github.com/true-sqn/fabric@v2.1.1+incompatible/docs/source/smartcontract/smartcontract.md (about) 1 # Smart Contracts and Chaincode 2 3 **Audience**: Architects, application and smart contract developers, 4 administrators 5 6 From an application developer's perspective, a **smart contract**, together with 7 the [ledger](../ledger/ledger.html), form the heart of a Hyperledger Fabric 8 blockchain system. Whereas a ledger holds facts about the current and historical 9 state of a set of business objects, a **smart contract** defines the executable 10 logic that generates new facts that are added to the ledger. A **chaincode** 11 is typically used by administrators to group related smart contracts for 12 deployment, but can also be used for low level system programming of Fabric. In 13 this topic, we'll focus on why both **smart contracts** and **chaincode** exist, 14 and how and when to use them. 15 16 In this topic, we'll cover: 17 18 * [What is a smart contract](#smart-contract) 19 * [A note on terminology](#terminology) 20 * [Smart contracts and the ledger](#ledger) 21 * [How to develop a smart contract](#developing) 22 * [The importance of endorsement policies](#endorsement) 23 * [Valid transactions](#valid-transactions) 24 * [Channels and chaincode definitions](#channels) 25 * [Communicating between smart contracts](#intercommunication) 26 * [What is system chaincode?](#system-chaincode) 27 28 ## Smart contract 29 30 Before businesses can transact with each other, they must define a common set of 31 contracts covering common terms, data, rules, concept definitions, and 32 processes. Taken together, these contracts lay out the **business model** that 33 govern all of the interactions between transacting parties. 34 35 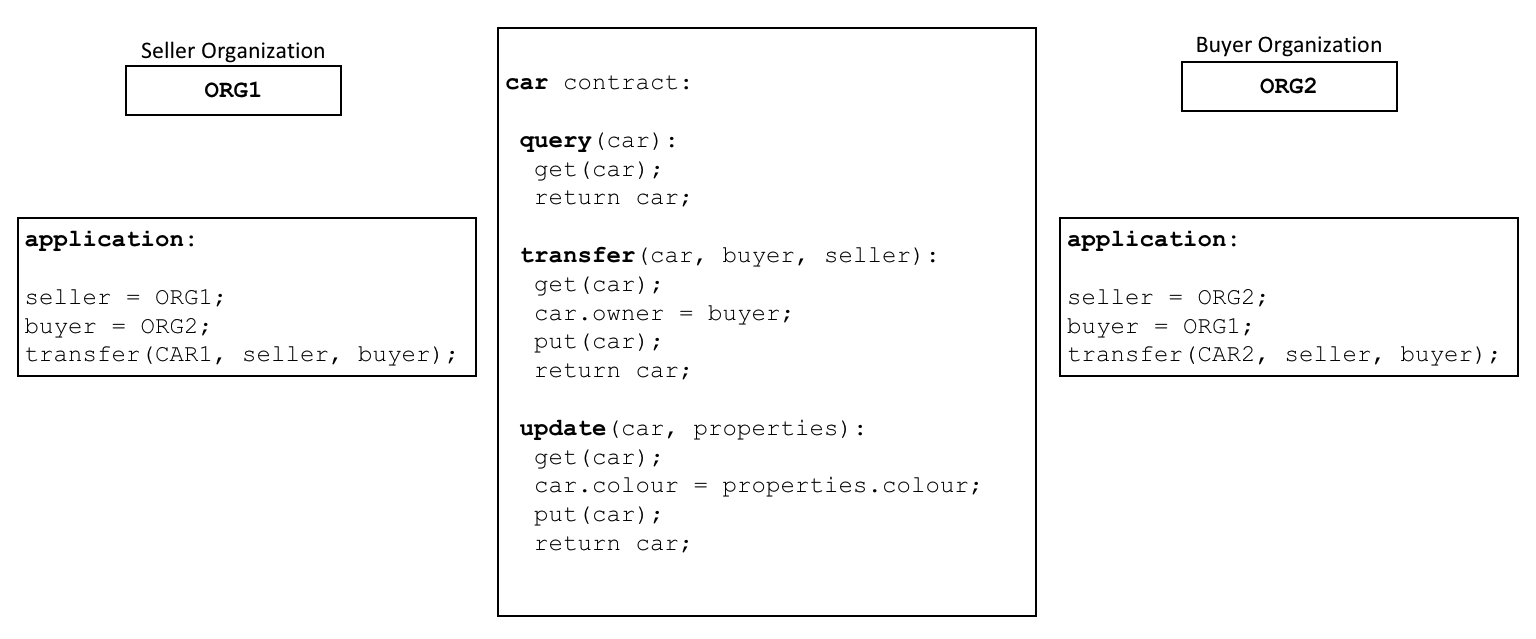 *A smart contract defines the 36 rules between different organizations in executable code. Applications invoke a 37 smart contract to generate transactions that are recorded on the ledger.* 38 39 Using a blockchain network, we can turn these contracts into executable programs 40 -- known in the industry as **smart contracts** -- to open up a wide variety of 41 new possibilities. That's because a smart contract can implement the governance 42 rules for **any** type of business object, so that they can be automatically 43 enforced when the smart contract is executed. For example, a smart contract 44 might ensure that a new car delivery is made within a specified timeframe, or 45 that funds are released according to prearranged terms, improving the flow of 46 goods or capital respectively. Most importantly however, the execution of a 47 smart contract is much more efficient than a manual human business process. 