sigs.k8s.io/cluster-api@v1.7.1/docs/book/src/tasks/experimental-features/runtime-sdk/implement-lifecycle-hooks.md (about) 1 # Implementing Lifecycle Hook Runtime Extensions 2 3 <aside class="note warning"> 4 5 <h1>Caution</h1> 6 7 Please note Runtime SDK is an advanced feature. If implemented incorrectly, a failing Runtime Extension can severely impact the Cluster API runtime. 8 9 </aside> 10 11 ## Introduction 12 13 The lifecycle hooks allow hooking into the Cluster lifecycle. The following diagram provides an overview: 14 15 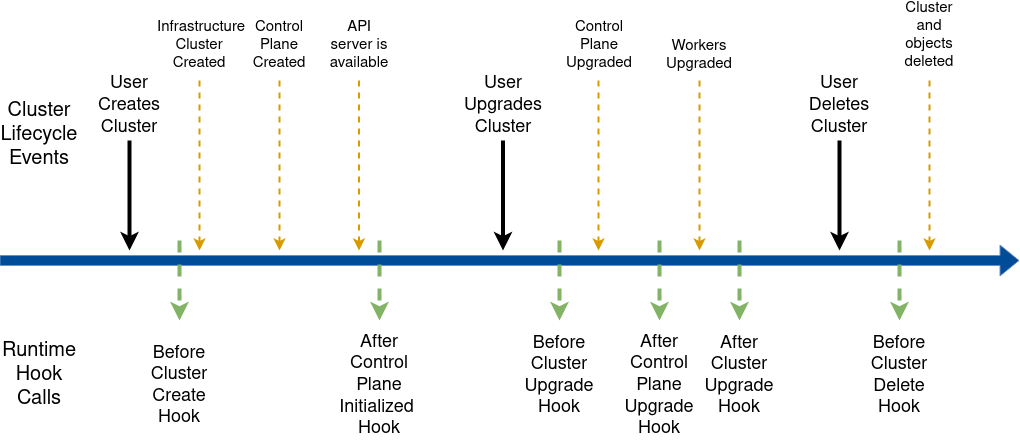 16 17 Please see the corresponding [CAEP](https://github.com/kubernetes-sigs/cluster-api/blob/main/docs/proposals/20220414-runtime-hooks.md) 18 for additional background information. 19 20 ## Guidelines 21 22 All guidelines defined in [Implementing Runtime Extensions](implement-extensions.md#guidelines) apply to the 23 implementation of Runtime Extensions for lifecycle hooks as well. 24 25 In summary, Runtime Extensions are components that should be designed, written and deployed with great caution given 26 that they can affect the proper functioning of the Cluster API runtime. A poorly implemented Runtime Extension could 27 potentially block lifecycle transitions from happening. 28 29 Following recommendations are especially relevant: 30 31 * [Blocking and non Blocking](implement-extensions.md#blocking-hooks) 32 * [Error messages](implement-extensions.md#error-messages) 33 * [Error management](implement-extensions.md#error-management) 34 * [Avoid dependencies](implement-extensions.md#avoid-dependencies) 35 36 ## Definitions 37 38 ### BeforeClusterCreate 39 40 This hook is called after the Cluster object has been created by the user, immediately before all the objects which 41 are part of a Cluster topology(*) are going to be created. Runtime Extension implementers can use this hook to 42 determine/prepare add-ons for the Cluster and block the creation of those objects until everything is ready. 43 44 #### Example Request: 45 46 ```yaml 47 apiVersion: hooks.runtime.cluster.x-k8s.io/v1alpha1 48 kind: BeforeClusterCreateRequest 49 settings: <Runtime Extension settings> 50 cluster: 51 apiVersion: cluster.x-k8s.io/v1beta1 52 kind: Cluster 53 metadata: 54 name: test-cluster 55 namespace: test-ns 56 spec: 57 ... 58 status: 59 ... 60 ``` 61 62 #### Example Response: 63 64 ```yaml 65 apiVersion: hooks.runtime.cluster.x-k8s.io/v1alpha1 66 kind: BeforeClusterCreateResponse 67 status: Success # or Failure 68 message: "error message if status == Failure" 69 retryAfterSeconds: 10 70 ``` 71 72 For additional details, you can see the full schema in <button onclick="openSwaggerUI()">Swagger UI</button>. 73 74 (*) The objects which are part of a Cluster topology are the infrastructure Cluster, the Control Plane, the 75 MachineDeployments and the templates derived from the ClusterClass. 76 77 ### AfterControlPlaneInitialized 78 79 This hook is called after the Control Plane for the Cluster is marked as available for the first time. Runtime Extension 80 implementers can use this hook to execute tasks, for example component installation on workload clusters, that are only 81 possible once the Control Plane is available. This hook does not block any further changes to the Cluster. 82 83 #### Example Request: 84 85 ```yaml 86 apiVersion: hooks.runtime.cluster.x-k8s.io/v1alpha1 87 kind: AfterControlPlaneInitializedRequest 88 settings: <Runtime Extension settings> 89 cluster: 90 apiVersion: cluster.x-k8s.io/v1beta1 91 kind: Cluster 92 metadata: 93 name: test-cluster 94 namespace: test-ns 95 spec: 96 ... 