github.com/xgzlucario/GigaCache@v0.0.0-20240508025442-54204e9c8a6b/README.md (about) 1 # GigaCache 2 3 [](https://goreportcard.com/report/github.com/xgzlucario/GigaCache) [](https://pkg.go.dev/github.com/xgzlucario/GigaCache)   [](https://codecov.io/gh/xgzlucario/GigaCache) [](https://github.com/xgzlucario/GigaCache/actions/workflows/rotom.yml) 4 5 GigaCache 是一个 Golang 编写的高性能缓存库,使用紧凑的字节数组作为数据容器,为 GB 级序列化数据而设计,支持设置过期时间与淘汰机制,相比 `stdmap` 有更快的速度,更高的内存效率,和更小的 GC 延迟。 6 7 特性: 8 9 1. 高效管理序列化的数据,存储相同数据量相比 `map[string]string` 内存占用减少 **60%**,性能提升 **40%+**,GC 延迟降低 **30%**。 10 2. 采用分片技术减小锁粒度,并分块管理数据 11 3. 键值对独立的过期时间支持,使用定期淘汰策略驱逐过期的键值对 12 4. 内置迁移算法,定期整理碎片空间,以释放内存 13 5. 类似于 `bigcache` 规避 GC 的设计,上亿数据集的 P99 延迟在微秒级别 14 15 你可以阅读 [博客文档](https://lucario.cn/posts/gigacache/) 了解更多的技术细节。 16 17 # 性能 18 19 下面是插入 2000 万条数据的性能对比测试,`GigaCache` 的插入速度相比 `stdmap` 提升 **40%+**,内存占用减少了 **60%** 左右,GC 延迟降低了 **30%**。 20 21 ``` 22 gigacache 23 entries: 20000000 24 alloc: 1103 mb 25 gcsys: 7 mb 26 heap inuse: 1103 mb 27 heap object: 2598 k 28 gc: 13 29 pause: 2.092264ms 30 cost: 13.172292035s 31 ``` 32 33 ``` 34 stdmap 35 entries: 20000000 36 alloc: 2703 mb 37 gcsys: 16 mb 38 heap inuse: 2709 mb 39 heap object: 29597 k 40 gc: 11 41 pause: 2.702983ms 42 cost: 18.730218048s 43 ``` 44 45 **详细测试** 46 47 运行 `make bench` 命令进行详细测试: 48 49 ``` 50 goos: linux 51 goarch: amd64 52 pkg: github.com/xgzlucario/GigaCache 53 cpu: 13th Gen Intel(R) Core(TM) i5-13600KF 54 BenchmarkSet/stdmap-20 3978168 360.2 ns/op 225 B/op 3 allocs/op 55 BenchmarkSet/cache-20 5956052 234.2 ns/op 95 B/op 2 allocs/op 56 BenchmarkSet/cache/disableEvict-20 7046114 214.1 ns/op 104 B/op 2 allocs/op 57 BenchmarkGet/stdmap-20 8400390 152.7 ns/op 15 B/op 1 allocs/op 58 BenchmarkGet/cache-20 10187661 117.5 ns/op 16 B/op 2 allocs/op 59 