sigs.k8s.io/cluster-api@v1.7.1/docs/book/src/developer/providers/bootstrap.md (about) 1 # Bootstrap Provider Specification 2 3 ## Overview 4 5 A bootstrap provider generates bootstrap data that is used to bootstrap a Kubernetes node. 6 7 For example, the Kubeadm bootstrap provider uses a [cloud-init](https://cloudinit.readthedocs.io/en/latest/) file for bootstrapping a node. 8 9 ## Data Types 10 11 ### Bootstrap API resource 12 A bootstrap provider must define an API type for bootstrap resources. The type: 13 14 1. Must belong to an API group served by the Kubernetes apiserver 15 2. Must be implemented as a CustomResourceDefinition. 16 1. The CRD name must have the format produced by `sigs.k8s.io/cluster-api/util/contract.CalculateCRDName(Group, Kind)`. 17 3. Must be namespace-scoped 18 4. Must have the standard Kubernetes "type metadata" and "object metadata" 19 5. Should have a `spec` field containing fields relevant to the bootstrap provider 20 6. Must have a `status` field with the following: 21 1. Required fields: 22 1. `ready` (boolean): indicates the bootstrap data has been generated and is ready 23 1. `dataSecretName` (string): the name of the secret that stores the generated bootstrap data 24 2. Optional fields: 25 1. `failureReason` (string): indicates there is a fatal problem reconciling the bootstrap data; 26 meant to be suitable for programmatic interpretation 27 2. `failureMessage` (string): indicates there is a fatal problem reconciling the bootstrap data; 28 meant to be a more descriptive value than `failureReason` 29 30 Note: because the `dataSecretName` is part of `status`, this value must be deterministically recreatable from the data in the 31 `Cluster`, `Machine`, and/or bootstrap resource. If the name is randomly generated, it is not always possible to move 32 the resource and its associated secret from one management cluster to another. 33 34 ### BootstrapTemplate Resources 35 36 For a given Bootstrap resource, you should also add a corresponding BootstrapTemplate resource: 37 38 ``` go 39 // PhippyBootstrapConfigTemplateSpec defines the desired state of PhippyBootstrapConfigTemplate. 40 type PhippyBootstrapConfigTemplateSpec struct { 41 Template PhippyBootstrapTemplateResource `json:"template"` 42 } 43 44 // +kubebuilder:object:root=true 45 // +kubebuilder:resource:path=phippybootstrapconfigtemplates,scope=Namespaced,categories=cluster-api,shortName=pbct 46 // +kubebuilder:storageversion 47 48 // PhippyBootstrapConfigTemplate is the Schema for the Phippy Bootstrap API. 49 type PhippyBootstrapConfigTemplate struct { 50 metav1.TypeMeta `json:",inline"` 51 metav1.ObjectMeta `json:"metadata,omitempty"` 52 53 Spec PhippyBootstrapConfigTemplateSpec `json:"spec,omitempty"` 54 } 55 56 type PhippyBootstrapConfigTemplateResource struct { 57 // Standard object's metadata. 58 // More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata 59 // +optional 60 ObjectMeta clusterv1.ObjectMeta `json:"metadata,omitempty"` 61 62 Spec PhippyBootstrapConfigSpec `json:"spec"` 63 } 64 ``` 65 66 The CRD name of the template must also have the format produced by `sigs.k8s.io/cluster-api/util/contract.CalculateCRDName(Group, Kind)`. 67 68 ### List Resources 69 70 For any resource, also add list resources, e.g. 71 72 ```go 73 //+kubebuilder:object:root=true 74 75 // PhippyBootstrapConfigList contains a list of Phippy Bootstrap Configurations. 76 type PhippyBootstrapConfigList struct { 77 metav1.TypeMeta `json:",inline"` 78 metav1.ListMeta `json:"metadata,omitempty"` 79 Items []PhippyBootstrapConfig `json:"items"` 80 } 81 82 //+kubebuilder:object:root=true 83 84 // PhippyBootstrapConfigTemplateList contains a list of PhippyBootstrapConfigTemplate. 