48 49 In the [diagram above](#smart-contract), we can see how two organizations, 50 `ORG1` and `ORG2` have defined a `car` smart contract to `query`, `transfer` and 51 `update` cars. Applications from these organizations invoke this smart contract 52 to perform an agreed step in a business process, for example to transfer 53 ownership of a specific car from `ORG1` to `ORG2`. 54 55 56 ## Terminology 57 58 Hyperledger Fabric users often use the terms **smart contract** and 59 **chaincode** interchangeably. In general, a smart contract defines the 60 **transaction logic** that controls the lifecycle of a business object contained 61 in the world state. It is then packaged into a chaincode which is then deployed 62 to a blockchain network. Think of smart contracts as governing transactions, 63 whereas chaincode governs how smart contracts are packaged for deployment. 64 65 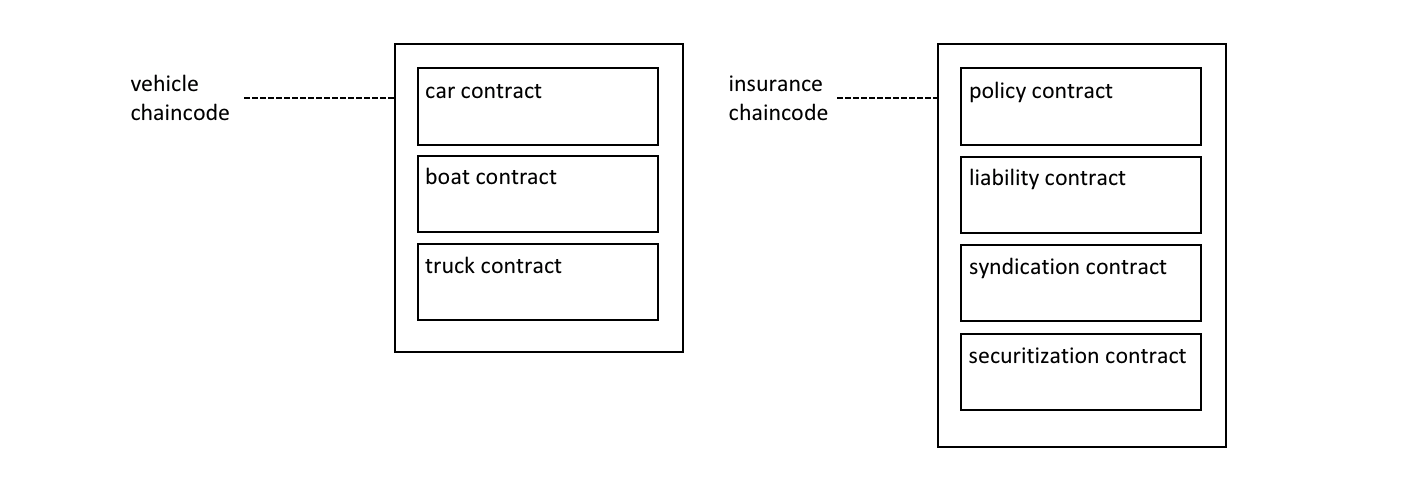 *A smart contract is defined 66 within a chaincode. Multiple smart contracts can be defined within the same 67 chaincode. When a chaincode is deployed, all smart contracts within it are made 68 available to applications.* 69 70 In the diagram, we can see a `vehicle` chaincode that contains three smart 71 contracts: `cars`, `boats` and `trucks`. We can also see an `insurance` 72 chaincode that contains four smart contracts: `policy`, `liability`, 73 `syndication` and `securitization`. In both cases these contracts cover key 74 aspects of the business process relating to vehicles and insurance. In this 75 topic, we will use the `car` contract as an example. We can see that a smart 76 contract is a domain specific program which relates to specific business 77 processes, whereas a chaincode is a technical container of a group of related 78 smart contracts. 79 80 81 ## Ledger 82 83 At the simplest level, a blockchain immutably records transactions which update 84 states in a ledger. A smart contract programmatically accesses two distinct 85 pieces of the ledger -- a **blockchain**, which immutably records the history of 86 all transactions, and a **world state** that holds a cache of the current value 87 of these states, as it's the current value of an object that is usually 88 required. 89 90 Smart contracts primarily **put**, **get** and **delete** states in the world 91 state, and can also query the immutable blockchain record of transactions. 