BenchmarkScan/stdmap-20 151 7814006 ns/op 0 B/op 0 allocs/op 60 BenchmarkScan/cache-20 100 11475189 ns/op 0 B/op 0 allocs/op 61 BenchmarkRemove/stdmap-20 16358985 66.26 ns/op 16 B/op 1 allocs/op 62 BenchmarkRemove/cache-20 7386679 151.4 ns/op 16 B/op 2 allocs/op 63 BenchmarkMigrate/stdmap-20 676 1739740 ns/op 6441224 B/op 1685 allocs/op 64 BenchmarkMigrate/cache-20 343 3561328 ns/op 24620 B/op 1024 allocs/op 65 BenchmarkMigrate/cache/parallel-20 1183 1033018 ns/op 42423 B/op 2091 allocs/op 66 BenchmarkIdx/newIdx-20 1000000000 0.7566 ns/op 0 B/op 0 allocs/op 67 BenchmarkIdx/newIdxx-20 1000000000 0.3761 ns/op 0 B/op 0 allocs/op 68 PASS 69 ``` 70 71 **测试环境** 72 73 ``` 74 goos: linux 75 goarch: amd64 76 pkg: github.com/xgzlucario/GigaCache 77 cpu: AMD Ryzen 7 5800H with Radeon Graphics 78 ``` 79 80 # 使用 81 82 首先安装 GigaCache 到本地: 83 84 ```bash 85 go get github.com/xgzlucario/GigaCache 86 ``` 87 88 运行下面的代码示例: 89 90 ```go 91 package main 92 93 import ( 94 "fmt" 95 cache "github.com/xgzlucario/GigaCache" 96 ) 97 98 func main() { 99 m := cache.New(cache.DefaultOptions) 100 101 m.Set("foo", []byte("bar")) 102 // Set with expired time. 103 m.SetEx("foo1", []byte("bar1"), time.Minute) 104 // Set with deadline. 105 m.SetTx("foo2", []byte("bar2"), time.Now().Add(time.Minute).UnixNano()) 106 107 val, ts, ok := m.Get("foo") 108 fmt.Println(string(val), ok) // bar, (nanosecs), true 109 110 ok := m.Delete("foo1") // true 111 if ok { 112 // ... 113 } 114 115 // or Range cache 116 m.Scan(func(key, val []byte, ts int64) bool { 117 // ... 118 return false 119 }) 120 } 121 ``` 122 123 # 内部架构 124 125 GigaCache 126 127 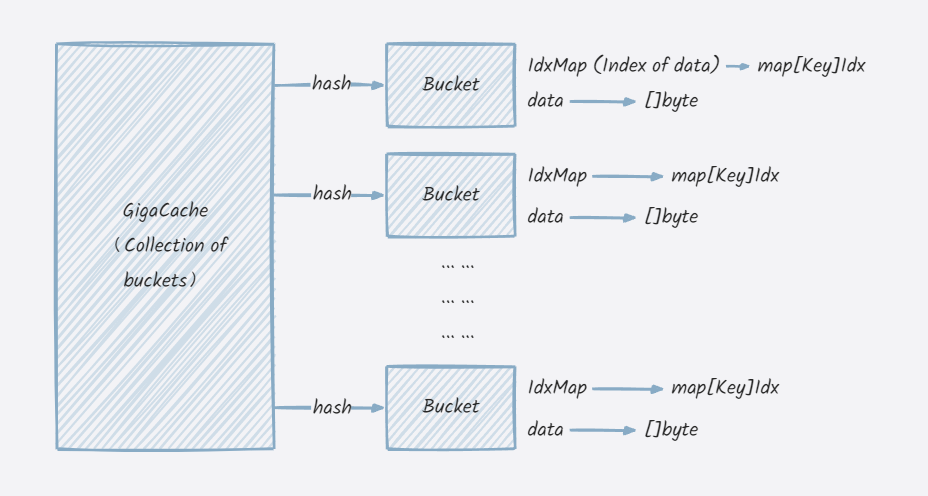 128 129 Key & Idx 130 131 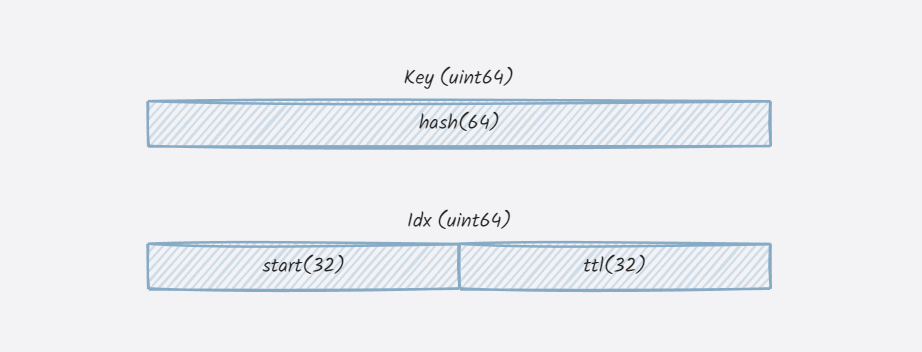 132 133 Bucket 134 135 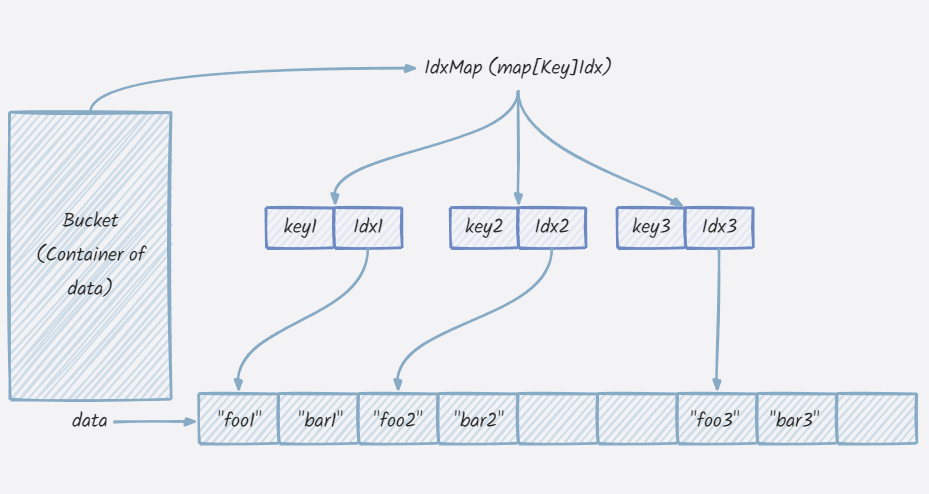