github.com/wendylau87/warungpintar2021/inventorysvc@v0.0.0-20210508064910-5fb678f1d33e/README.md (about) 1 # go-clean-architecture-web-application-boilerplate 2 A web application boilerplate built with go and clean architecture. 3 Most of this application built by standard libray. 4 5 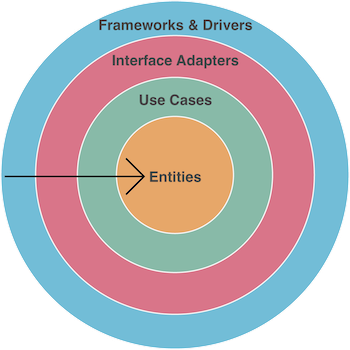 6 7 # Get Started 8 `cp app/.env_example app/.env` 9 `docker-compose build` 10 `docker-compose up` 11 12 After running docker, you need to execute sql files in `app/database/sql`. 13 14 # Architecture 15 ``` 16 app 17 ├── database 18 │ ├── migrations 19 │ │ └── schema.sql 20 │ └── seeds 21 │ └── faker.sql 22 ├── domain 23 │ ├── post.go 24 │ └── user.go 25 ├── infrastructure 26 │ ├── env.go 27 │ ├── logger.go 28 │ ├── router.go 29 │ └── sqlhandler.go 30 ├── interfaces 31 │ ├── post_controller.go 32 │ ├── post_repository.go 33 │ ├── sqlhandler.go 34 │ ├── user_controller.go 35 │ └── user_repository.go 36 ├── log 37 │ ├── access.log 38 │ └── error.log 39 ├── main.go 40 └── usecases 41 ├── logger.go 42 ├── post_interactor.go 43 ├── post_repository.go 44 ├── user_interactor.go 45 └── user_repository.go 46 47 8 directories, 22 files 48 ``` 49 50 | Layer | Directory | 51 |----------------------|----------------| 52 | Frameworks & Drivers | infrastructure | 53 | Interface | interfaces | 54 | Usecases | usecases | 55 | Entities | domain | 56 57 # API 58 59 | ENDPOINT | HTTP Method | Parameters | 60 |----------|----------------|---------------| 61 | /users | GET | | 62 | /user | GET | ?id=[int] | 63 | /posts | GET | | 64 | /post | POST | | 65 | /post | DELETE | ?id=[int] | 66 67 # Controller method naming rule 68 69 | Controller Method | HTTP Method | Description | 70 |-------------------|-------------|--------------------------------------------| 71 | Index | GET | Display a listing of the resource | 72 | Store | POST | Store a newly created resource in storage | 73 | Show | GET | Display the specified resource | 74 | Update | PUT/PATCH | Update the specified resource in storage | 75 | Destroy | DELETE | Remove the specified resource from storage | 76 77 # Repository method naming rule 78 79 | Repository Method | Description | 80 |-------------------|------------------------------------------------------| 81 | FindByXX | Returns the entity identified by the given XX | 82 | FindAll | Returns all entities | 83 | Save | Saves the given entity | 84 | SaveByXX | Saves the given entity identified by the given XX | 85 | DeleteByXX | Deletes the entity identified by the given XX | 86 | Count | Returns the number of entities | 87 | ExistsBy | Indicates whether an entity with the given ID exists | 88 89 cf. [Spring Data JPA - Reference Documentation](https://docs.spring.io/spring-data/data-jpa/docs/current/reference/html/#repositories.core-concepts) 90 91 # Tests 92 I have no tests because of my laziness, but I will prepare tests in [github - gobel-api](https://github.com/bmf-san/gobel-api) which is a my more practical clean architecture application with golang. 93 94 # References 95 - [github - manuelkiessling/go-cleanarchitecture](https://github.com/manuelkiessling/go-cleanarchitecture) 96 - [github - rymccue/golang-standard-lib-rest-api](https://github.com/rymccue/golang-standard-lib-rest-api) 97 - [github - hirotakan/go-cleanarchitecture-sample](https://github.com/hirotakan/go-cleanarchitecture-sample) 98 - [Recruit Technologies - Go言語とDependency Injection](https://recruit-tech.co.jp/blog/2017/12/11/go_dependency_injection/) 99 - [Clean ArchitectureでAPI Serverを構築してみる](https://qiita.com/hirotakan/items/698c1f5773a3cca6193e) 100 - [github - ponzu-cms/ponzu](https://github.com/ponzu-cms/ponzu) 101 - [クリーンアーキテクチャの書籍を読んだのでAPIサーバを実装してみた](https://qiita.com/yoshinori_hisakawa/items/f934178d4bd476c8da32) 102 - [Go × Clean Architectureのサンプル実装](http://nakawatch.hatenablog.com/entry/2018/07/11/181453) 103 - [Uncle Bob – Payroll Case Study (A full implementation)](http://cleancodejava.com/uncle-bob-payroll-case-study-full-implementation/)