92 93 * A **get** typically represents a query to retrieve information about the 94 current state of a business object. 95 * A **put** typically creates a new business object or modifies an existing one 96 in the ledger world state. 97 * A **delete** typically represents the removal of a business object from the 98 current state of the ledger, but not its history. 99 100 Smart contracts have many 101 [APIs](../developapps/transactioncontext.html#structure) available to them. 102 Critically, in all cases, whether transactions create, read, update or delete 103 business objects in the world state, the blockchain contains an [immutable 104 record](../ledger/ledger.html) of these changes. 105 106 ## Development 107 108 Smart contracts are the focus of application development, and as we've seen, one 109 or more smart contracts can be defined within a single chaincode. Deploying a 110 chaincode to a network makes all its smart contracts available to the 111 organizations in that network. It means that only administrators need to worry 112 about chaincode; everyone else can think in terms of smart contracts. 113 114 At the heart of a smart contract is a set of `transaction` definitions. For 115 example, look at 116 [`fabcar.js`](https://github.com/hyperledger/fabric-samples/blob/{BRANCH}/chaincode/fabcar/javascript/lib/fabcar.js#L93), 117 where you can see a smart contract transaction that creates a new car: 118 119 ```javascript 120 async createCar(ctx, carNumber, make, model, color, owner) { 121 122 const car = { 123 color, 124 docType: 'car', 125 make, 126 model, 127 owner, 128 }; 129 130 await ctx.stub.putState(carNumber, Buffer.from(JSON.stringify(car))); 131 } 132 ``` 133 134 You can learn more about the **Fabcar** smart contract in the [Writing your 135 first application](../write_first_app.html) tutorial. 136 137 A smart contract can describe an almost infinite array of business use cases 138 relating to immutability of data in multi-organizational decision making. The 139 job of a smart contract developer is to take an existing business process that 140 might govern financial prices or delivery conditions, and express it as 141 a smart contract in a programming language such as JavaScript, Go, or Java. 142 The legal and technical skills required to convert centuries of legal language 143 into programming language is increasingly practiced by **smart contract 144 auditors**. You can learn about how to design and develop a smart contract in 145 the [Developing applications 146 topic](../developapps/developing_applications.html). 