gitlab.com/evatix-go/core@v1.3.55/README.md (about) 1 # `Core` Intro 2 3 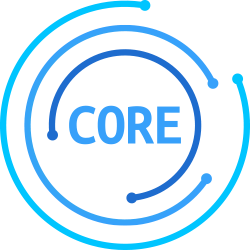 4 5 All common core infrastructure and constants combined package. 6 7 ## Git Clone 8 9 `git clone https://gitlab.com/evatix-go/core.git` 10 11 ### 2FA enabled, for linux 12 13 `git clone https://[YourGitLabUserName]:[YourGitlabAcessTokenGenerateFromGitlabsTokens]@gitlab.com/evatix-go/core.git` 14 15 ### Prerequisites 16 17 - Update git to latest 2.29 18 - Update or install the latest of Go 1.15.2 19 - Either add your ssh key to your gitlab account 20 - Or, use your access token to clone it. 21 22 ## Installation 23 24 `go get gitlab.com/evatix-go/core` 25 26 ### Go get issue for private package 27 28 - Update git to 2.29 29 - Enable go modules. (Windows : `go env -w GO111MODULE=on`, Unix : `export GO111MODULE=on`) 30 - Add `gitlab.com/evatix-go` to go env private 31 32 To set for Windows: 33 34 `go env -w GOPRIVATE=[AddExistingOnes;]gitlab.com/evatix-go` 35 36 To set for Unix: 37 38 `expoort GOPRIVATE=[AddExistingOnes;]gitlab.com/evatix-go` 39 40 ## Why `core?` 41 42 It makes our other go-packages DRY and concise. 43 44 ## Examples Videos 45 46 - [Core Basics Intro](https://drive.google.com/file/d/1CA4817zaehhWqgtAGI2UH7Tojtngcyjw/view) 47 - [Core Usage Video](https://drive.google.com/file/d/1kwC_3R-QIZE1pNK_9F7hFdYuGB0CSGYh/view?usp=sharing) 48 49 ## Examples 50 51 ```go= 52 // substituting functions as ternary operator 53 fmt.Println(conditional.Int(true, 2, 7)) // 2 54 fmt.Println(conditional.Int(false, 2, 7)) // 7 55 56 // making collection from array of strings 57 stringValues := []string{"hello", "world", "something"} 58 collectionPtr1 := corestr.NewCollectionPtrUsingStrings(&stringValues, constants.Zero) 59 fmt.Println(collectionPtr1) 60 /* outputs: 61 - hello 62 - world 63 - something 64 */ 65 66 // different methods of collection 67 fmt.Println(collectionPtr1.Length()) // 3 68 fmt.Println(collectionPtr1.IsEmpty()) // false 69 70 // adding more element including empty string 71 collectionPtr2 := collectionPtr1.AddsLock("else") 72 fmt.Println(collectionPtr2.Length()) // 4 73 74 // checking equality 75 fmt.Println(collectionPtr1.IsEqualsPtr(collectionPtr2)) // true 76 77 // creating CharCollectionMap using collection 78 sampleMap := collectionPtr1.CharCollectionPtrMap() 79 fmt.Println(sampleMap) 80 81 // methods on CharCollectionMap 82 fmt.Println(sampleMap.Length()) // 4 83 fmt.Println(sampleMap.AllLengthsSum()) // 4 84 fmt.Println(sampleMap.Clear()) // prints: # Summary of `*corestr.CharCollectionMap`, Length ("0") - Sequence `1` 85 otherMap := sampleMap.Add("another") 86 fmt.Println(otherMap) 87 /* prints: 88 # Summary of `*corestr.CharCollectionMap`, Length ("1") - Sequence `1` 89 1 . `a` has `1` items. 90 ## Items of `a` 91 - another 92 */ 93 94 // declaring an empty hashset of length 2 and calling methods on it 95 newHashSet := corestr.NewHashset(2) 96 fmt.Println(newHashSet.Length()) // 2 97 fmt.Println(newHashSet.IsEmpty()) // true 98 fmt.Println(newHashSet.Items()) // &map[] 99 100 // adding items to hashset 101 strPtr := "new" 102 newHashSet.AddPtr(&strPtr) 103 fmt.Println(newHashSet.Items()) // &map[new:true] 104 105 // adding map to hashset 106 newHashSet.AddItemsMap(&map[string]bool{"hi": true, "no": false}) 107 fmt.Println(newHashSet.Items()) // &map[hi:true new:true] 108 109 // math operations: getting the