github.com/true-sqn/fabric@v2.1.1+incompatible/docs/source/developapps/wallet.md (about) 1 # Wallet 2 3 **Audience**: Architects, application and smart contract developers 4 5 A wallet contains a set of user identities. An application run by a user selects 6 one of these identities when it connects to a channel. Access rights to channel 7 resources, such as the ledger, are determined using this identity in combination 8 with an MSP. 9 10 In this topic, we're going to cover: 11 12 * [Why wallets are important](#scenario) 13 * [How wallets are organized](#structure) 14 * [Different types of wallet](#types) 15 * [Wallet operations](#operations) 16 17 ## Scenario 18 19 When an application connects to a network channel such as PaperNet, it selects a 20 user identity to do so, for example `ID1`. The channel MSPs associate `ID1` with 21 a role within a particular organization, and this role will ultimately determine 22 the application's rights over channel resources. For example, `ID1` might 23 identify a user as a member of the MagnetoCorp organization who can read and 24 write to the ledger, whereas `ID2` might identify an administrator in 25 MagnetoCorp who can add a new organization to a consortium. 26 27 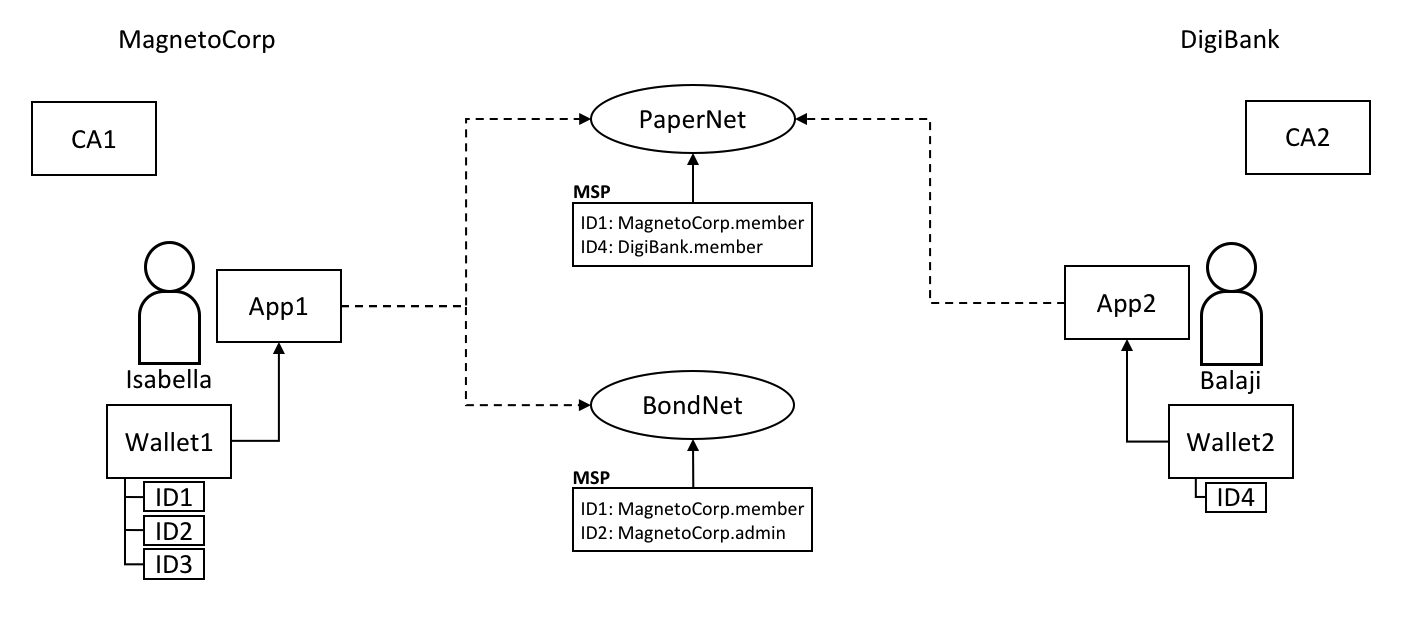 *Two users, Isabella and Balaji 28 have wallets containing different identities they can use to connect to 29 different network channels, PaperNet and BondNet.* 30 31 Consider the example of two users; Isabella from MagnetoCorp and Balaji from 32 DigiBank. Isabella is going to use App 1 to invoke a smart contract in PaperNet 33 and a different smart contract in BondNet. Similarly, Balaji is going to use 34 App 2 to invoke smart contracts, but only in PaperNet. (It's very 35 [easy](./application.html#construct-request) for applications to access multiple 36 networks and multiple smart contracts within them.) 37 38 See how: 39 40 * MagnetoCorp uses CA1 to issue identities and DigiBank uses CA2 to issue 41 identities. These identities are stored in user wallets. 42 43 * Balaji's wallet holds a single identity, `ID4` issued by CA2. Isabella's 44 wallet has many identities, `ID1`, `ID2` and `ID3`, issued by CA1. Wallets 45 can hold multiple identities for a single user, and each identity can be 46 issued by a different CA. 47 48 * Both Isabella and Balaji connect to PaperNet, and its MSPs determine that 49 Isabella is a member of the MagnetoCorp organization, and Balaji is a member 50 of the DigiBank organization, because of the respective CAs that issued their 51 identities. (It is 52 [possible](../membership/membership.html#mapping-msps-to-organizations) for an 53 organization to use multiple CAs, and for a single CA to support multiple 54 organizations.) 