147 148 149 ## Endorsement 150 151 Associated with every chaincode is an endorsement policy that applies to all of 152 the smart contracts defined within it. An endorsement policy is very important; 153 it indicates which organizations in a blockchain network must sign a transaction 154 generated by a given smart contract in order for that transaction to be declared 155 **valid**. 156 157 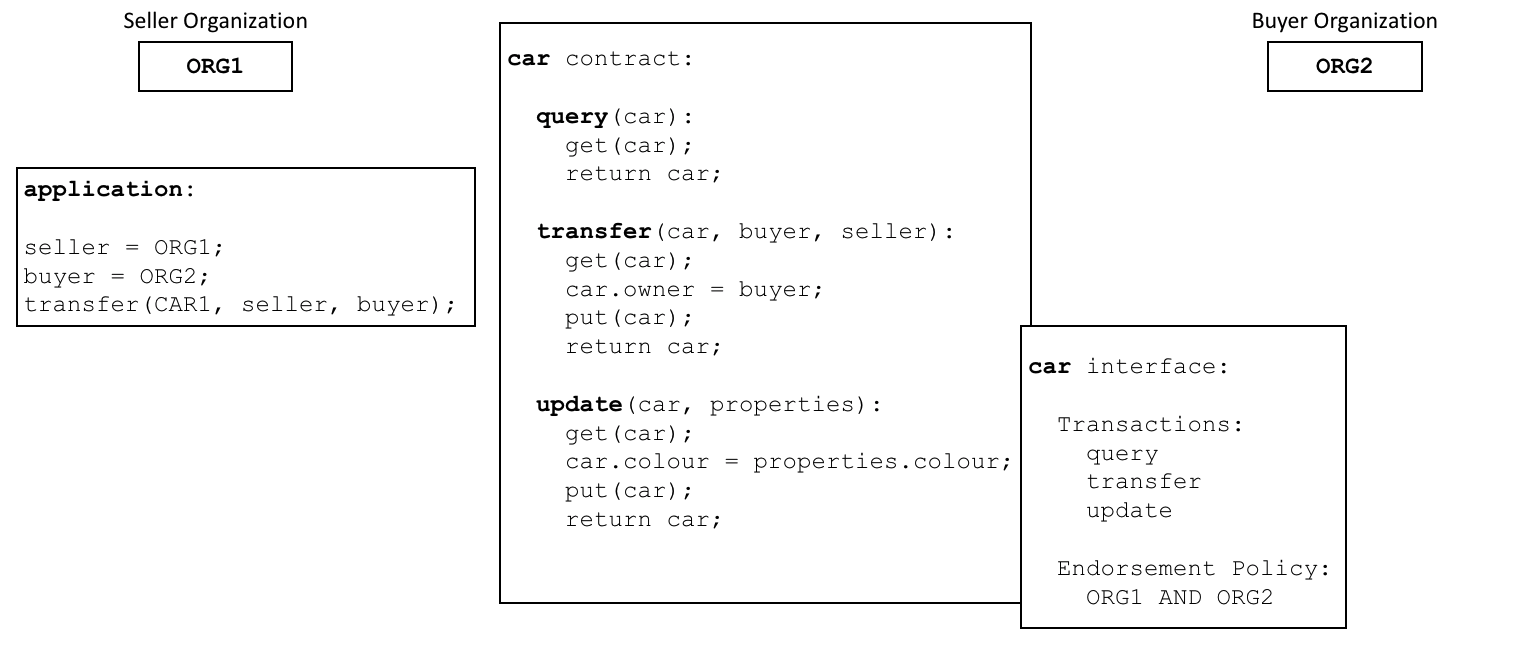 *Every smart contract has an 158 endorsement policy associated with it. This endorsement policy identifies which 159 organizations must approve transactions generated by the smart contract before 160 those transactions can be identified as valid.* 161 162 An example endorsement policy might define that three of the four organizations 163 participating in a blockchain network must sign a transaction before it is 164 considered **valid**. All transactions, whether **valid** or **invalid** are 165 added to a distributed ledger, but only **valid** transactions update the world 166 state. 167 168 If an endorsement policy specifies that more than one organization must sign a 169 transaction, then the smart contract must be executed by a sufficient set of 170 organizations in order for a valid transaction to be generated. In the example 171 [above](#endorsement), a smart contract transaction to `transfer` a car would 172 need to be executed and signed by both `ORG1` and `ORG2` for it to be valid. 173 174 Endorsement policies are what make Hyperledger Fabric different to other 175 blockchains like Ethereum or Bitcoin. In these systems valid transactions can be 176 generated by any node in the network. Hyperledger Fabric more realistically 177 models the real world; transactions must be validated by trusted organizations 178 in a network. For example, a government organization must sign a valid 179 `issueIdentity` transaction, or both the `buyer` and `seller` of a car must sign 180 a `car` transfer transaction. Endorsement policies are designed to allow 181 Hyperledger Fabric to better model these types of real-world interactions. 