97 status: 98 ... 99 ``` 100 101 #### Example Response: 102 103 ```yaml 104 apiVersion: hooks.runtime.cluster.x-k8s.io/v1alpha1 105 kind: AfterControlPlaneInitializedResponse 106 status: Success # or Failure 107 message: "error message if status == Failure" 108 ``` 109 110 For additional details, you can see the full schema in <button onclick="openSwaggerUI()">Swagger UI</button>. 111 112 ### BeforeClusterUpgrade 113 114 This hook is called after the Cluster object has been updated with a new `spec.topology.version` by the user, and 115 immediately before the new version is going to be propagated to the control plane (*). Runtime Extension implementers 116 can use this hook to execute pre-upgrade add-on tasks and block upgrades of the ControlPlane and Workers. 117 118 Note: While the upgrade is blocked changes made to the Cluster Topology will be delayed propagating to the underlying 119 objects while the object is waiting for upgrade. Example: modifying ControlPlane/MachineDeployments (think scale up), 120 or creating new MachineDeployments will be delayed until the target ControlPlane/MachineDeployment is ready to pick up the upgrade. 121 This ensures that the ControlPlane and MachineDeployments do not perform a rollout prematurely while waiting to be rolled out again for the version upgrade (no double rollouts). 122 This also ensures that any version specific changes are only pushed to the underlying objects also at the correct version. 123 124 #### Example Request: 125 126 ```yaml 127 apiVersion: hooks.runtime.cluster.x-k8s.io/v1alpha1 128 kind: BeforeClusterUpgradeRequest 129 settings: <Runtime Extension settings> 130 cluster: 131 apiVersion: cluster.x-k8s.io/v1beta1 132 kind: Cluster 133 metadata: 134 name: test-cluster 135 namespace: test-ns 136 spec: 137 ... 138 status: 139 ... 140 fromKubernetesVersion: "v1.21.2" 141 toKubernetesVersion: "v1.22.0" 142 ``` 143 144 #### Example Response: 145 146 ```yaml 147 apiVersion: hooks.runtime.cluster.x-k8s.io/v1alpha1 148 kind: BeforeClusterUpgradeResponse 149 status: Success # or Failure 150 message: "error message if status == Failure" 151 retryAfterSeconds: 10 152 ``` 153 154 For additional details, you can see the full schema in <button onclick="openSwaggerUI()">Swagger UI</button>. 155 156 (*) Under normal circumstances `spec.topology.version` gets propagated to the control plane immediately; however 157 if previous upgrades or worker machine rollouts are still in progress, the system waits for those operations 158 to complete before starting the new upgrade. 159 160 ### AfterControlPlaneUpgrade 161 162 This hook is called after the control plane has been upgraded to the version specified in `spec.topology.version`, 163 and immediately before the new version is going to be propagated to the MachineDeployments of the Cluster. 164 Runtime Extension implementers can use this hook to execute post-upgrade add-on tasks and block upgrades to workers 165 until everything is ready. 166 167 Note: While the MachineDeployments upgrade is blocked changes made to existing MachineDeployments and creating new MachineDeployments 168 will be delayed while the object is waiting for upgrade. Example: modifying MachineDeployments (think scale up), 169 or creating new MachineDeployments will be delayed until the target MachineDeployment is ready to pick up the upgrade. 170 This ensures that the MachineDeployments do not perform a rollout prematurely while waiting to be rolled out again for the version upgrade (no double rollouts). 