55 56 * Isabella can use `ID1` to connect to both PaperNet and BondNet. In both cases, 57 when Isabella uses this identity, she is recognized as a member of 58 MangetoCorp. 59 60 * Isabella can use `ID2` to connect to BondNet, in which case she is identified 61 as an administrator of MagnetoCorp. This gives Isabella two very different 62 privileges: `ID1` identifies her as a simple member of MagnetoCorp who can 63 read and write to the BondNet ledger, whereas `ID2` identities her as a 64 MagnetoCorp administrator who can add a new organization to BondNet. 65 66 * Balaji cannot connect to BondNet with `ID4`. If he tried to connect, `ID4` 67 would not be recognized as belonging to DigiBank because CA2 is not known to 68 BondNet's MSP. 69 70 ## Types 71 72 There are different types of wallets according to where they store their 73 identities: 74 75 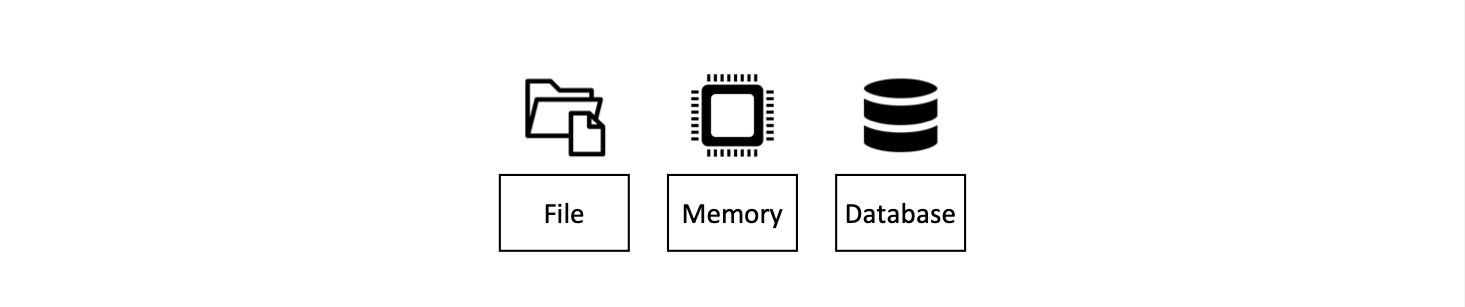 *The three different types of wallet storage: 76 File system, In-memory and CouchDB.* 77 78 * **File system**: This is the most common place to store wallets; file systems 79 are pervasive, easy to understand, and can be network mounted. They are a good 80 default choice for wallets. 81 82 * **In-memory**: A wallet in application storage. Use this type of wallet when 83 your application is running in a constrained environment without access to a 84 file system; typically a web browser. It's worth remembering that this type of 85 wallet is volatile; identities will be lost after the application ends 86 normally or crashes. 87 88 * **CouchDB**: A wallet stored in CouchDB. This is the rarest form of wallet 89 storage, but for those users who want to use the database back-up and restore 90 mechanisms, CouchDB wallets can provide a useful option to simplify disaster 91 recovery. 92 93 Use factory functions provided by the `Wallets` 94 [class](https://hyperledger.github.io/fabric-sdk-node/{BRANCH}/module-fabric-network.Wallets.html) 95 to create wallets. 96 97 ### Hardware Security Module 98 99 A Hardware Security Module (HSM) is an ultra-secure, tamper-proof device that 100 stores digital identity information, particularly private keys. HSMs can be 101 locally attached to your computer or network accessible. Most HSMs provide the 102 ability to perform on-board encryption with private keys, such that the private 103 keys never leave the HSM. 104 105 An HSM can be used with any of the wallet types. In this case the certificate 106 for an identity will be stored in the wallet and the private key will be stored 107 in the HSM. 108 109 To enable the use of HSM-managed identities, an `IdentityProvider` must be 110 configured with the HSM connection information and registered with the wallet. 