larger/smaller value from two given values 110 fmt.Println(coremath.MaxByte('e', 'i')) // 105 which represents 'i' in ASCII 111 fmt.Println(coremath.MinByte(23, 5)) // 5 112 113 // initializing issetter value 114 isSetterValue := issetter.False // initializing as false 115 fmt.Println(isSetterValue.HasInitialized()) // true 116 fmt.Println(isSetterValue.Value()) // 2 117 fmt.Println(isSetterValue.IsPositive()) // false 118 119 // sorting strings 120 fruits := []string{"banana", "mango", "apple"} 121 fmt.Println(strsort.Quick(&fruits)) // &[apple banana mango] 122 fmt.Println(strsort.QuickDsc(&fruits)) // &[mango banana apple] 123 124 // converting pointer strings to strings 125 mile := "mile" 126 km := "km" 127 measures := []*string{&mile, &km} 128 fmt.Println(converters.PointerStringsToStrings(&measures)) // &[mile km] 129 fmt.Printf("Type %T", converters.PointerStringsToStrings(&measures)) // Type *[]string 130 131 // comparing two int arays 132 Values := []int{1, 2, 3, 4} 133 OtherValues := []int{5, 6, 7, 8} 134 fmt.Println(corecompare.IntArray(Values, OtherValues)) // false 135 ``` 136 137 ## Acknowledgement 138 139 Any other packages used 140 141 ## Links 142 143 - [go - Calling a method on a nil struct pointer doesn't panic.](https://t.ly/aTp0) 144 - [Array of pointers to JSON - Stack Overflow](https://stackoverflow.com/questions/28101966/array-of-pointers-to-json) 145 - [Json Parsing of Array Pointers](https://play.golang.org/p/zTuMLBgGWk) 146 - [Go Slice Tricks Cheat Sheet 147 ](https://ueokande.github.io/go-slice-tricks/) 148 - [SliceTricks ยท golang/go Wiki 149 ](https://github.com/golang/go/wiki/SliceTricks) 150 - [ueokande/go-slice-tricks: Cheat Sheet for Go Slice Tricks](https://github.com/ueokande/go-slice-tricks) 151 - [Quick Sort in Go (Golang) - golangprograms.com](https://t.ly/pDyj) 152 - [Sorting using golang lib](https://play.golang.org/p/sJ8a464USeV) 153 - [Pointer Strings Sort](https://play.golang.org/p/8V8YYdQrO6q) 154 - [Golang Array process issue without copying (!Important)](https://play.golang.org/p/GvdJMPmCStz) 155 - [Linked List | Set 2 (Inserting a node) - GeeksforGeeks](https://t.ly/MMaY) 156 - [Go Data Structures: Linked List](https://t.ly/QLLy) 157 - [System info](https://github.com/zcalusic/sysinfo) 158 -[Stackoverflow Centos detect](https://stackoverflow.com/a/65207574) 159 160 ### Regex Patterns 161 162 #### Path RegEx Patterns 163 164 * [java - Regex pattern to validate Linux folder path - Stack Overflow](https://stackoverflow.com/questions/55069650/regex-pattern-to-validate-linux-folder-path/55070259) 165 * [regex - What is the most correct regular expression for a UNIX file path? - Stack Overflow](https://stackoverflow.com/questions/537772/what-is-the-most-correct-regular-expression-for-a-unix-file-path) 166 * [java - Regular expression to validate windows and linux path with extension - Stack Overflow](https://stackoverflow.com/questions/44289075/regular-expression-to-validate-windows-and-linux-path-with-extension) 167 * [javascript - Regex windows path validator - Stack Overflow](https://stackoverflow.com/questions/51494579/regex-windows-path-validator/51504254) 168 * [Path Regex fix for all OS](https://t.ly/1JuS) 169 170 ## Issues 171 172 - [Create your issues](https://gitlab.com/evatix-go/core/-/issues) 173 174 ## Notes 175 176 ## Contributors 177 178 ## License 179 180 [Evatix MIT License](/LICENSE)