171 This also ensures that any version specific changes are only pushed to the underlying objects also at the correct version. 172 173 #### Example Request: 174 175 ```yaml 176 apiVersion: hooks.runtime.cluster.x-k8s.io/v1alpha1 177 kind: AfterControlPlaneUpgradeRequest 178 settings: <Runtime Extension settings> 179 cluster: 180 apiVersion: cluster.x-k8s.io/v1beta1 181 kind: Cluster 182 metadata: 183 name: test-cluster 184 namespace: test-ns 185 spec: 186 ... 187 status: 188 ... 189 kubernetesVersion: "v1.22.0" 190 ``` 191 192 #### Example Response: 193 194 ```yaml 195 apiVersion: hooks.runtime.cluster.x-k8s.io/v1alpha1 196 kind: AfterControlPlaneUpgradeResponse 197 status: Success # or Failure 198 message: "error message if status == Failure" 199 retryAfterSeconds: 10 200 ``` 201 202 For additional details, you can see the full schema in <button onclick="openSwaggerUI()">Swagger UI</button>. 203 204 ### AfterClusterUpgrade 205 206 This hook is called after the Cluster, control plane and workers have been upgraded to the version specified in 207 `spec.topology.version`. Runtime Extensions implementers can use this hook to execute post-upgrade add-on tasks. 208 This hook does not block any further changes or upgrades to the Cluster. 209 210 #### Example Request: 211 212 ```yaml 213 apiVersion: hooks.runtime.cluster.x-k8s.io/v1alpha1 214 kind: AfterClusterUpgradeRequest 215 settings: <Runtime Extension settings> 216 cluster: 217 apiVersion: cluster.x-k8s.io/v1beta1 218 kind: Cluster 219 metadata: 220 name: test-cluster 221 namespace: test-ns 222 spec: 223 ... 224 status: 225 ... 226 kubernetesVersion: "v1.22.0" 227 ``` 228 229 #### Example Response: 230 231 ```yaml 232 apiVersion: hooks.runtime.cluster.x-k8s.io/v1alpha1 233 kind: AfterClusterUpgradeResponse 234 status: Success # or Failure 235 message: "error message if status == Failure" 236 ``` 237 238 For additional details, refer to the [Draft OpenAPI spec](https://editor.swagger.io/?url=https://raw.githubusercontent.com/kubernetes-sigs/cluster-api/main/docs/proposals/images/runtime-hooks/runtime-hooks-openapi.yaml). 239 240 ### BeforeClusterDelete 241 242 This hook is called after the Cluster deletion has been triggered by the user and immediately before the topology 243 of the Cluster is going to be deleted. Runtime Extension implementers can use this hook to execute 244 cleanup tasks for the add-ons and block deletion of the Cluster and descendant objects until everything is ready. 245 246 #### Example Request: 247 248 ```yaml 249 apiVersion: hooks.runtime.cluster.x-k8s.io/v1alpha1 250 kind: BeforeClusterDeleteRequest 251 settings: <Runtime Extension settings> 252 cluster: 253 apiVersion: cluster.x-k8s.io/v1beta1 254 kind: Cluster 255 metadata: 256 name: test-cluster 257 namespace: test-ns 258 spec: 259 ... 260 status: 261 ... 262 ``` 263 264 #### Example Response: 265 266 ```yaml 267 apiVersion: hooks.runtime.cluster.x-k8s.io/v1alpha1 268 kind: BeforeClusterDeleteResponse 269 status: Success # or Failure 270 message: "error message if status == Failure" 271 retryAfterSeconds: 10 272 ``` 273 274 For additional details, you can see the full schema in <button onclick="openSwaggerUI()">Swagger UI</button>. 275 276 <script> 277 // openSwaggerUI calculates the absolute URL of the RuntimeSDK YAML file and opens Swagger UI. 278 function openSwaggerUI() { 279 var schemaURL = new URL("runtime-sdk-openapi.yaml", document.baseURI).href 280 window.open("https://editor.swagger.io/?url=" + schemaURL) 281 } 282 </script>