111 For further details, refer to the [Using wallets to manage identities](https://hyperledger.github.io/fabric-sdk-node/{BRANCH}/tutorial-wallet.html) tutorial. 112 113 ## Structure 114 115 A single wallet can hold multiple identities, each issued by a particular 116 Certificate Authority. Each identity has a standard structure comprising a 117 descriptive label, an X.509 certificate containing a public key, a private key, 118 and some Fabric-specific metadata. Different [wallet types](#types) map this 119 structure appropriately to their storage mechanism. 120 121 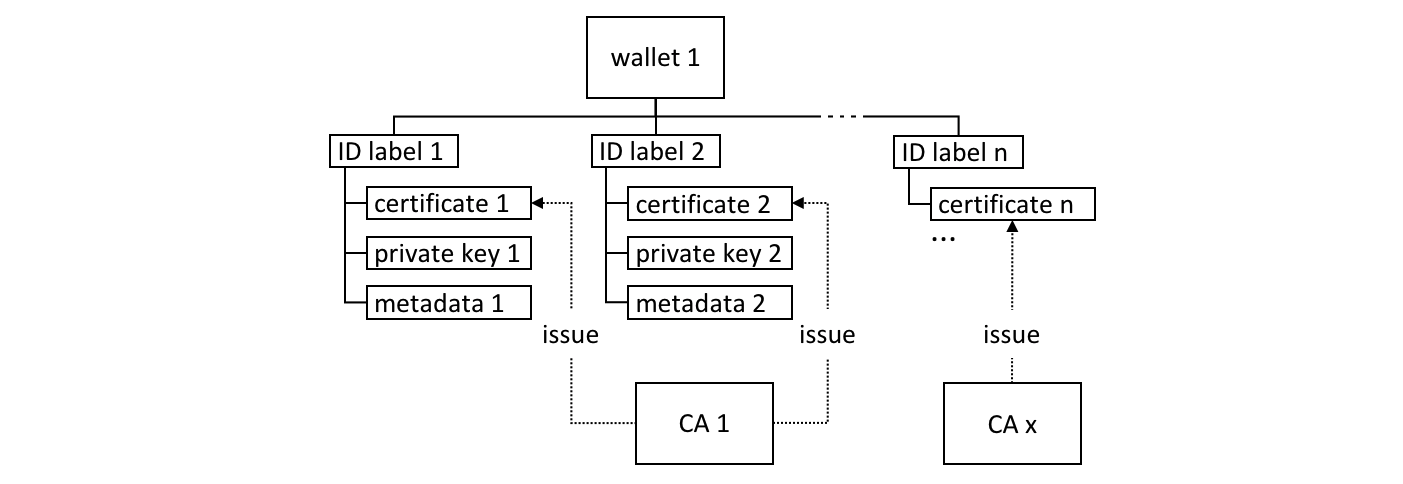 *A Fabric wallet can hold multiple 122 identities with certificates issued by a different Certificate Authority. 123 Identities comprise certificate, private key and Fabric metadata.* 124 125 There's a couple of key class methods that make it easy to manage wallets and 126 identities: 127 128 ```JavaScript 129 const identity: X509Identity = { 130 credentials: { 131 certificate: certificatePEM, 132 privateKey: privateKeyPEM, 133 }, 134 mspId: 'Org1MSP', 135 type: 'X.509', 136 }; 137 await wallet.put(identityLabel, identity); 138 ``` 139 140 See how an `identity` is created that has metadata `Org1MSP`, a `certificate` and 141 a `privateKey`. See how `wallet.put()` adds this identity to the wallet with a 142 particular `identityLabel`. 143 144 The `Gateway` class only requires the `mspId` and `type` metadata to be set for 145 an identity -- `Org1MSP` and `X.509` in the above example. It *currently* uses the 146 MSP ID value to identify particular peers from a [connection profile](./connectionprofile.html), 147 for example when a specific notification [strategy](./connectoptions.html) is 148 requested. In the DigiBank gateway file `networkConnection.yaml`, see how 149 `Org1MSP` notifications will be associated with `peer0.org1.example.com`: 150 151 ```yaml 152 organizations: 153 Org1: 154 mspid: Org1MSP 155 156 peers: 157 - peer0.org1.example.com 158 ``` 159 160 You really don't need to worry about the internal structure of the different 161 wallet types, but if you're interested, navigate to a user identity folder in 162 the commercial paper sample: 163 164 ``` 165 magnetocorp/identity/user/isabella/ 166 wallet/ 167 User1@org1.example.com.id 168 ``` 169 170 You can examine these files, but as discussed, it's