182 183 Finally, endorsement policies are just one example of 184 [policy](../access_control.html#policies) in Hyperledger Fabric. Other policies 185 can be defined to identify who can query or update the ledger, or add or remove 186 participants from the network. In general, policies should be agreed in advance 187 by the consortium of organizations in a blockchain network, although they are 188 not set in stone. Indeed, policies themselves can define the rules by which they 189 can be changed. And although an advanced topic, it is also possible to define 190 [custom endorsement policy](../pluggable_endorsement_and_validation.html) rules 191 over and above those provided by Fabric. 192 193 ## Valid transactions 194 195 When a smart contract executes, it runs on a peer node owned by an organization 196 in the blockchain network. The contract takes a set of input parameters called 197 the **transaction proposal** and uses them in combination with its program logic 198 to read and write the ledger. Changes to the world state are captured as a 199 **transaction proposal response** (or just **transaction response**) which 200 contains a **read-write set** with both the states that have been read, and the 201 new states that are to be written if the transaction is valid. Notice that the 202 world state **is not updated when the smart contract is executed**! 203 204 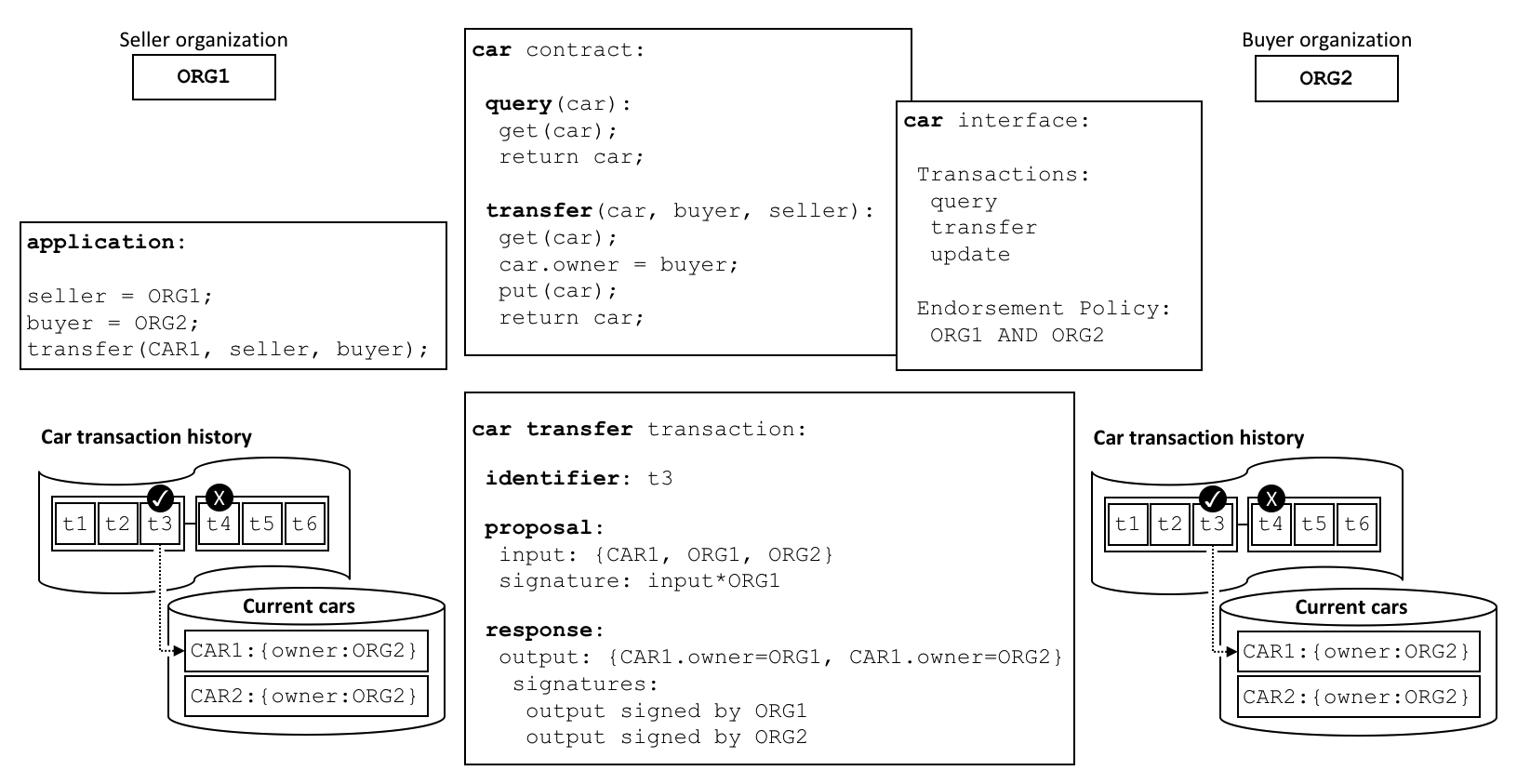 *All transactions have an 205 identifier, a proposal, and a response signed by a set of organizations. All 206 transactions are recorded on the blockchain, whether valid or invalid, but only 207 valid transactions contribute to the world state.* 208 209 Examine the `car transfer` transaction. You can see a transaction `t3` for a car 210 transfer between `ORG1` and `ORG2`. See how the transaction has input `{CAR1, 211 ORG1, ORG2}` and output `{CAR1.owner=ORG1, CAR1.owner=ORG2}`, representing the 212 change of owner from `ORG1` to `ORG2`. Notice how the input is signed by the 213 application's organization `ORG1`, and the output is signed by *both* 214 organizations identified by the endorsement policy, `ORG1` and `ORG2`. These 215 signatures were generated by using each actor's private key, and mean that 216 anyone in the network can verify that all actors in the network are in agreement 217 about the transaction details. 218 219 A transaction that is distributed to all peer nodes in the network is 220 **validated** in two phases by each peer. Firstly, the transaction is checked to 221 ensure it has been signed by sufficient organizations according to the endorsement 222 policy. Secondly, it is checked to ensure that the current value of the world state 223 matches the read set of the transaction when it was signed by the endorsing peer 224 nodes; that there has been no intermediate update. If a transaction passes both 225 these tests, it is marked as **valid**. All transactions are added to the 226 blockchain history, whether **valid** or **invalid**, but only **valid** 227 transactions result in an update to the world state. 