github.com/vipernet-xyz/tendermint-core@v0.32.0/CONTRIBUTING.md (about) 1 # Contributing 2 3 Thank you for your interest in contributing to Tendermint! Before 4 contributing, it may be helpful to understand the goal of the project. The goal 5 of Tendermint is to develop a BFT consensus engine robust enough to 6 support permissionless value-carrying networks. While all contributions are 7 welcome, contributors should bear this goal in mind in deciding if they should 8 target the main tendermint project or a potential fork. When targeting the 9 main Tendermint project, the following process leads to the best chance of 10 landing changes in master. 11 12 All work on the code base should be motivated by a [Github 13 Issue](https://github.com/tendermint/tendermint/issues). 14 [Search](https://github.com/tendermint/tendermint/issues?q=is%3Aopen+is%3Aissue+label%3A%22help+wanted%22) 15 is a good place start when looking for places to contribute. If you 16 would like to work on an issue which already exists, please indicate so 17 by leaving a comment. 18 19 All new contributions should start with a [Github 20 Issue](https://github.com/tendermint/tendermint/issues/new/choose). The 21 issue helps capture the problem you're trying to solve and allows for 22 early feedback. Once the issue is created the process can proceed in different 23 directions depending on how well defined the problem and potential 24 solution are. If the change is simple and well understood, maintainers 25 will indicate their support with a heartfelt emoji. 26 27 If the issue would benefit from thorough discussion, maintainers may 28 request that you create a [Request For 29 Comment](https://github.com/tendermint/spec/tree/master/rfc). Discussion 30 at the RFC stage will build collective understanding of the dimensions 31 of the problems and help structure conversations around trade-offs. 32 33 When the problem is well understood but the solution leads to large 34 structural changes to the code base, these changes should be proposed in 35 the form of an [Architectural Decision Record 36 (ADR)](./docs/architecture/). The ADR will help build consensus on an 37 overall strategy to ensure the code base maintains coherence 38 in the larger context. If you are not comfortable with writing an ADR, 39 you can open a less-formal issue and the maintainers will help you 40 turn it into an ADR. ADR numbers can be registered [here](https://github.com/tendermint/tendermint/issues/2313). 41 42 When the problem as well as proposed solution are well understood, 43 changes should start with a [draft 44 pull request](https://github.blog/2019-02-14-introducing-draft-pull-requests/) 45 against master. The draft signals that work is underway. When the work 46 is ready for feedback, hitting "Ready for Review" will signal to the 47 maintainers to take a look. 48 49 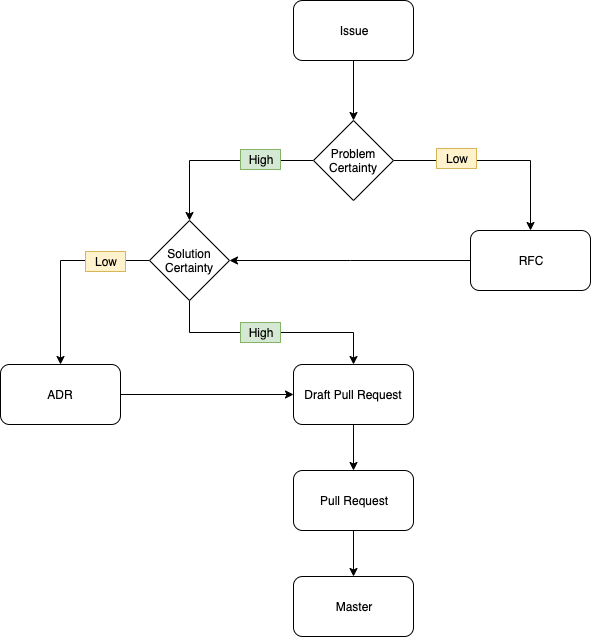 50 51 Each stage of the process is aimed at creating feedback cycles which align contributors and maintainers to make sure: 52 53 - Contributors don’t waste their time implementing/proposing features which won’t land in master. 54 - Maintainers have the necessary context in order to support and review contributions. 55 56 ## Forking 57 58 Please note that Go requires code to live under absolute paths, which complicates forking. 59 While my fork lives at `https://github.com/ebuchman/tendermint`, 60 the code should never exist at `$GOPATH/src/github.com/ebuchman/tendermint`. 61 Instead, we use `git remote` to add the fork as a new remote for the original repo, 62 `$GOPATH/src/github.com/tendermint/tendermint`, and do all the work there. 63 64 For instance, to create a fork and work on a branch of it, I would: 65 66 - Create the fork on github, using the fork button. 67 - Go to the original repo checked out locally (i.e. `$GOPATH/src/github.com/tendermint/tendermint`) 68 - `git remote rename origin upstream` 69 - `git remote add origin git@github.com:ebuchman/basecoin.git` 70 71 Now `origin` refers to my fork and `upstream` refers to the tendermint version. 72 So I can `git push -u origin master` to update my fork, and make pull requests to tendermint from there. 73 Of course, replace `ebuchman` with your git handle. 74 75 To pull in updates from the origin repo, run 76 77 - `git fetch upstream` 78 - `git rebase upstream/master` (or whatever branch you want) 79 80 ## Dependencies 81 82 We use [go modules](https://github.com/golang/go/wiki/Modules) to manage dependencies. 83 84 That said, the master branch of every Tendermint repository should just build 85 with `go get`, which means they should be kept up-to-date with their 86 dependencies so we can get away with telling people they can just `go get` our 87 software. 