easier to use the SDK to 171 manipulate these data. 172 173 ## Operations 174 175 The different wallet types all implement a common 176 [Wallet](https://hyperledger.github.io/fabric-sdk-node/{BRANCH}/module-fabric-network.Wallet.html) 177 interface which provides a standard set of APIs to manage identities. It means 178 that applications can be made independent of the underlying wallet storage 179 mechanism; for example, File system and HSM wallets are handled in a very 180 similar way. 181 182 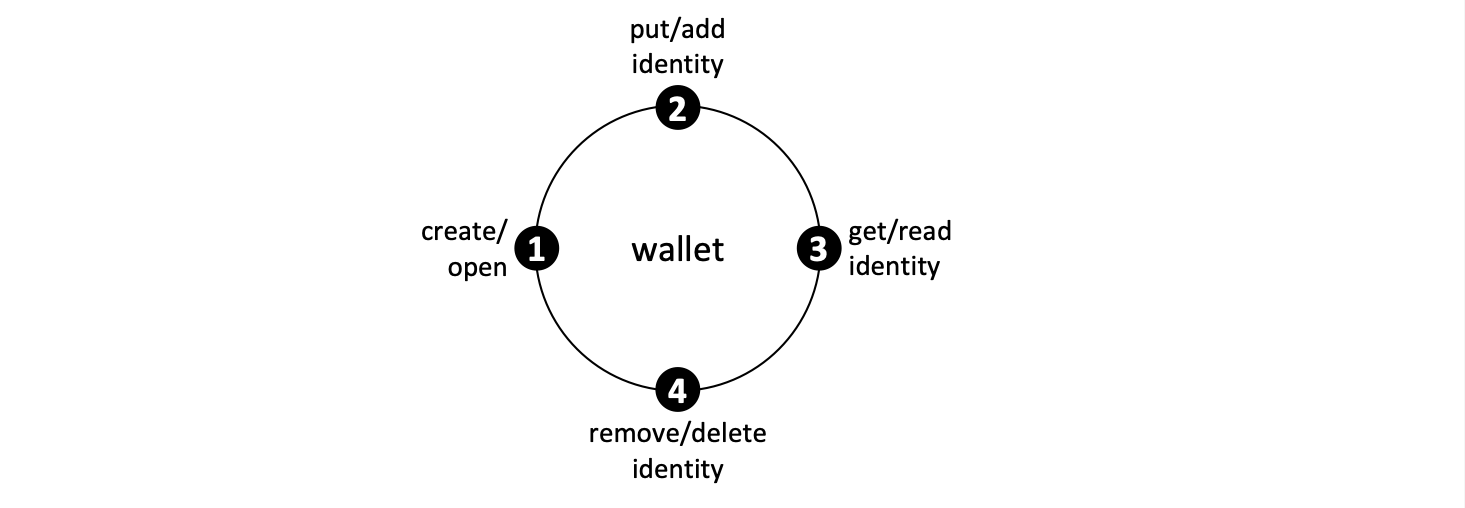 *Wallets follow a 183 lifecycle: they can be created or opened, and identities can be read, added and 184 deleted.* 185 186 An application can use a wallet according to a simple lifecycle. Wallets can be 187 opened or created, and subsequently identities can be added, updated, read and 188 deleted. Spend a little time on the different `Wallet` methods in the 189 [JSDoc](https://hyperledger.github.io/fabric-sdk-node/{BRANCH}/module-fabric-network.Wallet.html) 190 to see how they work; the commercial paper tutorial provides a nice example in 191 `addToWallet.js`: 192 193 ```JavaScript 194 const wallet = await Wallets.newFileSystemWallet('../identity/user/isabella/wallet'); 195 196 const cert = fs.readFileSync(path.join(credPath, '.../User1@org1.example.com-cert.pem')).toString(); 197 const key = fs.readFileSync(path.join(credPath, '.../_sk')).toString(); 198 199 const identityLabel = 'User1@org1.example.com'; 200 const identity = { 201 credentials: { 202 certificate: cert, 203 privateKey: key, 204 }, 205 mspId: 'Org1MSP', 206 type: 'X.509', 207 }; 208 209 await wallet.put(identityLabel, identity); 210 ``` 211 212 Notice how: 213 214 * When the program is first run, a wallet is created on the local file system at 215 `.../isabella/wallet`. 216 217 * a certificate `cert` and private `key` are loaded from the file system. 218 219 * a new X.509 identity is created with `cert`, `key` and `Org1MSP`. 220 221 * the new identity is added to the wallet with `wallet.put()` with a label 222 `User1@org1.example.com`. 223 224 That's everything you need to know about wallets. You've seen how they hold 225 identities that are used by applications on behalf of users to access Fabric 226 network resources. There are different types of wallets available depending on 227 your application and security needs, and a simple set of APIs to help 228 applications manage wallets and the identities within them. 229 230 <!--- Licensed under Creative Commons Attribution 4.0 International License 231 https://creativecommons.org/licenses/by/4.0/ -->