228 229 In our example, `t3` is a valid transaction, so the owner of `CAR1` has been 230 updated to `ORG2`. However, `t4` (not shown) is an invalid transaction, so while 231 it was recorded in the ledger, the world state was not updated, and `CAR2` 232 remains owned by `ORG2`. 233 234 Finally, to understand how to use a smart contract or chaincode with world 235 state, read the [chaincode namespace 236 topic](../developapps/chaincodenamespace.html). 237 238 ## Channels 239 240 Hyperledger Fabric allows an organization to simultaneously participate in 241 multiple, separate blockchain networks via **channels**. By joining multiple 242 channels, an organization can participate in a so-called **network of networks**. 243 Channels provide an efficient sharing of infrastructure while maintaining data 244 and communications privacy. They are independent enough to help organizations 245 separate their work traffic with different counterparties, but integrated enough 246 to allow them to coordinate independent activities when necessary. 247 248 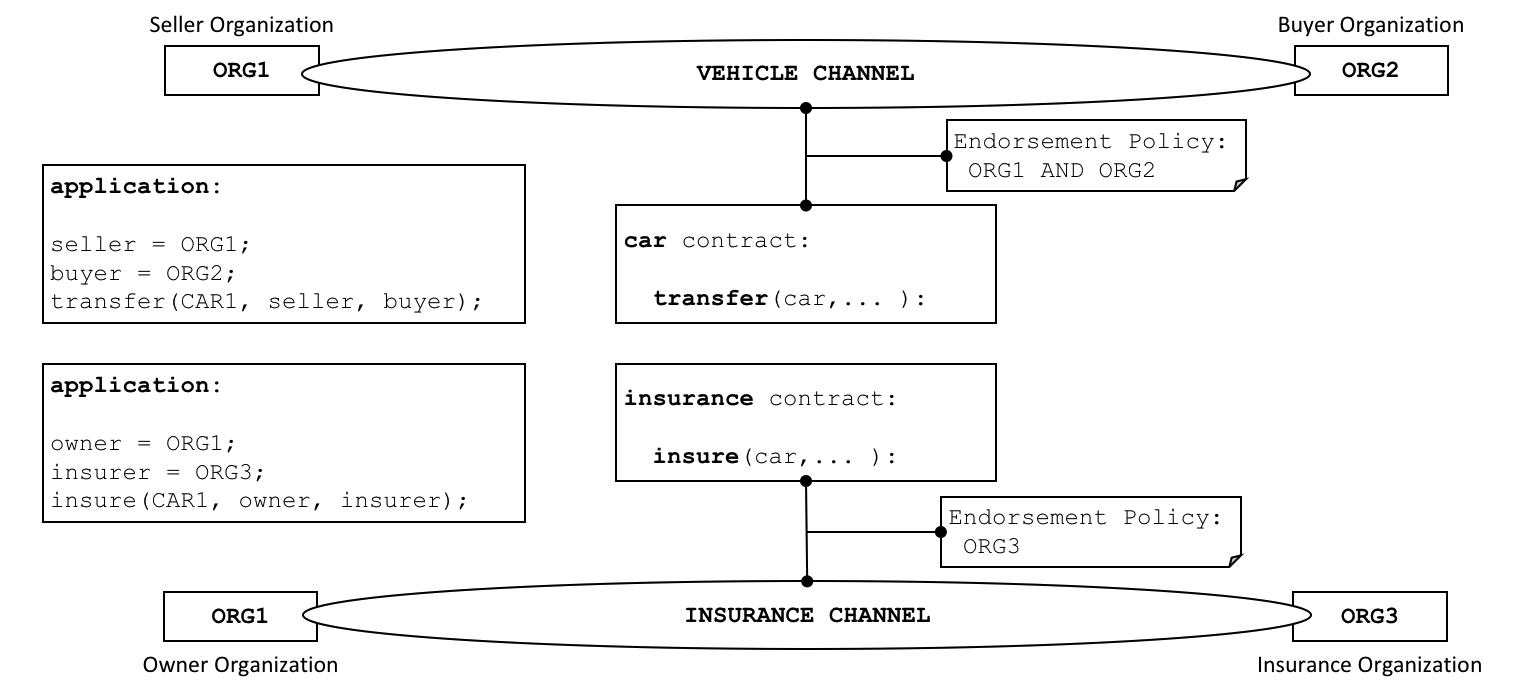 *A channel provides a 249 completely separate communication mechanism between a set of organizations. When 250 a chaincode definition is committed to a channel, all the smart contracts within 251 the chaincode are made available to the applications on that channel.* 252 253 While the smart contract code is installed inside a chaincode package on an 254 organizations peers, channel members can only execute a smart contract after 255 the chaincode has been defined on a channel. The **chaincode definition** is a 256 struct that contains the parameters that govern how a chaincode operates. These 257 parameters include the chaincode name, version, and the endorsement policy. 258 Each channel member agrees to the parameters of a chaincode by approving a 259 chaincode definition for their organization. When a sufficient number of 260 organizations (a majority by default) have approved to the same chaincode 261 definition, the definition can be committed to the channel. The smart contracts 262 inside the chaincode can then be executed by channel members, subject to the 263 endorsement policy specified in the chaincode definition. The endorsement policy 264 applies equally to all smart contracts defined within the same chaincode. 