88 89 Since some dependencies are not under our control, a third party may break our 90 build, in which case we can fall back on `go mod tidy`. Even for dependencies under our control, go helps us to 91 keep multiple repos in sync as they evolve. Anything with an executable, such 92 as apps, tools, and the core, should use dep. 93 94 Run `go list -u -m all` to get a list of dependencies that may not be 95 up-to-date. 96 97 When updating dependencies, please only update the particular dependencies you 98 need. Instead of running `go get -u=patch`, which will update anything, 99 specify exactly the dependency you want to update, eg. 100 `GO111MODULE=on go get -u github.com/tendermint/go-amino@master`. 101 102 ## Protobuf 103 104 We use [Protocol Buffers](https://developers.google.com/protocol-buffers) along with [gogoproto](https://github.com/gogo/protobuf) to generate code for use across Tendermint Core. 105 106 For linting and checking breaking changes, we use [buf](https://buf.build/). If you would like to run linting and check if the changes you have made are breaking then you will need to have docker running locally. Then the linting cmd will be `make proto-lint` and the breaking changes check will be `make proto-check-breaking`. 107 108 There are two ways to generate your proto stubs. 109 110 1. Use Docker, pull an image that will generate your proto stubs with no need to install anything. `make proto-gen-docker` 111 2. Run `make proto-gen` after installing `protoc` and gogoproto. 112 113 ### Installation Instructions 114 115 To install `protoc`, download an appropriate release (https://github.com/protocolbuffers/protobuf) and then move the provided binaries into your PATH (follow instructions in README included with the download). 116 117 To install `gogoproto`, do the following: 118 119 ```sh 120 $ go get github.com/gogo/protobuf/gogoproto 121 $ cd $GOPATH/pkg/mod/github.com/gogo/protobuf@v1.3.1 # or wherever go get installs things 122 $ make install 123 ``` 124 125 You should now be able to run `make proto-gen` from inside the root Tendermint directory to generate new files from proto files. 126 127 ## Vagrant 128 129 If you are a [Vagrant](https://www.vagrantup.com/) user, you can get started 130 hacking Tendermint with the commands below. 131 132 NOTE: In case you installed Vagrant in 2017, you might need to run 133 `vagrant box update` to upgrade to the latest `ubuntu/xenial64`. 134 135 ``` 136 vagrant up 137 vagrant ssh 138 make test 139 ``` 140 141 ## Changelog 142 143 Every fix, improvement, feature, or breaking change should be made in a 144 pull-request that includes an update to the `CHANGELOG_PENDING.md` file. 145 146 Changelog entries should be formatted as follows: 147 148 ``` 149 - [module] \#xxx Some description about the change (@contributor) 150 ``` 151 152 Here, `module` is the part of the code that changed (typically a 153 top-level Go package), `xxx` is the pull-request number, and `contributor` 154 is the author/s of the change. 155 156 It's also acceptable for `xxx` to refer to the relevent issue number, but pull-request 157 numbers are preferred. 158 Note this means pull-requests should be opened first so the changelog can then 159 be updated with the pull-request's number. 160 There is no need to include the full link, as this will be added 161 automatically during release. But please include the backslash and pound, eg. `\#2313`. 162 163 Changelog entries should be ordered alphabetically according to the 164 `module`, and numerically according to the pull-request number. 165 166 Changes with multiple classifications should be doubly included (eg. a bug fix 167 that is also a breaking change should be recorded under both). 168 169 Breaking changes are further subdivided according to the APIs/users they impact. 170 Any change that effects multiple APIs/users should be recorded multiply - for 171 instance, a change to the `Blockchain Protocol` that removes a field from the 172 header should also be recorded under `CLI/RPC/Config` since the field will be 173 removed from the header in rpc responses as well. 174 175 ## Branching Model and Release 176 177 The main development branch is master. 178 179 Every release is maintained in a release branch named `vX.Y.Z`. 180 181 Note all pull requests should be squash merged except for merging to a release branch (named `vX.Y`). This keeps the commit history clean and makes it 182 easy to reference the pull request where a change was introduced. 183 184 ### Development Procedure 185 186 - the latest state of development is on `master` 187 - `master` must never fail `make test` 188 - never --force onto `master` (except when reverting a broken commit, which should seldom happen) 189 - create a development branch either on github.com/tendermint/tendermint, or your fork (using `git remote add origin`) 190 - make changes and update the `CHANGELOG_PENDING.md` to record your change 191 - before submitting a pull request, run `git rebase` on top of the latest `master` 192 193 When you have submitted a pull request label the pull request with either `R:minor`, if the change can be accepted in a minor release, or `R:major`, if the change is meant for a major release. 