85 type PhippyBootstrapConfigTemplateList struct { 86 metav1.TypeMeta `json:",inline"` 87 metav1.ListMeta `json:"metadata,omitempty"` 88 Items []PhippyBootstrapConfigTemplate `json:"items"` 89 } 90 ``` 91 92 93 ### Bootstrap Secret 94 95 The `Secret` containing bootstrap data must: 96 97 1. Use the API resource's `status.dataSecretName` for its name 98 1. Have the label `cluster.x-k8s.io/cluster-name` set to the name of the cluster 99 1. Have a controller owner reference to the API resource 100 1. Have a single key, `value`, containing the bootstrap data 101 102 ## Behavior 103 104 A bootstrap provider must respond to changes to its bootstrap resources. This process is 105 typically called reconciliation. The provider must watch for new, updated, and deleted resources and respond 106 accordingly. 107 108 The following diagram shows the typical logic for a bootstrap provider: 109 110 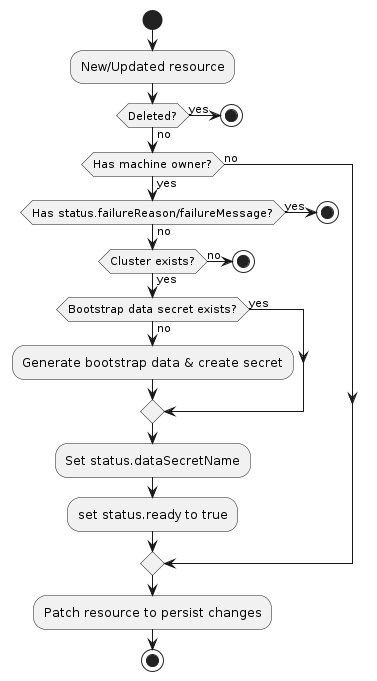 111 112 1. If the resource does not have a `Machine` owner, exit the reconciliation 113 1. The Cluster API `Machine` reconciler populates this based on the value in the `Machine`'s `spec.bootstrap.configRef` 114 field. 115 1. If the resource has `status.failureReason` or `status.failureMessage` set, exit the reconciliation 116 1. If the `Cluster` to which this resource belongs cannot be found, exit the reconciliation 117 1. Deterministically generate the name for the bootstrap data secret 118 1. Try to retrieve the `Secret` with the name from the previous step 119 1. If it does not exist, generate bootstrap data and create the `Secret` 120 1. Set `status.dataSecretName` to the generated name 121 1. Set `status.ready` to true 122 1. Patch the resource to persist changes 123 124 ## Sentinel File 125 126 A bootstrap provider's bootstrap data must create `/run/cluster-api/bootstrap-success.complete` (or `C:\run\cluster-api\bootstrap-success.complete` for Windows machines) upon successful bootstrapping of a Kubernetes node. This allows infrastructure providers to detect and act on bootstrap failures. 127 128 ## Taint Nodes at creation 129 130 A bootstrap provider can optionally taint worker nodes at creation with `node.cluster.x-k8s.io/uninitialized:NoSchedule`. 131 This taint is used to prevent workloads to be scheduled on Nodes before the node is initialized by Cluster API. 132 As of today the Node initialization consists of syncing labels from Machines to Nodes. Once the labels have been 133 initially synced the taint is removed form the Node. 134 135 ## RBAC 136 137 ### Provider controller 138 139 A bootstrap provider must have RBAC permissions for the types it defines, as well as the bootstrap data `Secret` 140 resources it manages. If you are using `kubebuilder` to generate new API types, these permissions should be configured 141 for you automatically. For example, the Kubeadm bootstrap provider the following configuration for its `KubeadmConfig` 142 type: 143 144 ``` 145 // +kubebuilder:rbac:groups=bootstrap.cluster.x-k8s.io,resources=kubeadmconfigs;kubeadmconfigs/status,verbs=get;list;watch;create;update;patch;delete 146 // +kubebuilder:rbac:groups="",resources=secrets,verbs=get;list;watch;create;update;patch;delete 147 ``` 148 149 A bootstrap provider may also need RBAC permissions for other types, such as `Cluster`. If you need 150 read-only access, you can limit the permissions to `get`, `list`, and `watch`. The following 151 configuration can be used for retrieving `Cluster` resources: 152 153 ``` 154 // +kubebuilder:rbac:groups=cluster.x-k8s.io,resources=clusters;clusters/status,verbs=get;list;watch 155 ``` 156 157 ### Cluster API controllers 158 159 The Cluster API controller for `Machine` resources is configured with full read/write RBAC permissions for all resources 160 in the `bootstrap.cluster.x-k8s.io` API group. This group represents all bootstrap providers for SIG Cluster 161 Lifecycle-sponsored provider subprojects. If you are writing a provider not sponsored by the SIG, you must add new RBAC 162 permissions for the Cluster API `manager-role` role, granting it full read/write access to the bootstrap resource in 163 your API group. 164 165 Note, the write permissions allow the `Machine` controller to set owner references and labels on the bootstrap 166 resources; they are not used for general mutations of these resources.