265 266 In the example [above](#channels), a `car` contract is defined on the `VEHICLE` 267 channel, and an `insurance` contract is defined on the `INSURANCE` channel. 268 The chaincode definition of `car` specifies an endorsement policy that requires 269 both `ORG1` and `ORG2` to sign transactions before they can be considered valid. 270 The chaincode definition of the `insurance` contract specifies that only `ORG3` 271 is required to endorse a transaction. `ORG1` participates in two networks, the 272 `VEHICLE` channel and the `INSURANCE` network, and can coordinate activity with 273 `ORG2` and `ORG3` across these two networks. 274 275 The chaincode definition provides a way for channel members to agree on the 276 governance of a chaincode before they start using the smart contract to 277 transact on the channel. Building on the example above, both `ORG1` and `ORG2` 278 want to endorse transactions that invoke the `car` contract. Because the default 279 policy requires that a majority of organizations approve a chaincode definition, 280 both organizations need to approve an endorsement policy of `AND{ORG1,ORG2}`. 281 Otherwise, `ORG1` and `ORG2` would approve different chaincode definitions and 282 would be unable to commit the chaincode definition to the channel as a result. 283 This process guarantees that a transaction from the `car` smart contract needs 284 to be approved by both organizations. 285 286 ## Intercommunication 287 288 A Smart Contract can call other smart contracts both within the same 289 channel and across different channels. It this way, they can read and write 290 world state data to which they would not otherwise have access due to smart 291 contract namespaces. 292 293 There are limitations to this inter-contract communication, which are described 294 fully in the [chaincode namespace](../developapps/chaincodenamespace.html#cross-chaincode-access) topic. 295 296 ## System chaincode 297 298 The smart contracts defined within a chaincode encode the domain dependent rules 299 for a business process agreed between a set of blockchain organizations. 300 However, a chaincode can also define low-level program code which corresponds to 301 domain independent *system* interactions, unrelated to these smart contracts 302 for business processes. 