194 195 ### Pull Merge Procedure 196 197 - ensure pull branch is based on a recent `master` 198 - run `make test` to ensure that all tests pass 199 - [squash](https://stackoverflow.com/questions/5189560/squash-my-last-x-commits-together-using-git) merge pull request 200 - the `unstable` branch may be used to aggregate pull merges before fixing tests 201 202 ### Git Commit Style 203 204 We follow the [Go style guide on commit messages](https://tip.golang.org/doc/contribute.html#commit_messages). Write concise commits that start with the package name and have a description that finishes the sentence "This change modifies Tendermint to...". For example, 205 206 \``` 207 cmd/debug: execute p.Signal only when p is not nil 208 209 [potentially longer description in the body] 210 211 Fixes #nnnn 212 \``` 213 214 Each PR should have one commit once it lands on `master`; this can be accomplished by using the "squash and merge" button on Github. Be sure to edit your commit message, though! 215 216 ### Release Procedure 217 218 #### Major Release 219 220 1. start on `master` 221 2. run integration tests (see `test_integrations` in Makefile) 222 3. prepare release in a pull request against `master` (to be squash merged): 223 - copy `CHANGELOG_PENDING.md` to top of `CHANGELOG.md` 224 - run `python ./scripts/linkify_changelog.py CHANGELOG.md` to add links for 225 all issues 226 - run `bash ./scripts/authors.sh` to get a list of authors since the latest 227 release, and add the github aliases of external contributors to the top of 228 the changelog. To lookup an alias from an email, try `bash ./scripts/authors.sh <email>` 229 - reset the `CHANGELOG_PENDING.md` 230 - bump the appropriate versions in `version.go` 231 4. push your changes with prepared release details to `vX.X` (this will trigger the release `vX.X.0`) 232 5. merge back to master (don't squash merge!) 233 234 #### Minor Release 235 236 Minor releases are done differently from major releases. Minor release pull requests should be labeled with `R:minor` if they are to be included. 237 238 1. Checkout the last major release, `vX.X`. 239 240 - `git checkout vX.X` 241 242 2. Create a release candidate branch off the most recent major release with your upcoming version specified, `rc1/vX.X.x`, and push the branch. 243 244 - `git checkout -b rc1/vX.X.x` 245 - `git push -u origin rc1/vX.X.x` 246 247 3. Create a cherry-picking branch, and make a pull request into the release candidate. 248 249 - `git checkout -b cherry-picks/rc1/vX.X.x` 250 251 - This is for devs to approve the commits that are entering the release candidate. 252 - There may be merge conflicts. 253 254 4. Begin cherry-picking. 255 256 - `git cherry-pick {PR commit from master you wish to cherry pick}` 257 - Fix conflicts 258 - `git cherry-pick --continue` 259 - `git push cherry-picks/rc1/vX.X.x` 260 261 > Once all commits are included and CI/tests have passed, then it is ready for a release. 262 263 5. Create a release branch `release/vX.X.x` off the release candidate branch. 264 265 - `git checkout -b release/vX.X.x` 266 - `git push -u origin release/vX.X.x` 267 > Note this Branch is protected once pushed, you will need admin help to make any change merges into the branch. 268 269 6. Merge Commit the release branch into the latest major release branch `vX.X`, this will start the release process. 270 271 7. Create a Pull Request back to master with the CHANGELOG & version changes from the latest release. 272 - Remove all `R:minor` labels from the pull requests that were included in the release. 273 > Note: Do not merge the release branch into master. 274 275 #### Backport Release 276 277 1. start from the existing release branch you want to backport changes to (e.g. v0.30) 278 Branch to a release/vX.X.X branch locally (e.g. release/v0.30.7) 279 2. cherry pick the commit(s) that contain the changes you want to backport (usually these commits are from squash-merged PRs which were already reviewed) 280 3. steps 2 and 3 from [Major Release](#major-release) 281 4. push changes to release/vX.X.X branch 282 5. open a PR against the existing vX.X branch 283 284 ## Testing 285 286 All repos should be hooked up to [CircleCI](https://circleci.com/). 287 288 If they have `.go` files in the root directory, they will be automatically 289 tested by circle using `go test -v -race ./...`. If not, they will need a 290 `circle.yml`. Ideally, every repo has a `Makefile` that defines `make test` and 291 includes its continuous integration status using a badge in the `README.md`. 292 293 ### RPC Testing 294 295 If you contribute to the RPC endpoints it's important to document your changes in the [Swagger file](./rpc/swagger/swagger.yaml) 296 To test your changes you should install `nodejs` and run: 297 298 ```bash 299 npm i -g dredd 300 make build-linux build-contract-tests-hooks 301 make contract-tests 302 ``` 303 304 This command will popup a network and check every endpoint against what has been documented