moreover, applications can use the name 177 gateway both statically and dynamically. 178 179 It can be helpful to have multiple gateways. Here are a few reasons: 180 181 * Handling requests on behalf of different users. 182 183 * Connecting to different networks simultaneously. 184 185 * Testing a network configuration, by simultaneously comparing its behaviour 186 with an existing configuration. 187 188 <!--- Licensed under Creative Commons Attribution 4.0 International License 189 https://creativecommons.org/licenses/by/4.0/ -->