303 304 The following are the different types of system chaincodes and their associated 305 abbreviations: 306 307 * `_lifecycle` runs in all peers and manages the installation of chaincode on 308 your peers, the approval of chaincode definitions for your organization, and 309 the committing of chaincode definitions to channels. You can read more about 310 how `_lifecycle` implements the Fabric chaincode lifecycle [process](../chaincode_lifecycle.html). 311 312 * Lifecycle system chaincode (LSCC) manages the chaincode lifecycle for the 313 1.x releases of Fabric. This version of lifecycle required that chaincode be 314 instantiated or upgraded on channels. You can still use LSCC to manage your 315 chaincode if you have the channel application capability set to V1_4_x or below. 316 317 * **Configuration system chaincode (CSCC)** runs in all peers to handle changes to a 318 channel configuration, such as a policy update. You can read more about this 319 process in the following chaincode 320 [topic](../configtx.html#configuration-updates). 321 322 * **Query system chaincode (QSCC)** runs in all peers to provide ledger APIs which 323 include block query, transaction query etc. You can read more about these 324 ledger APIs in the transaction context 325 [topic](../developapps/transactioncontext.html). 326 327 * **Endorsement system chaincode (ESCC)** runs in endorsing peers to 328 cryptographically sign a transaction response. You can read more about how 329 the ESCC implements this [process](../peers/peers.html#phase-1-proposal). 330 331 * **Validation system chaincode (VSCC)** validates a transaction, including checking 332 endorsement policy and read-write set versioning. You can read more about the 333 VSCC implements this [process](../peers/peers.html#phase-3-validation). 334 335 It is possible for low level Fabric developers and administrators to modify 336 these system chaincodes for their own uses. However, the development and 337 management of system chaincodes is a specialized activity, quite separate from 338 the development of smart contracts, and is not normally necessary. Changes to 339 system chaincodes must be handled with extreme care as they are fundamental to 340 the correct functioning of a Hyperledger Fabric network. For example, if a 341 system chaincode is not developed correctly, one peer node may update its copy 342 of the world state or blockchain differently compared to another peer node. This 343 lack of consensus is one form of a **ledger fork**, a very undesirable situation. 344 345 <!--- Licensed under Creative Commons Attribution 4.0 International License 346 https://creativecommons.org/licenses/by/4.0/ -->